Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial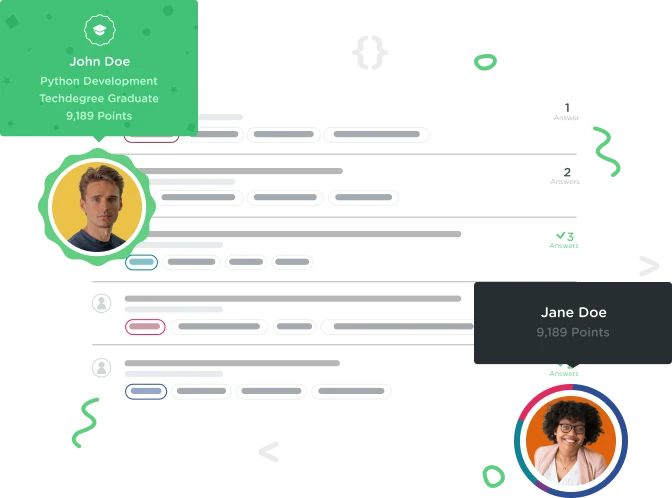

Karar Al-Shanoon
iOS Development with Swift Techdegree Student 300 Pointsconfused
hi, can someone help me
// Enter your code below
let value = 200
let divisor = 5
let someOperation = 20 + 400 % 10 / 2 - 15
let anotherOperation = 52 * 27 % 200 / 2 + 5
// Task 1 - Enter your code below
let result = 200 % 5
// Task 2 - Enter your code below
0 == result
let isPerfectMultiple = true
let someOperation = 5
let anotherOperation =7
someOperation =< anotherOperation
let isGreater = true
3 Answers
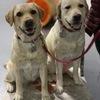
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Karar,
You have a couple of errors in Task 1, although by coincidence your code is passing the check:
- In Step 1 you are supposed to be using the constants
value
anddivisor
but you are using number literals. - In Step 2 you want to assign to the constant
isPerfectMultiple
whatever the result of comparing result and 0 is. You are comparing result and 0 but not doing anything with the value. Then you are assigningtrue
toisPerfectMultiple
regardless of what the result of the comparison is.
In Task 2, The wording of the challenge is very unclear. The first paragraph implies you need to know whether someOperation is greater, less, or equal to anotherOperation, but in the second paragraph it wants you to only use the greater or equal than operator to perform a single comparison. Ignore the first paragraph and concentrate on the second paragraph: you need to assign to isGreater
the result of comparing (using the >=
operator) someOperation
and anotherOperation
. You have a few issues:
- you are attempting to assign a value to
someOperation
. You are not being asked to do that, and moreover, you cannot assign a value to it because it is a constant that already has a value; - same issue with
anotherOperation
- you are using an invalid operator (
=<
). The correct operator for greater than or equal to is>=
, note that the greater sign comes before the equals sign (easy to remember: it's the same order as the name of the operator) - your comparison is not being assigned to a constant (you need to assign the comparison's result to
isGreater
) - you are assigning
true
toisGreater
, not the result of the comparison.
Hope that helps
Cheers
Alex
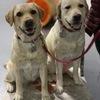
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi AJ Robertson
You can write:
let isGreater: Bool
if anotherOperation >= someOperation {
isGreater = true
} else {
isGreater = false
}
(note that using this approach we have to declare isGreater
before we go into the conditional, as constants and variables declared in a conditional fall out of scope when the conditional ends)
However, that isn't what is in Karar's code. The word if
appears nowhere in the code. So that code assigns true
to isGreater
regardless of the result of the conditional.
As for your question about how else could it be written, remember that anotherOperation >= someOperation
is itself an expression that resolves to true or false. Indeed, it is this fact that makes it possible to use this comparison as the test for the if
statement. Since anotherOperation >= someOperation
will produce true or false, we can just assign that directly to isGreater
:
let isGreater = anotherOperation >= someOperation
in the above line of code, isGreater
will have the value true
if anotherOperation
is greater than or equal to someOperation
, and will have the value of false otherwise.
Hope that's clear
Cheers
Alex