Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial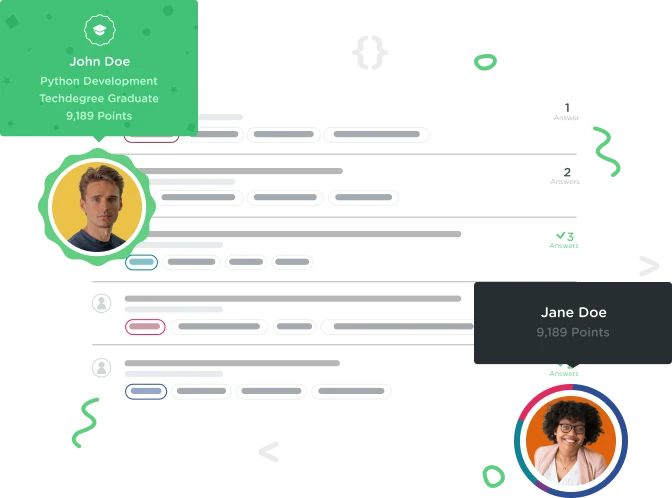

Srdjan Cestic
6,855 PointsConditional statement
Why my code don't pass challenge?
function max(11,22) { if ( 11 < 22 ) { return 22; } else { return 11; } }
5 Answers
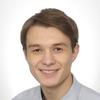
Sergey Podgornyy
20,660 PointsTry this code:
function max(a,b) {
if (a>=b)
return a;
else
return b;
}
You need to create function with arguments. This is main idea of function (to reuse them). After that you can use your function. For example:
max(11,22);
Read more about Functions here - http://www.w3schools.com/js/js_functions.asp

Srdjan Cestic
6,855 Pointsfunction max(10,2) { if (10 > 2) { return 10; } else { return 2; } alert( max('10,2') ); }
What's wrong with this code? Second challenge didn't pass...

Srdjan Cestic
6,855 PointsProblem is : SyntaxError: Unexpected number on first line (function).
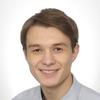
Sergey Podgornyy
20,660 PointsFirst, you need to define arguments as variables. Second, you need to call function outside the function body. Third, you need to send integers, as arguments.
function max(a, b) {
if (a > b) {
return a;
} else {
return b;
}
}
alert( max(10,2) );
In the last string you send to the function 10 as a
and 2 as b
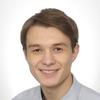
Sergey Podgornyy
20,660 PointsRead more about Functions here - http://www.w3schools.com/js/js_functions.asp

Srdjan Cestic
6,855 PointsI think I got it.
So function is some type of ''formula'' and I don't use the numbers in it, I use it to create the form, and later when I call function I add numbers like you said - alert( max(10,2) ); am I correct?
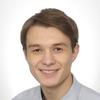
Sergey Podgornyy
20,660 PointsThe most basic benefit of having a concept of function is that it allows us to use the same function more than once in an expression with different parameter values.
Functions are "self contained" modules of code that accomplish a specific task. Functions usually "take in" data, process it, and "return" a result. Once a function is written, it can be used over and over and over again. Functions can be "called" from the inside of other functions.
Every function has its own Workspace. This means that every variable inside the function is only usable during the execution of the function (and then the variables go away).
More you can find here - http://www.cs.utah.edu/~germain/PPS/Topics/functions.html
In JavaScript are some predefined functions, such as alert
, prompt
, confirm
etc. That means, that you don't need to write dozens lines of code for alert some text, you just type name of function and send some text, as a parameter.
We can create user-defined functions, if we need to repeat some code more then one time. For example,
function title(name){
document.write("#####################################<br>");
document.write("########### "+name+" ###########<br>");
document.write("#####################################<br>");
}
This function create 3 lines with name of title. For call the function we just type a name of function and parameters in bracets:
title("Cats");
document.write("The domestic cat (Felis catus or Felis silvestris catus) is a small, typically furry, domesticated, and carnivorous mammal. They are often called house cats when kept as indoor pets or simply cats when there is no need to distinguish them from other felids and felines. Cats are often valued by humans for companionship and their ability to hunt vermin.<br>");
title("Dogs");
document.write("The domestic dog (Canis lupus familiaris or Canis familiaris) is a domesticated canid which has been selectively bred for millennia for various behaviors, sensory capabilities, and physical attributes.<br>");
This is much shorter as write same code a lot of times:
document.write("#####################################<br>");
document.write("########### Cats ###########<br>");
document.write("#####################################<br>");
document.write("The domestic cat (Felis catus or Felis silvestris catus) is a small, typically furry, domesticated, and carnivorous mammal. They are often called house cats when kept as indoor pets or simply cats when there is no need to distinguish them from other felids and felines. Cats are often valued by humans for companionship and their ability to hunt vermin.<br>");
document.write("#####################################<br>");
document.write("########### Dogs ###########<br>");
document.write("#####################################<br>");
document.write("The domestic dog (Canis lupus familiaris or Canis familiaris) is a domesticated canid which has been selectively bred for millennia for various behaviors, sensory capabilities, and physical attributes.<br>");
Code without functions take more place, that with function.

Srdjan Cestic
6,855 PointsSo my last question/claim is correct? Once I write function I can later use it to call it with different values?
Like function max above for example, function is write, name is max, parameters are letter (a,b), form of function is down (conditionals) and when I want to use function max I call it with console.log or alert etc. and name it with max and value in parenthesis (any numbers I want), like alert( max (2,10));
And with last comment I see that functions are used to write once and used whenever you need it, because it's not practical to write code over and over again.Functions make code simplier.
I know my understanding of this is pretty simple, but I need to know am I really understand this, not just passing challenge.
Thanks Sergey!
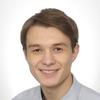
Sergey Podgornyy
20,660 PointsYes, you can reuse function any time you need. And yes, you can send to function different parameters, like max(10, 8);
or max(3,9);
. First number will be a
in function local scope, and second one will be b
in function local scope. But always be careful with parameters! If you create function with two parameters, you MUST call this function also with two parameters.
Correct, functions make code simpliers, it's main idea =) When you will learn Object-Oriented JavaScript, you'll fill how useful they are ;)
It looks like you understand this now. Way to go!

Srdjan Cestic
6,855 PointsThanks Sergey for answer and your time. :)
Srdjan Cestic
6,855 PointsSrdjan Cestic
6,855 PointsYour code work, but in challenge they said to put some random numbers.
When I change a and b with numbers challenge don't pass, I can't understand this part.
Sergey Podgornyy
20,660 PointsSergey Podgornyy
20,660 PointsTry JS random function
max(Math.floor((Math.random() * 10) + 1),Math.floor((Math.random() * 10) + 1));