Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial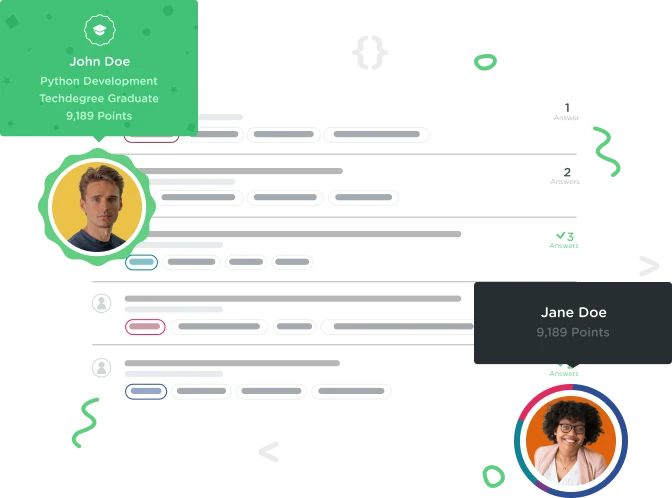

Travers Geoffray
Python Development Techdegree Graduate 26,967 PointsCompletely lost on this challenge question, would someone be able to help steer me in the right direction?
Have trouble getting started here. Also. is the button in this case supposed to add numbers and bold the whole text or just the numbers?
const laws = document.getElementsByTagName('li');
const indexText = document.getElementById('boldIndex');
const button = document.getElementById('embolden');
button.addEventListener('click', (e) => {
const index = parseInt(indexText.value, 10);
for (let i = 0; i < laws.length; i += 1) {
let law = laws[i];
// replace 'false' with a correct test condition on the line below
if (false) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>Newton's Laws</title>
</head>
<body>
<h1>Newton's Laws of Motion</h1>
<ul>
<li>An object in motion tends to stay in motion, unless acted on by an outside force.</li>
<li>Acceleration is dependent on the forces acting upon an object and the mass of the object.</li>
<li>For every action, there is an equal and opposite reaction.</li>
</ul>
<input type="text" id="boldIndex">
<button id="embolden">Embolden</button>
<script src="app.js"></script>
</body>
</html>
1 Answer

Ryan Groom
18,674 PointsTravers Geoffray Your code should look like this:
// replace 'false' with a correct test condition on the line below
if (indexText.value == i) {
law.style.fontWeight = 'bold';
} else {
law.style.fontWeight = 'normal';
}
In this "if" statement, indexText.value is representative of the number that you enter into the input field. The function runs through a loop for every list item you have on the page and the "i" in the "if" statement is representative of the current iteration of the loop you are on. So on the first iteration i = 0 and where it says law = laws[i], it is setting the variable law equal to laws[0] which is the first instance of a list item (all your list items were stored in an array referenced by the variable "laws" if you look at the constant declared at the top of your JS file). So if your inputs value matches the current iteration of the loop that you're on, then it sets the font weight of that specific list item to bold. I hope this makes sense, if not let me know what you need cleared up!
Travers Geoffray
Python Development Techdegree Graduate 26,967 PointsTravers Geoffray
Python Development Techdegree Graduate 26,967 PointsCompletely makes sense, thank you Ryan!