Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial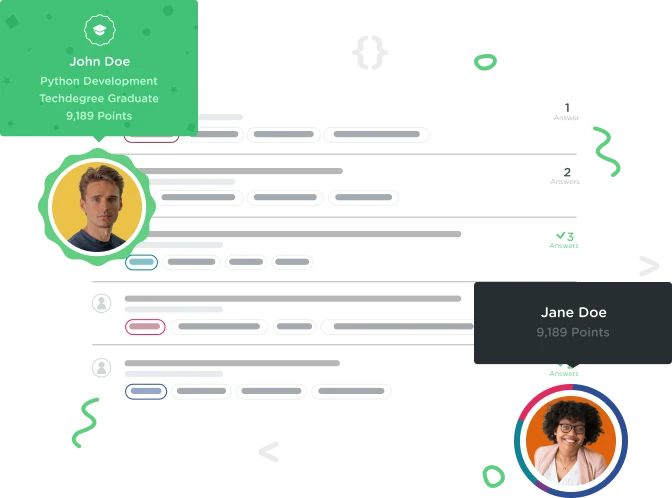
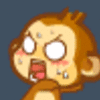
shadryck
12,151 PointsCondition statement can't see passed parameter when in while loop.
When running this small program it runs perfectly fine without the while loop, but when the conditional statement resides inside the while loop, it won't read the check parameter anymore. I'm guessing this is related to how scopes work. If someone is willing to explain me how I could do this better I would be grateful.
var stock = ['apples', 'eggs', 'milk', 'cookies', 'cheese', 'bread'];
var exitLoop = false;
function isInStock(check){
var checked = stock.indexOf(check);
while (exitLoop === false) {
if (checked >= 0) {
alert(check + " is in stock.");
exitLoop = true;
} else if (check.toLowerCase(", ") === 'list') {
alert(stock.join(', '));
exitLoop = true;
} else if(check === "" && check === null) {
alert(check + ' is not in stock.');
exitLoop = true;
} else {
alert("Something went wrong.");
break;
}
}
}
isInStock(prompt("Search for a product in our store. Type \'list\' to show all of the produce and \'quit\' to exit."));
2 Answers
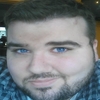
Marcus Parsons
15,719 PointsHey Vincent,
There are several errors in your code. The first one I noticed is that you have ", " inside of the toLowerCase()
method. The toLowerCase()
method doesn't take any parameters at all. It only acts on the string it's called on.
The next error I noticed is in your second else-if clause. The logic of that statement is incorrect. You shouldn't use an && (and) here because you want to check if check
is "" or null. Instead, you should use the || (or) operator.
However, the best way to exit the program is to check for falsey values of check or if check
is equal to "quit":
else if (!check || check === "quit") {
alert('Exiting program');
exitLoop = true;
}
This allows for users to just hit cancel or enter to exit out of the program because it checks for "", null, undefined, NaN, false, or 0. They can also type out "quit" if they'd like.
The bottom else statement should be for when a search doesn't return anything because this is when the A) the program didn't find anything in the list B) they didn't ask to list everything C) they didn't quit the program. This is when you would want to say that the search term isn't in stock.
So, you should change the bottom else to:
else {
alert(check + ' is not in stock.');
exitLoop = true;
}
Also, in your prompt, you are escaping the single quotes when you don't need to. This is only necessary if your outside quotes of the string were single quotes or if you were using double quotes inside of this string.
Also, I wouldn't quit the program until the user wants to quit the program. So, I would prompt for a new value of check under each condition except for the quit condition.

Iain Simmons
Treehouse Moderator 32,305 PointsIt works for me in this JSFiddle I created with your code: https://jsfiddle.net/Lc8wL5bm/
Unfortunately, you aren't really handling the blank string or quit conditions properly.
An empty string coming from the prompt
function won't also be null
and you're not checking if they type quit
.
The search and list parts work fine though. They do need to get the string exactly right though (apple
won't return anything but apples
will).
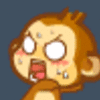
shadryck
12,151 PointsThank you. :)
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsHere is the fiddle I made with the updated code:
https://jsfiddle.net/mugtpxsn/1
shadryck
12,151 Pointsshadryck
12,151 PointsApologies for the slow responce. Thank you for correcting the mistakes. It works just fine now.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsNo worries, Vincent! You're very welcome :)