Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial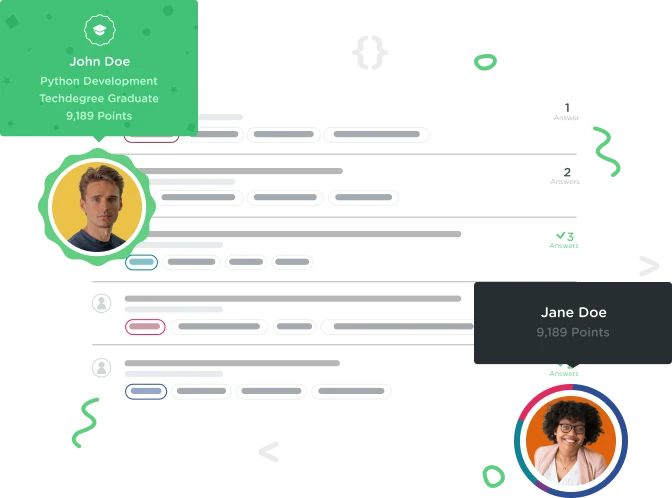

richard shu
Courses Plus Student 390 Pointsconfused
i genuinely don't know what the task is asking me to do.
3 Answers
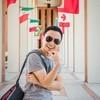
hamsternation
26,616 PointsSo to answer your question, basically, anything with parenthesis is a function. "if", "del", "in" are statements and not exactly the same as functions.
Functions are things that takes in arguments, some examples:
print('hello world')
int('1234567')
range(1,5)
the way a function is created is using the "def" statement. Notice again that def
is a keyword and a statement but it's not a function in itself.
a function can be thought of for now as a bunch of statements combined together.
so going back to how a function is created, we start with "def" and then we name the function as such:
def printer(something):
what this did is created a function printer that takes an argument, "something". That allows us to use this function you created later without writing the code again like this:
printer(5)
printer(6)
printer(7)
But the problem is that right now, our defined function, printer doesn't do anything, since there's nothing inside the function.
to do so, we have to take our function and add what we want to do to it, like this:
def printer(something):
print(something * "Hi ")
notice that something inside the printer function matches exactly with the word something inside the print function. This is a variable that means print("whatever number the person typed" * "Hi "), so continuing from the above example,
printer(5)
# gets translated by the function into:
print(5 * "Hi ")
Hi Hi Hi Hi Hi
printer(6)
# gets translated by the function into:
print(6 * "Hi ")
Hi Hi Hi Hi Hi Hi
printer(7)
# gets translated by the function into:
print(7 * "Hi ")
Hi Hi Hi Hi Hi Hi Hi
notice that once you def the function printer once, all you have to do is type printer(some_number)
and you run the function.
I hope this was an easier explanation than the documentations. Keep at it! It gets easy once you understand it!
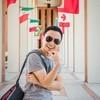
hamsternation
26,616 PointsThe challenges wants you to create a function that takes in a number input.
For example:
print_hi(5)
the result should be the word "Hi " 5 times, such as this:
>>>print_hi(5)
"Hi Hi Hi Hi Hi"
Let me know if that answers your question of if you need more help. :)

richard shu
Courses Plus Student 390 Pointsprinter = print("Hi " * 5) does that make printer a variable which is why it doesn't work? so a function is like print, del, if, in. and so on. right? am i creating a new function? it feels overwhelming complicated lol.

akhter ali
15,778 PointsThis challenge wants you to create a function that takes an argument.
First you can create a function, then add an argument to that function. Arguments can also be named parameters. I suggest you look at the examples here. https://www.tutorialspoint.com/python/python_functions.htm
Once you've created that, it wants you to print "Hi" as many times as the argument/parameter. Meaning if I call the function printer(5) it will print "Hi" 5 times. I suggest you read the question here. Just remember it wants you to print this value. https://stackoverflow.com/questions/6024522/can-perl-do-multiplying-a-string-like-python
richard shu
Courses Plus Student 390 Pointsrichard shu
Courses Plus Student 390 Pointsdude thankyouuu sooooo muchhh
richard shu
Courses Plus Student 390 Pointsrichard shu
Courses Plus Student 390 Pointsthis really helped it like blew my mind into a bigger world, thankyou
hamsternation
26,616 Pointshamsternation
26,616 PointsHaha I felt the same way when I learned about it. It's crazy how powerful these things are!