Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial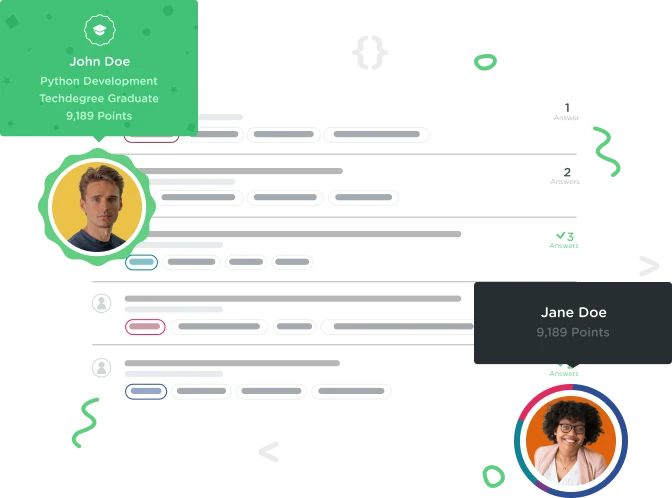

Nick Huemmer
Front End Web Development Techdegree Graduate 26,840 PointsConditional statement not working - what's wrong with my syntax?
I'm trying to solve this challenge using a conditional statement with my function, but I'm not sure what's wrong with my syntax?
I'm using if else
, but JS isn't liking how I've concocted the solution.
const inputLow = prompt('Please provide your lowest number');
const inputHigh = prompt('Please provide your higher number');
const lowerNumber = parseInt(inputLow);
const upperNumber = parseInt(inputHigh);
const main = document.querySelector('main');
if (inputLow >= 0 && inputHigh) {
function getRandomNumber(lowerNumber, upperNumber) {
const randomNumber = Math.floor(Math.random() * (upperNumber - lowerNumber) ) + lowerNumber;
return main.innerHTML = `${randomNumber} is a random number between ${lowerNumber} and ${upperNumber}`;
} else {
main.innerHTML = 'You need to provide two integers (whole numbers greater than zero), try again.'
console.log('It did not work!')
}
}
There's something funny happening around the else
but I haven't been able to figure out what it is.
It took me some time to figure out the Markdown for this code block, it now includes all the code. I can include the Jsdoc if it's helpful.
1 Answer
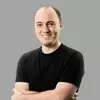
Josh Thackeray
9,895 PointsHi Nick,
It looks like your issue here is that your placement of the if
statement and the function
declaration are interfering with each other in how the curly brackets are placed.
If you take a look at the following block, can you see how you have opened an if
statement and then immediately opened your function
declaration? This is fine, however as you've opened them in this order you must then close the function before having your } else {
//Before
if (inputLow >= 0 && inputHigh) {
function getRandomNumber(lowerNumber, upperNumber) {
const randomNumber = Math.floor(Math.random() * (upperNumber - lowerNumber) ) + lowerNumber;
return main.innerHTML = `${randomNumber} is a random number between ${lowerNumber} and ${upperNumber}`;
} else {
main.innerHTML = 'You need to provide two integers (whole numbers greater than zero), try again.'
console.log('It did not work!')
}
}
//After
if (inputLow >= 0 && inputHigh) {
function getRandomNumber(lowerNumber, upperNumber) {
const randomNumber = Math.floor(Math.random() * (upperNumber - lowerNumber) ) + lowerNumber;
return main.innerHTML = `${randomNumber} is a random number between ${lowerNumber} and ${upperNumber}`;
}
} else {
main.innerHTML = 'You need to provide two integers (whole numbers greater than zero), try again.'
console.log('It did not work!')
}
//Or in case you're wanting the if statement inside your function
function getRandomNumber(lowerNumber, upperNumber) {
if (inputLow >= 0 && inputHigh) {
const randomNumber = Math.floor(Math.random() * (upperNumber - lowerNumber) ) + lowerNumber;
return main.innerHTML = `${randomNumber} is a random number between ${lowerNumber} and ${upperNumber}`;
} else {
main.innerHTML = 'You need to provide two integers (whole numbers greater than zero), try again.'
console.log('It did not work!')
}
}
That should solve your syntax error.
I'm not sure if you've included the full code here so correct me if there's parts I'm missing however it doesn't look like you're calling this function anywhere in your code. I would also take a look at keeping your function specific to what it is you're calling it, in your case you've created a function to return a random number between 2 numbers. Take a look at my example below to see how I would lay this out.
const inputLow = prompt('Please provide your lowest number');
const inputHigh = prompt('Please provide your higher number');
const lowerNumber = parseInt(inputLow);
const upperNumber = parseInt(inputHigh);
const main = document.querySelector('main');
//Declaring the getRandomNumber function to be used in the near future
function getRandomNumber(lowerNumber, upperNumber) {
const randomNumber = Math.floor(Math.random() * (upperNumber - lowerNumber) ) + lowerNumber;
//only want this to return a number so I can decide what I want to do with this later in the script
//this keeps the function specific as by the name `getRandomNumber` it seems like something that would just return
//instead of writing anything
return randomNumber;
}
//We should now check if the input matches our validation
if (inputLow >= 0 && inputHigh) {
//Use our previously declared function to get the random number
const randomNumber = getRandomNumber(inputLow, inputHigh);
//and write this to the page
main.innerHTML = `${randomNumber} is a random number between ${inputLow} and ${inputHigh}`;
} else {
main.innerHTML = 'You need to provide two integers (whole numbers greater than zero), try again.'
console.log('It did not work!')
}
P.S. Apologies if there's any bugs in this, I haven't tested it after writing it out

Nick Huemmer
Front End Web Development Techdegree Graduate 26,840 PointsThanks for the help, Josh. I can see what you did with the curly braces between the function and conditional statement and I played around with the code some with some interesting results.
It will take me playing around with it a bit more to get it to work right. Again, thanks for the input!
Nick Huemmer
Front End Web Development Techdegree Graduate 26,840 PointsNick Huemmer
Front End Web Development Techdegree Graduate 26,840 PointsI'm using parseInt here instead of the +unary to make the strings integers just to make the code a bit more straightforward. That part of the code works when I remove the
if else
, it's just the conditional statements that aren't working here.I'm almost certain it has to do with syntax, but I'm not sure what exactly except that it has to do with the
else
.