Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial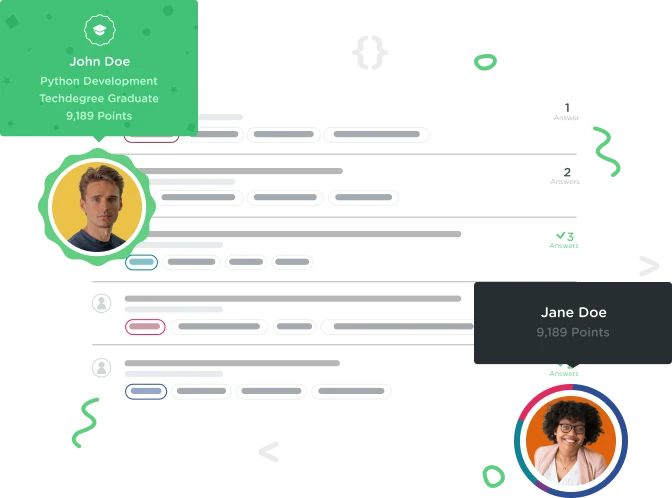
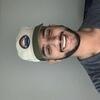
heebers
Python Development Techdegree Student 1,835 PointsConcatenation and Memory Storage With Mutable and Immutable Objects (Mainly Tuples and Lists)
Hello,
I am building upon another thread that asked a similar question, however I feel the need to build off that in order to build my domain knowledge on this topic.
When creating a variable that is a tuple and concatenating with another tuple but storing that result in the same variable name prior to concatenation the object id types are different. This is expected since tuples are immutable and the original object is disposed of while the variable name is now referencing our new object. (Please see code snippet below)
obj1 = 1,2,3,4,5
print(id(obj1))
obj2= 6,7,8,9,10
obj1 = obj1+obj2
print(obj1)
print(id(obj1)) #The id of this object is not the same as when we printed it earlier (As expected)
However, when we follow the same process for lists the id of the object prior to concatenation and after is actually different. This is where I'm confused and please correct me If I am not thinking of this properly. However, since lists are mutable shouldn't concatenation modify the original object so in this case there is no actual disposal of the object prior to concatenation (and therefore no variable reassignment occurred). Therefore, shouldn't the object id's be the same?
list1 = [1,2,3,4]
list2 = [1,2,3,4]
print(id(list1))
list1 = list1 + list2
print(id(list1))
1 Answer
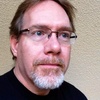
Chris Freeman
Treehouse Moderator 68,457 PointsHey heebers, great question!
The right hand equation list1 + list2
creates a new list each time it evaluates. It is this new list that gets a new id
and then is pointed to by list1
.
>> list1 = [4, 5, 6]
>>> list2 = [3, 2, 1]
>>> id(list1), id(list2)
(140148603620800, 140148602798720)
>>> list1 + list2
[4, 5, 6, 3, 2, 1]
>>> id(list1 + list2)
140148602799168
>>> id(list1 + list2)
140148602798656
>>> list1 = list1 + list2
>>> id(list1)
140148602920896
Depending on the freed memory space, the newly created list may or may not happen to use the same memory location as a previous object. Above, the temp object is in two different locations. But since the object pointed to by list1
is "in use" when the new temp list is created, the temp list location will always be different then the original list1
. Thus, the new list1
will be located a the new address.
Edit: if the code was list1 += list2
then the list1
id would remain the same since this translates to an append operation on the original object.
Post back if you need more help. Good luck!!
heebers
Python Development Techdegree Student 1,835 Pointsheebers
Python Development Techdegree Student 1,835 PointsYes, thank you so much. I appreciate you taking the time to do this I was really caught up on this one.
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsInterestingly, if you use the incremental add
+=
then the ids remain unchanged:Using += calls
lst1._iadd_(lst2)
which preserves the id.heebers
Python Development Techdegree Student 1,835 Pointsheebers
Python Development Techdegree Student 1,835 PointsWhen using the += to increment is the ID preserved because python assumes we would not be changing the variable when incrementing it at the same time?
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 Points+=
calls the__iadd__
method of thelst1
that modifiesself
in place. No new object is created.heebers
Python Development Techdegree Student 1,835 Pointsheebers
Python Development Techdegree Student 1,835 PointsOh thatβs pretty neat