Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial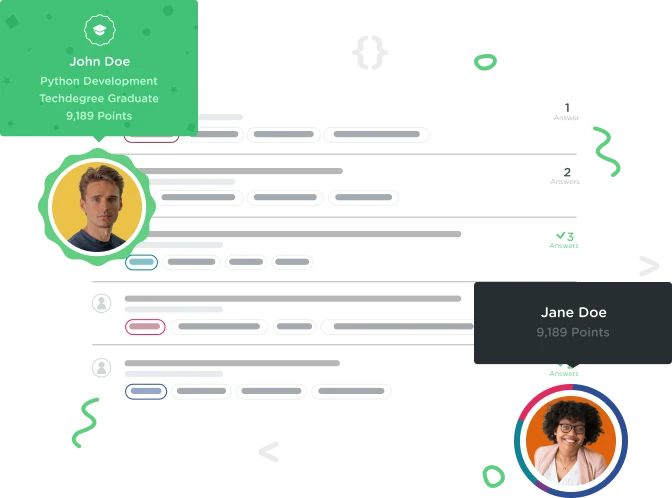
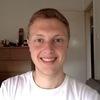
Samuel Cruz
9,794 PointsConditional statements inside a function. Using greater than operators???
Hi I have been stuck on this question now for about 5 hours. The question is: Create a new function named max which accepts two numbers as arguments (you can name the arguments, whatever you would like). The function should return the larger of the two numbers.
HINT: You'll need to use a conditional statement to test the 2 parameters to see which is the larger of the two.
It tells me that the 'max' function is not returning a number. Can someone look at my code and tell me what Im doing wrong and please give an answer please. Thanks, Im really all out of ideas Ive watched previous videos and I still cant figure it out, thank you.
function max(hip, hop){
if ( one < two ); {
var large = 2;
}return large;
}
var one = 1;
var two = 2;
max(one, two);
2 Answers
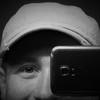
Ryan Rankin
8,261 PointsOkay, for this problem you're going to practice passing arguments to the function "max". The arguments are declared in the parenthesis like this "function max (argument1, argument2)." Like the problem says you can name the arguments whatever you would like, but remember you're passing numbers to them when you call the function. Your end result should look more simple like this.
function max (argument1, argument2) {
if (argument1 > argument2) {
return argument1;
} return argument2;
}
max(10, 5); //Here I'm changing the value of the arguments and it will return 10
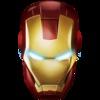
Michael Mroczka
40,979 PointsYou are declaring the variable called large only INSIDE the if-statement. So if one is less than two then it will make the variable called 'large' and set it equal to the number 2. Then it will exit the if statement and return the variable called 'large' with the number two in it just as you expected it to. But what happens if one is NOT less than two? It will skip the entire if-statement and just try to return the variable called 'large'. But you haven't declared a variable called 'large' yet since you never entered the if-statement! Try this instead...
function max(one, two){
var large = 0;
if ( one < two ); {
large = two;
}
else{
large = one;
}
return large;
}
var one = 1;
var two = 2;
max(one, two);
Alternatively, you could simplify it further by removing the large variable entirely and just directly returning the answer like this...
function max(one, two){
if ( one < two ); {
return two; // it made it into this if-statement, so we know two is largest and we can return it directly
}
return one; // it made it to this return statement, so we know it skipped the if-statement, meaning one is largest and we can return that instead
}
var one = 1;
var two = 2;
max(one, two);
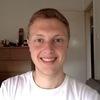
Samuel Cruz
9,794 Pointsthanks for reply
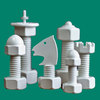
Steven Parker
230,274 PointsIf I had to guess why this answer keeps getting downvoted (not by me) it might be because while the comments made are valid, they don't really pertain to the challenge, nor help to clear up the confusion between variables and parameters.
Samuel Cruz
9,794 PointsSamuel Cruz
9,794 PointsThank you, Your example worked. But why didn't there have to be an 'else' clause just before 'return argument2;'?