Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial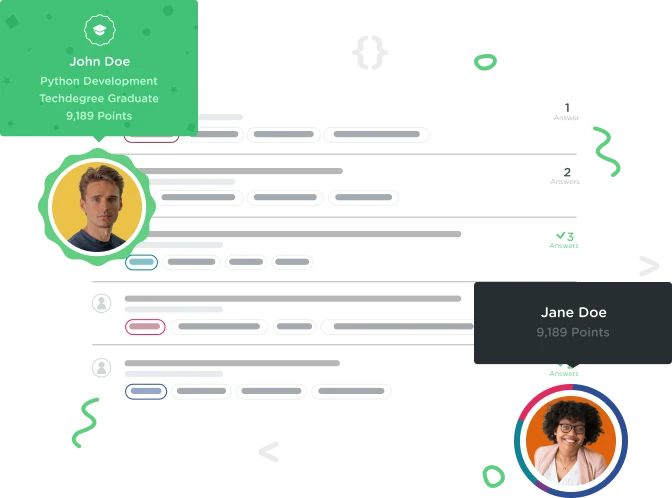
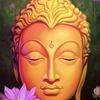
Craig Mayne
Courses Plus Student 1,675 PointsConfused about response in the $.post function, can someone explain?
I'm trying to get my head around what is happening under the hood with the following function:
var url = $(this).attr("action");
var formData = $(this).serialize();
$.post(url, formData, function(response)){
$('#signup').html("<p>Thanks for signing up!</p>")
}
The url and formData doesn't matter, what I'm interested in knowing is how response is processed. In other words, how does the post function retrieve the value in the anonymous function's ()'s to check whether it succeeds or fails.
Can someone write a set of basic functions that would do something similar? That would give me a good idea of what is happening to response.
Thanks
3 Answers

Tayler Kemsley
21,479 PointsHi Craig,
All the jQuery AJAX stuff does make life really damn easy, but it's hard to see what's actually happening behind the scenes.
Have you been working though the AJAX basics course? Because the full breakdown of how AJAX requests work is covered really well in there: https://teamtreehouse.com/library/ajax-basics
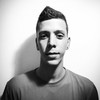
Jesus Mendoza
23,288 Points$.post is an ajax method define inside jQuery which takes 3 params: url, data and a callback function which will be executed after your .post function succesfully ran. The callback function takes 1 param which is is the response (you can call it whatever you want).
Behind the scenes the post method is doing something like this:
- Check the url passed
- Post the data coming from the post method to the url passed
- Get a response from the server
- Store the response data inside the param from the callback function.
- Execute the callback function
That's why you're able to use the data coming from the server, because at some point, the post method stored that data inside the param given by you inside the callback function and then executed that callback funtion. Take in mind that jQuery is just a library made to make JavaScript easier to use, so behind the scenes is using raw JavaScript.
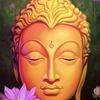
Craig Mayne
Courses Plus Student 1,675 PointsIn this instance is it necessary to pass the response into the callback function seeing as we didn't do anything with it?
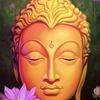
Craig Mayne
Courses Plus Student 1,675 PointsI've worked it out actually, I feel so stupid haha.
response isn't being extracted from the anonymous function, it's being passed into the function lol, it's just not called within the function. For some reason I was thinking it was something happening within the anonymous function that would make the response.
Forget me asking this question, but thanks for your time. :)
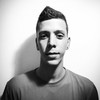
Jesus Mendoza
23,288 PointsYeah your code is a little bit wrong, the function should be called from outside the params of the .post method.
var url = $(this).attr("action");
var formData = $(this).serialize();
$.post(url, formData, function(response) {
$('#signup').html("<p>Thanks for signing up!</p>")
});

Tayler Kemsley
21,479 PointsAh I love that feeling when it clicks in your head!
But yeah, the response (which could be called anything, I usually use just res because typing 5 extra characters is way too demanding) is whatever the server is passing back to you, and the function is what you DO with that response.
So if the response was an object you could get to a value in that object using response.foo in your callback function or if it was an array and you needed the third item you could use response[2].
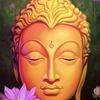
Craig Mayne
Courses Plus Student 1,675 PointsYep, I think the thing that confused me was the fact that response was never used within the function, so it seems unnecessary to pass it into the function anyway. But as it was I thought there must be a reason why behind the scenes and I was trying to figure what it could be. I'm glad it's not as complicated as I thought.
Craig Mayne
Courses Plus Student 1,675 PointsCraig Mayne
Courses Plus Student 1,675 PointsHey Tayler,
Yes I've been going through that course and that's where I came across that function. Callback functions have never been properly explained in treehouse though I have found other resources where I've been able to find out a bit about them, but in this case I was wondering how response in particular is "extracted" if you like, from the anonymous function and tested.
In other words, I don't understand how an argument can be pulled out of an anonymous function when that function is not doing anything with the argument itself. Which is why I was thinking a simple example would help me understand that part at least, and not the actual $.post function itself.