Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial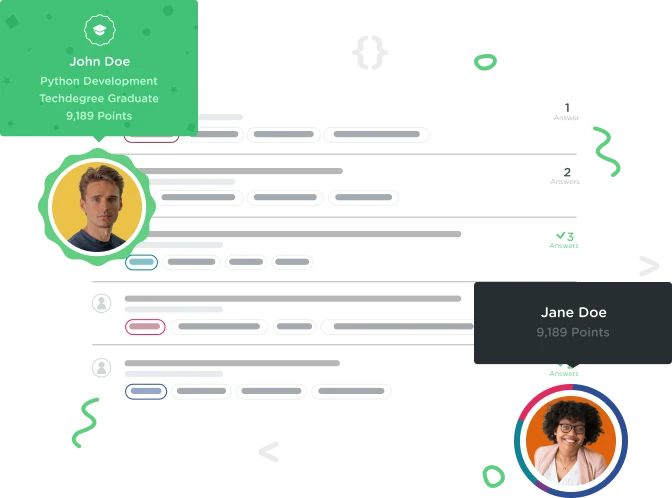
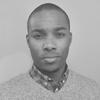
Henry Williams
iOS Development Techdegree Student 3,775 PointsCompleting "Putting the Fun back in Function" challenge with For loop
It seems i'm missing something here.
I am able to complete this challenge, at least to step two, without using a For loop but I don't really understand how to incorporate the for loop.
Help?
# add_list([1, 2, 3]) should return 6
# summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6."
# Note: both functions will only take *one* argument each.
shopping_list = []
def add_list(num):
for num in add_list
num = 1, 2, 3
return sum(num)
2 Answers
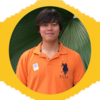
Erick Kusnadi
21,615 Pointsdef add_list(num):
for num in add_list
num = 1, 2, 3
return sum(num)
So let's break this down, the function add_list should take a list of numbers instead of just one number, so let's change the parameter name from num to num_list for number list.
def add_list(num_list):
for num in add_list
num = 1, 2, 3
return sum(num)
So the way you want to use the for loop is actually like this:
for num in num_list:
#do this
You would read the code like this: For every num or number in num_list or number list, colon, do this. The way the for loop works in python is you first put a variable name in this case num that will act as the item in the list you are iterating through. So first you use a variable name, num, then you use in and give it the list name in this case you want to use num_list which you passed into the function at the beginning.
So for every loop, the variable num will change into the next item in num_list.
For example, if num_list = 1,2,3 . On the first iteration num will equal 1, then on the second go, num will equal 2, and finally num will equal 3. When all the numbers in the list are used, the for loop will end.
Finally let's make one more variable that will be the sum total of adding all the numbers in the list. Let's call it total. The final code should look something like this:
def add_list(num_list):
total = 0
for num in num_list: #for every number in number_list
total += num #add number to the total
return total
Let me know if you need any more help.
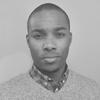
Henry Williams
iOS Development Techdegree Student 3,775 PointsSweet. I get it now.
Thanks a bunch!