Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial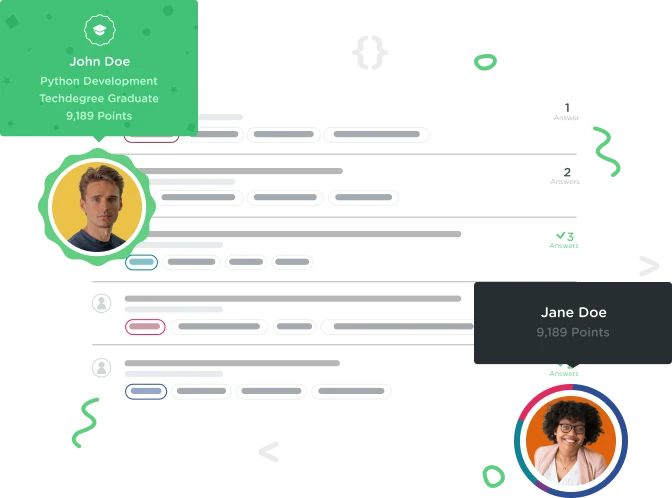

Josh Boersma
Courses Plus Student 2,486 PointsComputed Properties
I don't understand how computed properties work. I can't tell how they are different from methods because they look the exact same. I am supposed to code a computed property named Area that returns the area of the square, but every solution I have provided is rejected. Any pointers would be much appreciated.
namespace Treehouse.CodeChallenges
{
class Square : Polygon
{
public double SideLength { get; private set; }
public Square(double sideLength) : base(4) {
SideLength = sideLength;
}
public double Area(){
get {
return SideLength * SideLength;
}
}
}
}
namespace Treehouse.CodeChallenges
{
public class Polygon
{
public int NumSides { get; private set; }
public Polygon(int numSides)
{
NumSides = numSides;
}
}
}
1 Answer
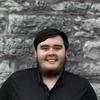
Michael Hulet
47,912 PointsComputed properties don't quite look like methods. Namely, since they will never take any parameters by definition, you don't use parentheses to define them or call them. You'll just use the get
keyword with brackets, and put the code you need to compute its value between those brackets. It'd look something like this:
namespace Example
{
class Triangle : Polygon
{
public double baseLength{ get; private set; }
public double height{ get; private set; }
public double Area{
get{
return 0.5 * baseLength * height;
}
}
}
}
Josh Boersma
Courses Plus Student 2,486 PointsJosh Boersma
Courses Plus Student 2,486 PointsI changed my code to mirror yours, but it still failed to compile (I'm at work and can't look at errors cause web filter). Do you have any other tips. Your suggested code (my question's code is updated to match) is the first thing I tried. I'm not sure what they're looking for.
Michael Hulet
47,912 PointsMichael Hulet
47,912 PointsWhen I changed my code to be similar, I passed the challenge, so it'd be helpful to know the error messages you're getting. I'd love to help once you get back from work and see what they are! Also, it's important to note that my code above doesn't pass the challenge because it's doing something different than what is asked. It illustrates the concept of why your code above doesn't pass, but Treehouse doesn't like it when someone posts an answer that can be copied and pasted directly into a challenge and pass, so I made mine a bit different than what the challenge wants