Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial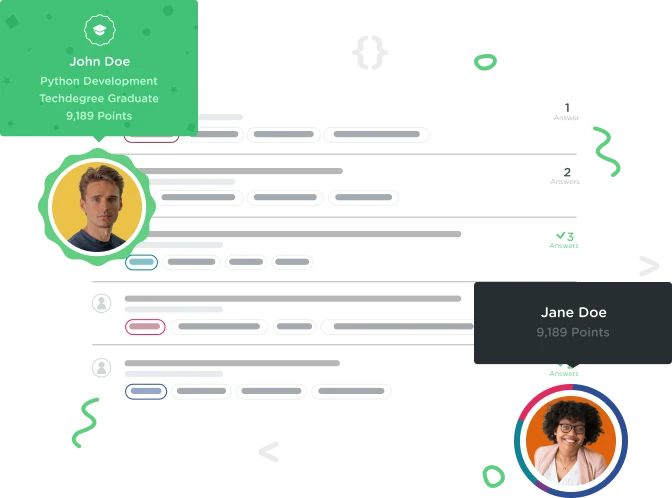

Chris Roberts
3,970 PointsCompleting the FizzBuzz challenge using a switch statement
I did the FizzBuzz challenge a while ago but I'm going back through the course and recapping everything. When I first did the challenge I did it using an if statement. The code worked fine but this time around I wanted to try it using a switch statement as I struggle with them and find myself using if/else statements whenever possible. I've spent the last 20 minutes or so fiddling around with it but I can't seem to find a way of doing it.
Thanks for any help :)
2 Answers

kasaltrix
9,491 PointsI completed the challenge too on my first attempt using the if/else statement. I also wanted to know how to complete the challenge using a switch statement so I searched online to find a solution. There is just a small trick that you have to keep in mind for this problem which is given below. It works because 'true' is a constant, so the code under the first case statement with an expression that evaluates to true will be executed. Although I recommend using if/else for these kind of problems. Hope this helps :)
func fizzBuzz(n: Int) -> String {
switch (true) {
case (n%3 == 0 && n%5 == 0):
return "Fizzbuzz"
case n%3 == 0:
return "Fizz"
case n%5 == 0:
return "Buzz"
default:
return "\(n)"
}
}

Izzy Ali
2,419 PointsAn alternative way of doing using switch would be this:
for i in 1...100 {
/* 1 */
switch (i % 3, i % 5) {
/* 2 */
case (0, 0):
print("FizzBuzz", terminator: " ")
case (0, _):
print("Fizz", terminator: " ")
case (_, 0):
print("Buzz", terminator: " ")
/* 3 */
case (_, _):
print(i, terminator: " ")
}
} print("")
Hereโs whatโs going on:
- You construct a tuple in the switch expression.
- Each of the cases checks a result of the modulo operation. The underscore means you donโt care and it matches any value.
- A tuple pattern with all underscores, (_, _), matches any value thus works as a default case
Though I would recommend using a if statement though for this problem
Chris Roberts
3,970 PointsChris Roberts
3,970 Pointsoooooohhhhhhhh I see. Took me some time to figure out the whole "switch true" thing but I get it now, the value you're getting from the cases is true or false and can't be compared to something like an Int. Appreciate the answer!
kasaltrix
9,491 Pointskasaltrix
9,491 PointsGlad that you figured it out :)