Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial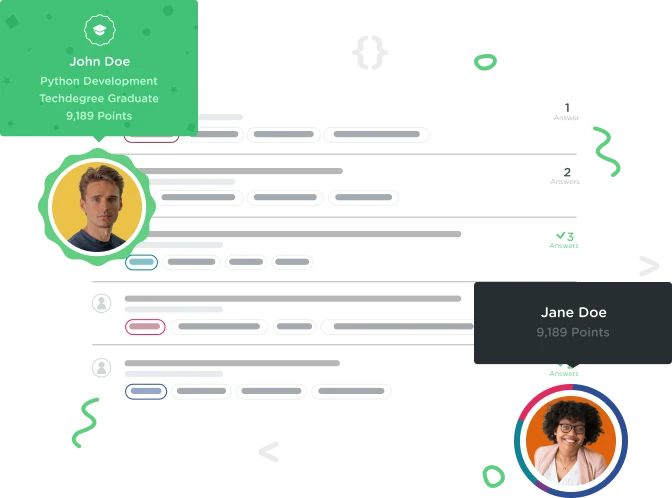

Truong Minh Nguyen
12,587 PointsComponent returned value cannot starts with an iteration
Hi guys. I have a react component as follows:
const PlanetList = (props) => {
return (
// <div className="container">
{props.planets.map(planet =>
<Planet
key={planet.id}
name={planet.name}
diameter={planet.diameter}
moons={planet.moons}
desc={planet.desc}
url={planet.url}
/>
)}
// </div>
);
}
The commented lines will cause the error 'Unexpected token, expected ,' but if I uncomment them, it works normally.
So the returned statement must always start with an element like <div className="container">
?
2 Answers
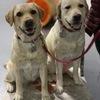
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Truong,
Like Bader says in his comment, a valid JSX object cannot be just a JSX expression. Thus, the curly braces that enclose
the JavaScript code must be inside an element (such as a div
).
Also, valid JSX object must have exactly one outer, 'parent' element, but it can have any number of child elements. Remember, that when Babel converts the JSX to JavaScript, it is going to be turning your JSX into a React.createElement()
call, and if we look at the syntax for React.createElement()
:
React.createElement(
type,
[props],
[...children]
)
As you can see, the first argument must be a single element, in this case a 'div', but it could be any container element, such as a ul
.
When you uncomment your lines of code, you are putting all the planet elements inside the one container element, which is valid. When you have the lines commented, you have a pile of sibling elements but no parent, and therefore no single element to be the type
argument in createElement()
Hope that clears things up.
Kind regards,
Alex

Hakan Ergoksen
2,168 PointsHi Truong,
Babel compiler doesn't understand your code. Because you have syntax error. You must remove curly braces if you want to use JavaScript Expressions alone.
You should write it like this:
const PlanetList = (props) => {
return (
props.planets.map(planet =>
<Planet
key={planet.id}
name={planet.name}
diameter={planet.diameter}
moons={planet.moons}
desc={planet.desc}
url={planet.url}
/>
)
);
}
You can also write this way:
const PlanetList = (props) => {
props.planets.map(planet =>
<Planet
key={planet.id}
name={planet.name}
diameter={planet.diameter}
moons={planet.moons}
desc={planet.desc}
url={planet.url}
/>
)
}
Bader Alsabah
4,738 PointsBader Alsabah
4,738 PointsI believe the return statement must always return a JSX object - not a JSX expression (which is what you have between curly braces). So as long as you return a JSX object (built in or custom) - you can insert within it as many different expressions as you want - assuming they're valid. I sometimes put console.logs between my JSX object opening and closing tags to debug my code.