Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial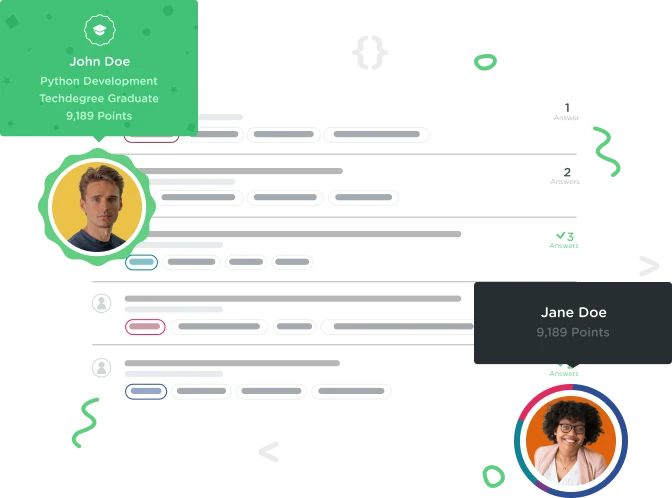
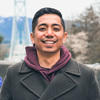
Stheven Cabral
Full Stack JavaScript Techdegree Graduate 29,854 PointsConfused about calling a component method from another component.
In this video, Guil creates the following method in the App component: handleScoreChange = (delta) => { // this.setState( prevState => ({ // score: prevState.score + 1 // })); console.log(delta); }
Then creates a prop in the Counter tag located in the Player component that passes in the handleScoreChange method: const Player = (props) => { return ( <div className="player"> <span className="player-name"> <button className="remove-player" onClick={() => props.removePlayer(props.id)}>✖</button> { props.name } </span>
<Counter
score={props.score}
index={props.index}
changeScore={props.changeScore}
/>
</div>
); }
Then in the Counter component, he calls the changeScore prop using an anonymous function: const Counter = (props) => { return ( <div className="counter"> <button className="counter-action decrement" onClick={() => props.changeScore(-1)}> - </button> <span className="counter-score">{ props.score }</span> <button className="counter-action increment" onClick={() => props.changeScore(1)}> + </button> </div> ); }
My question is, why do we have to use an anonymous function to call the changeScore prop? Why can't we just pass in the changeScore prop like this:
<button className="counter-action decrement" onClick={props.changeScore(-1)}> - </button>
2 Answers

Christopher Dehner
10,708 PointsParentheses are important because they are how JavaScript knows to execute a function. They're almost like a "method" (like .parseInt for strings or .map for arrays) that signals to JS that you want the function to run.
Because of this, there are a couple ways to write a callback function in onClick events (in React specifically):
- call the function directly without parentheses
<button onClick={ myFunction }> Call myFunction </button>
or
- call the function with parentheses inside of a function declaration This creates a reference for the click event instead of executing the function immediately when the JS engine runs.
<button onClick={()=>{myFunction()}}> Call myFunction </button>
Since you have to pass in the (delta) argument with parentheses, #2 is the only method that will work.
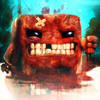
Henrique Hemerly
8,587 PointsThank you, Christopher, that's exactly what I was confused about.
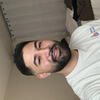
Darrel Valdiviezo
Full Stack JavaScript Techdegree Student 13,473 PointsThanks Christopher
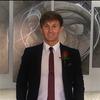
Liam Clarke
19,938 PointsHello
The reason its in an arrow function is so that this
is bound within changeScore
when you pass it as a prop.
Check this out on Reacts site explaining this behaviour
Hope this helps
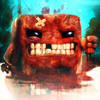
Henrique Hemerly
8,587 PointsThank you for the reply, Liam. However, my issue was not with the binding but with the fact that a callback inside a callback was necessary
Henrique Hemerly
8,587 PointsHenrique Hemerly
8,587 PointsWould like to know this as well...