Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial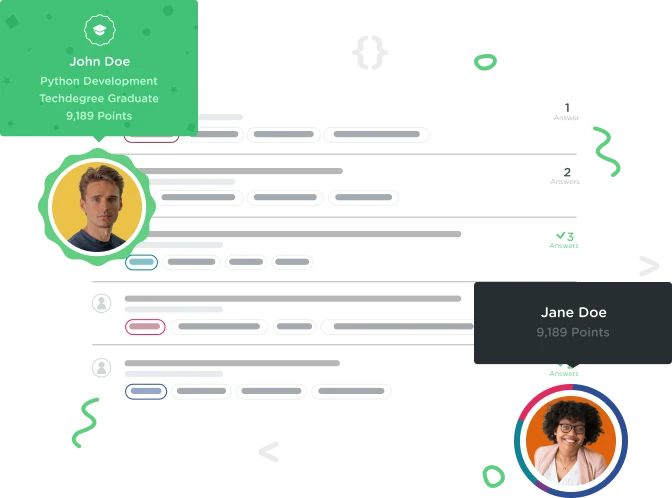
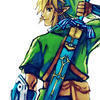
Dhruv Patel
8,287 PointsConfused
Am I doing the right thing? I know a datetime object holds a minute attribute so why does it not pass?
def minutes(dt1, dt2):
return round(dt2.minute - dt1.minute).minute
8 Answers
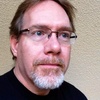
Chris Freeman
Treehouse Moderator 68,423 PointsYou can subtract two datetime
objects to get a timedelta
object. The attribute datetime.minute
is an int
. Subtracting two integers gives back an integer which has no minute
attribute.
the approach of focusing on the minute differences ignores that other attributes of the two datetime
objects, such as year, hour, may also be different. Subtract the two datetime
objects directly, then obtain the difference in minutes from the timedelta
object. Note timedelta
does not have a minutes
attribute:
# from help(datetime.timedelta):
Methods:
|
| total_seconds(...)
| Total seconds in the duration.
Using timedeltas:
# Task 1 of 1
# Write a function named 'minutes' that takes two datetimes and returns
# the number of minutes, rounded, between them. The first will always be
# older and the second newer. You'll need to subtract the first from the
# second.
def minutes(dtime1, dtime2):
deltat = dtime2 - dtime1
return round(deltat.total_seconds() / 60)
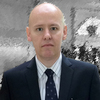
Nathan Tallack
22,159 PointsYou are going to want to use the timestamp method on the datetime value to convert it to the number of seconds since epoch. Then subtract the older from the newer and round the difference divided by 60 (to convert to minutes).
Your code would look something like this.
def minutes(dt1, dt2):
return round((dt2.timestamp() - dt1.timestamp()) / 60)
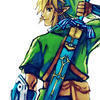
Dhruv Patel
8,287 PointsThanks
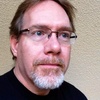
Chris Freeman
Treehouse Moderator 68,423 PointsWhy was Nathan's answer downvoted?
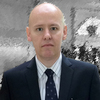
Nathan Tallack
22,159 PointsI downvoted it myself to draw more attention to your cleaner way (which i upvoted). ;)
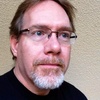
Chris Freeman
Treehouse Moderator 68,423 PointsNathan, your answer isn't wrong so I wouldn't downvote it. As Best Answer, your solution will be presented at the top regardless of the votes. If you prefer, the best answer can be switch to my answer. Yours can remain as a valid alternate solution.
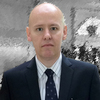
Nathan Tallack
22,159 PointsThat sounds like a plan. Mark that as best answer. :)
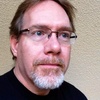
Chris Freeman
Treehouse Moderator 68,423 PointsChanging Best Answer per request.

Andrea Carlevato
2,277 PointsI just want to flag that the unclarity in challenges description is an obvious pattern in this Track. The author should get it together and fix the wording. Exercises are very simple in themself but are made frustrating by the very poor english and structure used.

Chris Robinson
4,491 PointsI'm really confused by the wording of this question. Am I the only one?
"Write a function named minutes that takes two datetimes and, using timedelta.total_seconds() to get the number of seconds, returns the number of minutes, rounded, between them."
1) Write a function named minutes that takes two datetimes and, 2) using time delta.total_seconds() to get the number of seconds, 3) returns the number of minutes, 4) rounded, 5) between them.
Lots of commas in a lot of places that make the flow of thought a bit difficult for me. And should #3 sound more imperative? Should it say 'return' rather than 'returns'? Something about this just seems off to me. Just thinking out loud. Anyone else experience this confusion?
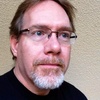
Chris Freeman
Treehouse Moderator 68,423 PointsHey Chris, no you're not the only one who has been confused by this challenge wording.
Sometimes the jargon when describing a task in a particular language takes getting used to. In this case, part of the challenge is to parse the description. I approach a challenge such as this by breaking the description into three parts: 1) signature description (the function name and parameter interface) aka "inputs", 2) hints on body of function, aka "the guts", and 3) the returned object description, aka "outputs".
Looking at the comma-infested description, I see:
- "Write a function named
minutes
that takes twodatetimes
..." (input) and, - "using
timedelta.total_seconds()
to get the number of seconds..." (guts), - "returns the number of minutes, rounded, between them." (output)
If the returns part of the sentence were broken out to another sentence, the imperative form, "Return the number...", would work fine. In the same sentence, "Write a function... that... returns the number of minutes...", the plural is correct.
Kenneth likes to construct challenges that make you think and ask questions. If everything was "obvious" you're not being challenged enough. Anytime something needs clarification, continue to seek help in the forum. All levels of questions are welcome! Keep up the good work!

Greg Welches
58 Pointswe are all confused
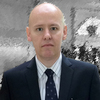
Nathan Tallack
22,159 PointsSo what chris is saying is that we dont have to use the timedelta method I used to get two ints out and then work with them in the complex way I did. We could use another method called timedelta that allows me to pass in two times and get that result back.
Much cleaner. :)

Greg Welches
58 Pointswe are all confused
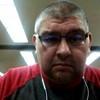
micram1001
33,833 PointsReading the question is difficult for me. I had to break down the question.
Write a function named minutes that takes two datetimes and, using timedelta.total_seconds() to get the number of seconds, returns the number of minutes, rounded, between them. The first will always be older and the second newer. You'll need to subtract the first from the second.
Writing a function is easy
def minutes():
add two parameters
(datetime1, datetime2)
use timedelta.total_seconds(), return in minutes rounded
example 3600 seconds 1 minute is equal to 60 seconds. so divide by 60 3600/60 = 60 minutes
datetime1 (older) datetime2 (newer)
subtract datetime1 - datetime2
I understood the return but not the round function until reading the above solutions.
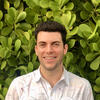
justlevy
6,325 PointsDoes this work?
Based on the question requirements I don't think it's correct since I didn't pass two parameters when declaring the function (eg... def minutes(dt1, dt2)
)
Thanks!
import datetime
def minutes():
dt2 = datetime.datetime.now()
dt1 = datetime.datetime(1988, 5, 12)
difference = (dt2 - dt1)
print(round(difference.total_seconds()/60))
print(type(difference))
minutes()
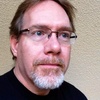
Chris Freeman
Treehouse Moderator 68,423 PointsHey justlevy, It has the gist of the solution. This i,ssue, as you mention, the function does not accept arguments. a simple fix would be to change the first few lines to:
def minutes(dt1, dt1):
difference = (dt2 - dt1)
The second change would be to return
the difference rather than print
it.
Post back if you need more help.
Nathan Tallack
22,159 PointsNathan Tallack
22,159 PointsOh wow! I did not think of this! And this is why we should read class references. ;)
Hara Gopal K
Courses Plus Student 10,027 PointsHara Gopal K
Courses Plus Student 10,027 PointsHi Chris,
if timedelta.total_seconds() returns a value in seconds, why divide by 60 ?
Thank You
Taig Mac Carthy
8,139 PointsTaig Mac Carthy
8,139 PointsNot all heroes wear capes