Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial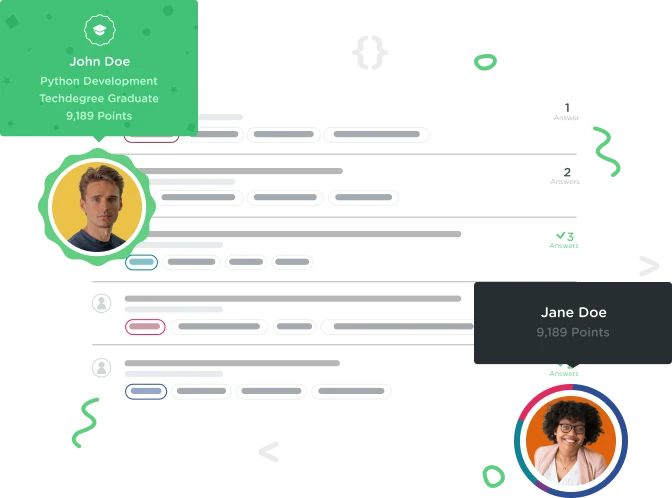

Drew Cook
14,612 PointsConfirm if wanting to delete an item
I figured if we were going to perfect this project, a good confirmation about deleting the items may make it more user friendly. What if the user accidentally hits the delete button and didn't want to actually remove the item? A good confirming message would fix this.
I have figured it out but would like to challenge anyone who wants to do this.
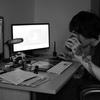
Andrew Robinson
16,372 PointsThis is what I came up with, it basically creates a div for the alert message, and a div container (position: absolute) so I could position the dialogue in the center of the page at the same location each time, if you click Yes, it deletes the task, if you click No, it just removes the dialogue.
http://port-80-iq0ldlzxig.treehouse-app.com/
// Create a dialogue with yes/no option, if yes delete, if no remove dialogue
var confirmDialogue = function() {
var listItem = this.parentNode;
var ul = listItem.parentNode;
var body = document.body;
var yesButton = document.createElement('button');
var noButton = document.createElement('button');
var divContainer = document.createElement('div');
var alertContainer = document.createElement('div');
alertContainer.className = 'alert';
yesButton.innerText = 'Yes';
noButton.innerText = 'No';
alertContainer.innerHTML = '<p>Delete this item?</p>';
alertContainer.appendChild(yesButton);
alertContainer.appendChild(noButton);
divContainer.setAttribute('id', 'overlay');
divContainer.appendChild(alertContainer);
body.appendChild(divContainer);
yesButton.addEventListener('click', function(){
deleteTask(ul, listItem, divContainer, body);
});
noButton.addEventListener('click', function(){
body.removeChild(divContainer);
return;
});
}
// Pass dialogue container and body container to deleteTask so it can also remove the dialogue
var deleteTask = function(ul, listItem, divContainer, body){
console.log('del');
ul.removeChild(listItem);
body.removeChild(divContainer);
}
// change deleteButton click event to confirmDialogue
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log('bind list item events');
var checkBox = taskListItem.querySelector('input[type=checkbox]');
var editButton = taskListItem.querySelector('button.edit');
var deleteButton = taskListItem.querySelector('button.delete');
editButton.onclick = editTask;
deleteButton.onclick = confirmDialogue;
checkBox.onchange = checkBoxEventHandler;
}
#overlay {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(255, 255, 255, .7);
}
.alert {
margin: 200px auto;
padding: 10px;
text-align: center;
width: 200px;
height: 100px;
background: white;
border-radius: 5px;
box-shadow: 0 1px 5px rgba(0, 0, 0, .3);
}
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsOr how about adding Undo / Redo functionality? :)