Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial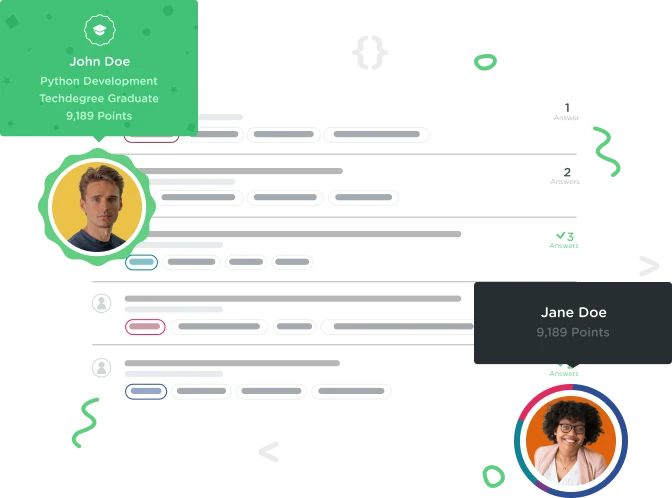

Daniel Scheiter
8,794 PointsConfused about not using input()
I am having trouble doing this challenge. My attempt failed and I don't know what to do now.
add_list = []
def add_to_list(number):
add_list.append(number)
def show_list():
print("the sum of")
for number in add_list:
print(number)
print("is 6")
show_list()
1 Answer
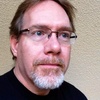
Chris Freeman
Treehouse Moderator 68,423 PointsThe task is asking: Make a function named add_list
that takes a list. The function should then add all of the items in the list together and return the total. Assume the list contains only numbers. You'll probably want to use a for loop. You will not need to use input().
What may not be clear is you do not have to provide an input or function calling mechanism in your code. When you click on the "Check Work" button, a grader will be invoked which both calls your code with test data and also checks the returned values.
For this task you need to return
the sum of the items in the input list. Adding hints to your code:
#add_list = [] #<-- global not needed
def add_list(number_list): #<-- change name to "add_list". Name must match task so grader can properly call it;
# Renamed parameter to indicate list
add_list.append(number_list) # <-- REPLACE this statement with code that adds up the items in number_list
return total #<-- add return statement so the calling code can see the result placed in a variable (called total in this case)
# Not needed:
#def show_list():
# print("the sum of")
# for number in add_list:
# print(number)
# print("is 6")
#show_list()
Add comments if you have further questions! Good Luck!
Daniel Scheiter
8,794 PointsDaniel Scheiter
8,794 PointsI tried to follow what you said but it came up with this error. Bummer! add_list didn't return the right answer. Got 1 when adding [1, 2, 3], expected 6
(I put a pipe to separate the lines) [edit formatting --cf]
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsYour are very close. After looking at your reformatted code, it seems the
return
statement is indented to far and is return after the first past of the loop.Dedent it to close the
for
statement.