Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial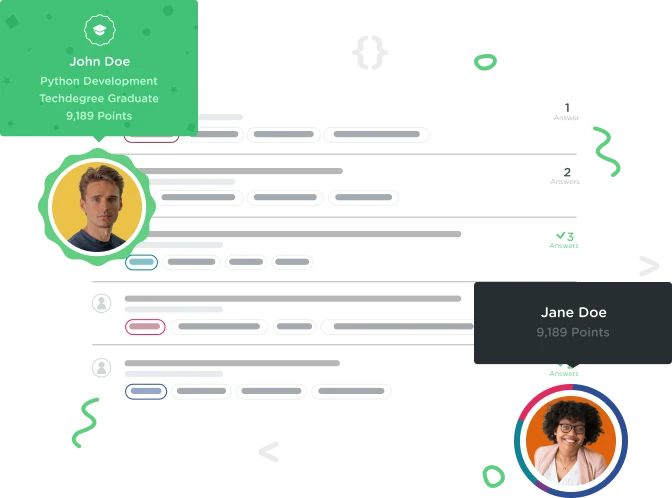
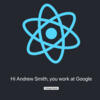
drew s
Python Development Techdegree Graduate 19,491 PointsComputer Science Java: My program is not running. In addition I am fully sure I did this correctly. I need help.
a) Write a program that reads two numbers from the keyboard representing, respectively, an investment and an interest rate (you will expect the user to enter a number such as .065 for the interest rate, representing 6.5% interest rate). Your program should calculate and output (in $ notation) the future value of the investment in 5, 10 and 20 years using the following formula future value = investment*(1 + interest rate)^year You will assume that the interest rate is an annual rate and is compounded annually.
b) Write a program that reads a commercial website URL, from the keyboard, you should expect that the URL starts with www. and ends with .com. Retrieve the name of the list and output it. For instance, if the user inputs
Here's What I did:
import java.util.*;
import java.lang.*;
import java.io.*;
class ProgAsssign1{
public static double getFutureValue(int investment, double interestRate, int year){
return Math.pow(investment * (1 + interestRate), year);
}
public static void main(String[] args)throws java.lang.Exception{
int investment;
double interestRate;
Scanner a = new Scanner(System.in);
System.out.println("Enter the investment amount: ");
investment = a.nextInt();
System.out.println("Enter the interest rate: ");
interestRate = a.nextDouble();
double futureValue = getFutureValue(investment, interestRate, 5);
System.out.println("Future value after 5 years is: " + futureValue);
futureValue = getFutureValue(investment, interestRate, 10);
System.out.println("Future value after 10 years is: " + futureValue);
futureValue = getFutureValue(investment, interestRate, 20);
System.out.println("Future value after 20 years is: " + futureValue);
}
}
import java.io.*;
import java.util.*;
import java.util.Scanner;
import java.text.*;
import java.math.*;
import java.util.regex.*;
public class ProgAsssign2{
public void site(Scanner in){
System.out.println("Enter number of websites: ");
int n = in.nextInt();
for(int i = 0; i < n; i++){
System.out.println("Enter the URL of the websites");
String s = in.next();
String pattern = "(([wW]{3}.) [-a-zA-ZO-9+&@#/%?=~_|!..,.;]*(.[cC][oO][mM]|.[eE][dD][uU]))";
if(s.matches(pattern)){
String[] a = s.split("[wW]{3}.");
String[] b = a[1].split("[cC][oO][mM].[eE][dD][uU]");
System.out.println("The name of the website is '" + b[O] + "'");
}
else{
System.out.println("Please enter URL");
}
}
in.close();
}
}
3 Answers
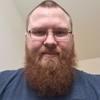
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsWhat error message are you getting?

Yanuar Prakoso
15,196 PointsHi Felix
I will answer the question a):
I try to recreate your code and it runs in my IDE but your formula to calculate Future Value is wrong. This is my correction:
public static double getFutureValue(int investment, double interestRate, int year){
//return Math.pow(investment * (1 + interestRate), year);<--this is worng since it calculate(invest*(1+interest))^year
return investment * Math.pow((1+interestRate), year);//<- this is how you should calculate
}
I hope this can help a little. PS: I failed to see why you need to import java.lang.* and java.io.* , since as far as I know you only need to import the Scanner's package which is the java.util.Scanner. Well it might just me. Forgive my lack of knowledge

Yanuar Prakoso
15,196 PointsAs for the question b:
I have recreate and your code in my IDE, please note since I am only testing your code I directly put it in Main class and main method:
package com.teamtreehouse;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// write your code here
Scanner in = new Scanner(System.in);
System.out.println("Enter number of websites: ");
int n = in.nextInt();
for(int i = 0; i < n; i++){
System.out.println("Enter the URL of the websites");
String s = in.next();
//String pattern = "[wW]{3}.[a-zA-Z0-9+&@#/%?=~_|!.,;]*(.[cC][oO][mM]|.[eE][dD][uU])";<-you don't need this
if(s.matches("[wW]{3}.[a-zA-Z0-9+&@#/%?=~_|!.,;-]*(.[cC][oO][mM]|.[eE][dD][uU])")){//<-this how you should use String.matches(regex) method
String[] a = s.split("[wW]{3}.");
String[] b = a[1].split(".[cC][oO][mM]|.[eE][dD][uU]");//<- this is the correct regex
System.out.println("The name of the website is '" + b[0] + "'");
}
else{
System.out.println("You have entered invalid URL");//<-- I changed this
}
}
in.close();
}
}
First thing first since I am using Main class I declared Scanner as new object not passed argument. Then the most important point here is when you put s.matches(regex) you need to define the regex inside literally (at least in my IDE it is) that is why your code failed to read your patterns (maybe).
If you insist to declare the regex pattern in separate variable and since you already imported java.util.regex.*;, you must declare the regex not the String. Like this:
System.out.println("Enter the URL of the websites");
String s = in.next();
Pattern pattern = Pattern.compile("[wW]{3}.[a-zA-Z0-9+&@#/%?=~-_|!.,;]*(.[cC][oO][mM]|.[eE][dD][uU])");
Matcher matcher = pattern.matcher(s);
if(matcher.matches()){
//and so on...
}
Then in your pattern regex I add '-' character since some website (although not recommended) can put it in their address.
The most important point here let's start from the most obvious: why do you put println("Please enter URL"); in the **else statement? You do not provide any loop when the user input invalid URL. It is more appropriate since you terminate the process when the users input invalid URL is to give them message You have entered invalid URL
Okay next point: from what I can read from your code is you want to split the URL or most likely host name address from for instance www.google,com or www.ocw.mit.edu to google and ocw.mit respectively.
In order to do that your regex in the ** String[] b = a[1].split(".[cC][oO][mM].[eE][dD][uU]");** is incorrect. If you keep using that regex the it will return google.com and ocw.mit,edu rather the intended format.
You need to add OR pattern | between them. That is what I did in my code upstairs.
I hope this can help a little. Any other inputs are welcomed. Please forgive my lack of knowledge in regex and coding I try my best to give the most useful answer possible.