Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial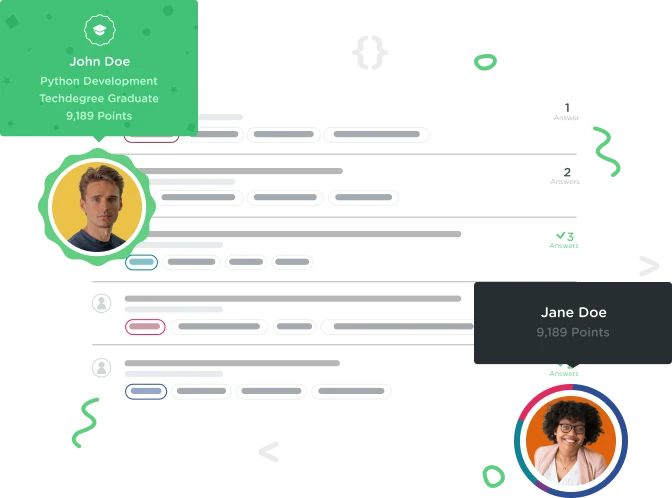
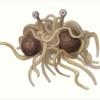
Anthony Gomez
Full Stack JavaScript Techdegree Student 10,089 PointsComponents as Classes vs Function or arrow functions.
in this course Guil Hernandez teach us how to create basic components using functions, but I've been wondering why not as a JS class from the beginning, using JS Classes save us a lot writing / time and the code it's much cleaner.
import React, { Component } from 'react';
class Header extends Component {
render() {
return (
<header>
<h1>Scoreboard</h1>
<span>Players:1</span>
</header>
);
}
}
export default Header;
4 Answers

Cooper Runstein
11,850 PointsThere is a big difference between function based components and class based components. Mainly class based components can have their own state -- this is not something you'll always want. You'll want only a few container components to have their own state, creating functional components makes sure you don't give state to components that shouldn't be managing state. Functional components can make things a lot easier to manage, you'll never be able to mutate state in a functional component, you can only interact with props. There are times where I only have a single class based component in my entire application with 20+ functional components.
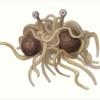
Anthony Gomez
Full Stack JavaScript Techdegree Student 10,089 Pointshum... I have to keep experimenting, I'm new in react, I've been working for a while with angularjs and angular 2, where I work we use AngularJS and we do angular components using ES6 JS Classes, I know it's a different story but I thought that there was not a significant difference between function and es6 clases besides the way we write them, like I explained above I like to uses classes makes the code cleaner and easier to read.
import React, { Component } from 'react';
// Easily import components bits.
import Header from './header/header';
import Player from './player/player';
/*
* No need of function declaration just class name and make sure it extends from component
*/
class Scoreboard extends Component {
render() {
/*
* If I wanted to use props I don't need to function Scoreboard(props)
* just {props.prop}
*/
return (
<div className="scoreboard">
<Header title="Scoreboard" totalPlayers={1}></Header>
<Player></Player>
</div>
);
}
}
export default Scoreboard;

Cooper Runstein
11,850 PointsI would disagree, classes and functions are equally easier to read, but remember that 'classes' in JavaScript are more special syntax than anything else. In React all data flows top down, functional components essentially force you to follow that model. You can use classes for all components, but that gives every component the ability to run lifecycle methods and hold state. This is normally not a good thing, only top level components should be doing that sort of thing.

Cooper Runstein
11,850 PointsI think a lot of it is personal preference, I know a lot of guys a lot better at React than I am have advised me to use functional components where I can, so that's what I do. I like the fact that you don't ever need to worry about this/binding issues, they're often easier to test, and they're always dumb components no matter what. If you work in a team, functional components are also a really obvious sign to others that might add to your code that the component is not meant to directly touch state or do much other than present itself.
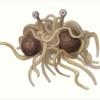
Anthony Gomez
Full Stack JavaScript Techdegree Student 10,089 PointsCooper Runstein you may be right, I'll try to read some more around the Internet, according to Guil Hernandez there isn't anything wrong with just declaring your components using classes from the beginning.
Again there are standards set by the community that are good to follow, I'll check the Airbnb react style guide.
https://github.com/airbnb/javascript/blob/master/react/README.md
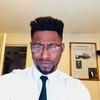
Aurelius Calliste {Callicodes}
8,196 PointsHi guys
Interesting discussion you are having and I learned a lot from what I read. I am a newbie with React and wondered why Guil chose to remain with the curly braces and parentheses. Isn't it also cleaner to remove them?
Thanks
p.s. Treehouse is absolutely brilliant and thank you for all of your great tutorials.
Aurelius
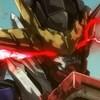
Blake Morrison
9,665 PointsI'm guessing Guil did that to explicitly show what is being returned from the component

fairest
Full Stack JavaScript Techdegree Graduate 19,303 PointsThe Airbnb React/JSX Style Guide has a paragraph on this subject that makes sense: If you don’t have state or refs, prefer normal functions (not arrow functions) over classes.
They also advise to steer away from arrow functions: If you don’t have state or refs, prefer normal functions (not arrow functions) over classes. What is your opinion in this?
Anthony Gomez
Full Stack JavaScript Techdegree Student 10,089 PointsAnthony Gomez
Full Stack JavaScript Techdegree Student 10,089 PointsAlso thanks a lot for this awesome course, I really like every course you give Guil Hernandez, awesome work, keep it that way :).