Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial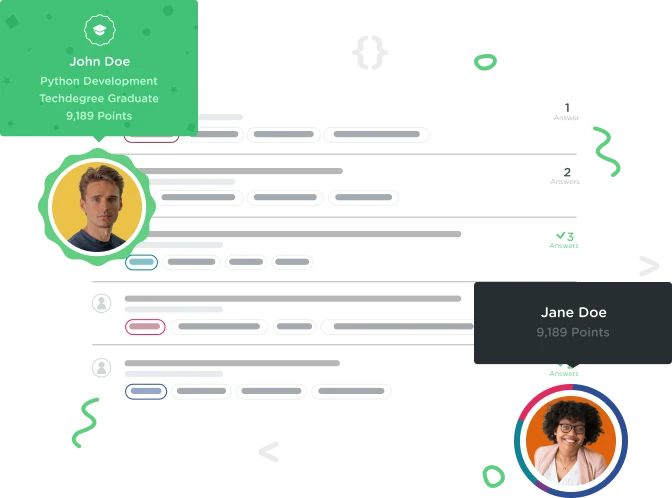
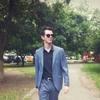
Ioan Ionkov
Courses Plus Student 6,921 Pointsconditional statement issue on PHP basics
I am trying to solve one of the tasks in the conditional section, the task itself is: "Check if each student has a GPA of 4.0. If the student has a GPA of 4.0, use the student's name variable to display "NAME made the Honor Roll". If not, use the variable to display "NAME has a GPA of GPA" and the error I am receiving is " Bummer! You need to check that $studentOneGPA is equal to 4.0". But I check for it and upon running the script on a server, everything is displaying as it should correctly. Here is my code:
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if ( $studentOneGPA >= 4.0)
echo "$studentOneName " . "made the Honor Roll ";
else
echo "$studentOneName " . "has a GPA of" . " $studentOneGPA";
if ($studentTwoGPA >= 4.0)
echo " $studentTwoName " . "made the Honor Roll";
else
echo " $studentTwoName " . "has a GPA of" . " $studentTwoGPA";
?>
Moderator edited: markdown was added to the question to properly render the code. Please see the Markdown Cheatsheet link at the bottom of the "Add an Answer" section for tips on how to post code to the Community.
2 Answers

Gamzat Mukailov
12,627 PointsI found the problem!
I dont know why but you have to use this code, because it is the only one which works.
<?php
$studentOneName = 'Dave';
$studentOneGPA = 3.8;
$studentTwoName = 'Treasure';
$studentTwoGPA = 4.0;
//Place your code below this comment
if ($studentOneGPA == 4.0) {
echo $studentOneName . " made the Honor Roll";
} else {
echo $studentOneName . " has a GPA of " . $studentOneGPA;
}
if ($studentTwoGPA == 4.0) {
echo $studentTwoName . " made the Honor Roll";
} else {
echo $studentTwoName . " has a GPA of " . $studentTwoGPA;
}
?> ```
I have it from: https://teamtreehouse.com/community/code-challenge-conditionals
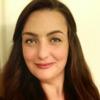
Jennifer Nordell
Treehouse TeacherHi there! You are correct, you should be using the == sign as the challenge requires it and will not accept the >=. However, it also seems to be demanding that your echo statements be contained within curly braces/brackets {}
. I'm fairly convinced that they're unnecessary when the code block only contains one statement, but it is causing the challenge to fail. Here's some pseudocode to show what they're wanting.
if ($this == $that) {
echo "They're equal.";
}
else {
echo "They're not equal.";
}
Give it another shot using the double equals sign and curly braces/brackets around your if else blocks.
Hope this helps!
Ioan Ionkov
Courses Plus Student 6,921 PointsIoan Ionkov
Courses Plus Student 6,921 PointsOther than the >= operator, I have also tried doing it with ==