Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial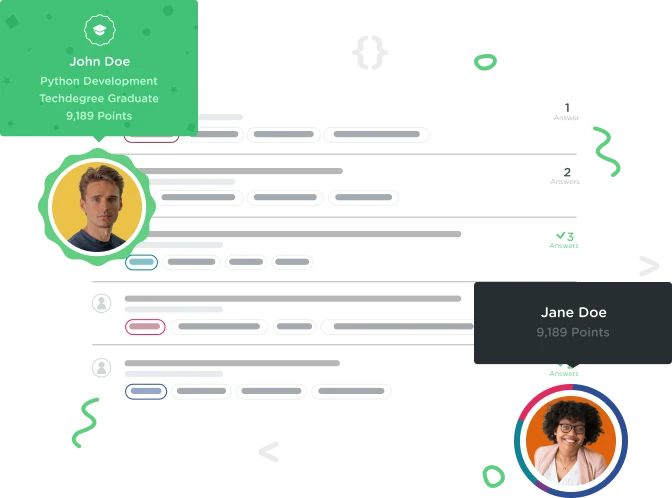
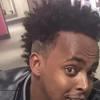
Abdijabar Mohamed
1,462 PointsConfused about how I should use switch and points together
//Where is the error in my code
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(direction: String) {
switch direction{
case "Up": y.Point = y+1
case "Down": y.Point = y-1
case "Left": x.Point = x-1
case "Righ": x.Point = x+1
default: break
}
}
}
THE QUESTION IS:
In the editor you've been provided with two classes - Point to represent a coordinate point and Machine. The machine has a move method that doesn't do anything because most machines are motionless.
Your task is to subclass Machine and create a new class named Robot. In the Robot class, override the move method and provide the following implementation. If you enter the string "Up" the y coordinate of the Robot's location increases by 1. "Down" decreases it by 1. If you enter "Left", the x coordinate of the location property decreases by 1 while "Right" increases it by 1.
Note: If you use a switch statement you can use the break statement in the default clause to exit the current iteration.
5 Answers
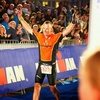
Steve Hunter
57,712 PointsHi there,
You have a location
variable that is of type Point
. A Point
has two properties, x
and y
. To access these properties, you access them from the instance variable, location
. So, you can access the x
position with location.x
and the y
position with location.y
.
You have the correct implementation of the switch statement, i.e. you are adding and taking away from x and y appropriately, but you've not quite accessed them correctly. s above, you need to go through location
to access each, not straight to the Point
struct/class. Use the ++
and --
operators on each position to move the location
.
Your code will look something like:
class Robot: Machine {
override func move(direction: String){
switch direction{
case "Up" : location.y++
case "Down" : location.y--
case "Left" : location.x--
case "Right" : location.x++
default : break
}
}
}
I hope that helps.
Steve.
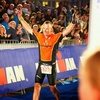
Steve Hunter
57,712 PointsChange the parameter type going into the func - this works:
func enum_move(direction: Direction){ // not an override - different parameter
switch direction{
case .Up : location.y++
case .Down : location.y--
case .Left : location.x--
case .Right : location.x++
}
}
Tested and looks good! :-) If you're using this to pass the challenge, you'll need to rename the method else the tests will fail. I'll have a go to see if I can get it to pass now.
Steve.
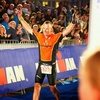
Steve Hunter
57,712 Points[EDIT] - Of course it won't pass the tests in the challenge as that is expecting to send a String "Up" to move the Robot, not an enum value. The enum method needs to be used such as:
let robot = Robot()
robot.move(Direction.Right)
That's not a string so the challenge tests using ...
robot.move("Up")
... will fail.
Steve.
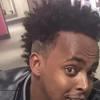
Abdijabar Mohamed
1,462 PointsThanks Steve. I appreciate
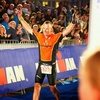
Steve Hunter
57,712 PointsNo problem - I hope it helped.
Steve.
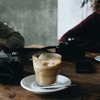
Mitchell Warmerdam
4,225 PointsHi Steve,
Thanks for your answer.
However, I have one question. When I try to link the cases to the Enum "Direction", I get an error. Could you explain why this is not possible?
class Robot: Machine {
override func move(direction: String){
switch direction{
case .Up : location.y++
case .Down : location.y--
case .Left : location.x--
case .Right : location.x++
default: break
}
}
}
Thanks, Mitchell
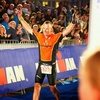
Steve Hunter
57,712 PointsHi Mitchell,
How have you implemented the Enum?
Steve.
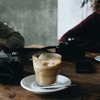
Mitchell Warmerdam
4,225 PointsHi Steve,
The Enum is implemented as followed
enum Direction {
case Left
case Right
case Up
case Down
}
Cheers, Mitchell
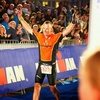
Steve Hunter
57,712 PointsApologies, I'm being slow today - been out running so the brain's a bit dead!
The func
is expecting the user to call it by sending in a String
as a parameter. How will the user call the func using your enum?
I'm just firing up Xcode (after the update has installed) and will have a go at finding a solution for you.
Steve.
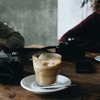
Mitchell Warmerdam
4,225 PointsThanks Steve. I understand that part now. However, I'm still struggling with completing the challenge. In Xcode I get no errors but in the challenge the following code is not approved:
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
enum Direction {
case Left
case Right
case Up
case Down
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
class Robot: Machine {
override func move(direction: String){
switch direction{
case "Up" : location.y++
case "Down" : location.y--
case "Left" : location.x--
case "Right" : location.x++
default : break
}
}
}
Hopefully you can help me with this last question ;)
Thanks, Mitchell
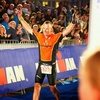
Steve Hunter
57,712 PointsYour code works fine for me here - there may be a temporary glitch in the compiler?
Steve.
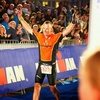
Steve Hunter
57,712 PointsMitchell,
The reason your code won't work is that there are two challenges that are essentially the same. One uses Strings, the other uses Enums.
This question was initially about the string implementation. This challenge.
You are trying to solve this challenge which uses Enums.
That code should look like:
func move(direction: Direction) {
// Enter your code below
switch direction{
case .Up: location.y++
case .Down: location.y--
case .Right: location.x++
case .Left: location.x--
}
}
Apologies for the confuson - I only just saw the other challenge when another student asked about it. I went to link them to this post, but saw that the quiz was slightly different!!
Sorry for the confusion - I hope you go this sorted eventually. Luckily, my solution with the Enums would work, though.
Steve.

Dale Bailey
20,269 PointsThere is currently an error on treehouse where by you need to escape the ' in "Do nothing! I'm a machine!" like so "Do nothing! I\'m a machine!" This is the correct code
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I\'m a machine!")
}
}
// Enter your code below
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up": ++self.location.y
case "Down": --self.location.y
case "Left": --self.location.x
case "Right": ++self.location.x
default: break
}
}
}
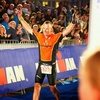
Steve Hunter
57,712 PointsHi Dale,
The escape character will restore the code formatting, ensuring the colours are consistent but the compiler/parser should still pass the correct code without escaping the apostrophe?
Steve.

Dale Bailey
20,269 PointsYeah, Just thought it was worth mentioning as syntax highlighting quickly shows up any other errors users may be having