Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial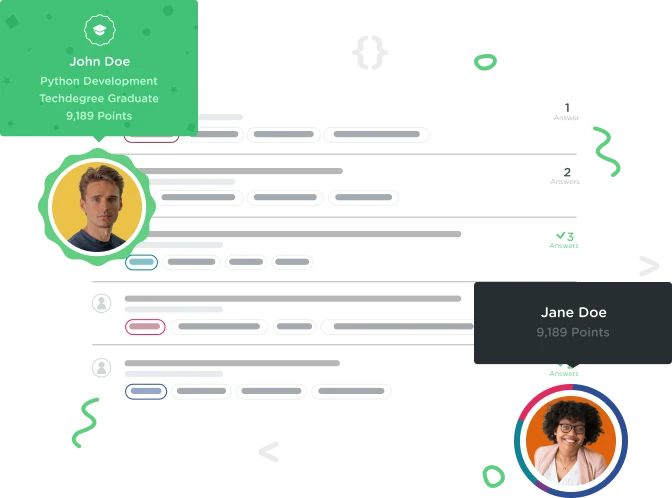

Derek Aldana
1,095 PointsComputed Propeties
I have watched the video a couple of times now and I cant figure this method of coding out. I dont get it. I need to write the computed property. I have tried multiple ways now and I cant get it.
namespace Treehouse.CodeChallenges
{
class Square : Polygon
{
public double readonly area;
public double SideLength { get; private set; }
public double Area {get {area}}
public Square(double sideLength) : base(4)
{
SideLength = sideLength;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Polygon
{
public int NumSides { get; private set; }
public Polygon(int numSides)
{
NumSides = numSides;
}
}
}
3 Answers

andren
28,558 PointsA computed property is essentially just a method that gets treated like a property. In the sense that it can be used exactly like a property would be used normally but when you reference it you don't just pull out a value already stored in it like you do with normal properties, you actually run a method and get what the method ends up returning.
You place the method you want to run in brackets directly following the get keyword. So if you for example wanted a computer property that was called TwoPlusTwo that simply calculated what 2+2 is each time it was called it would be written like this:
public int TwoPlusTwo {
get {
return 2 + 2;
}
}
That computed property would of course return 4, and while it's a fairly useless computed property it does show you how computed properties are structured. In order to calculate the area of a square you multiply the sides together, so the solution to this challenge simply looks like this:
public double Area {
get {
return SideLength * SideLength;
}
}
You don't need to define any read only properties or anything like that to solve this challenge.
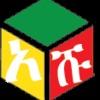
Ashenafi Ashebo
15,021 Pointsnamespace Treehouse.CodeChallenges { class Square : Polygon { public double SideLength { get; private set; }
public double Area { // This is a property but treated as the method because it is a computed property.
get {
return SideLength * SideLength;
}
}
public Square(double sideLength) : base(4)
{
SideLength = sideLength;
}
}
}

Edward Ries
7,388 PointsThe computed property needs to look more like this. It's important to include the return; public double Area { get { return SideLength * SideLength ; } }

Derek Aldana
1,095 PointsThanks!
Derek Aldana
1,095 PointsDerek Aldana
1,095 PointsOk cause it kept asking if my variable was a readonly which is why I kept doing that. Thanks a bunch!!!! Cleared it up nicely.