Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial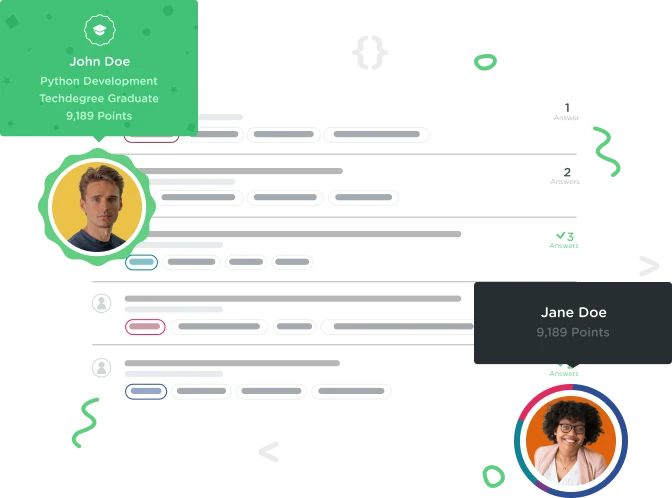

Unsubscribed User
3,436 PointsConfused about the functions
Hello, The following code was used in the example mentioned in the video:
function print(message) {
document.write(message);
}
function printList (list) {
var listHtml = "<ol>";
for (var i=0; i<list.length; i+=1){
listHtml += "<li>" + list[i] + "</li>";
}
listHtml += "</ol>";
print (listHtml);
}
printList (playList);
I'm confused about the function argument "message" and "list". Here are my questions: 1- What are they here? Just a string? 2- Why did we use "document.write (message)" and not "document.write (print)"? 3- Why did we use "list.length" and not "printList.length"? 4- What is "print"? It wasn't declared before using it in "print(listHtml)"
2 Answers
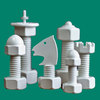
Steven Parker
230,274 PointsThis should answer your questions:
- The names "message" and "list" are function parameters. They act as placeholders in the function definition for the actual variables or values that will be passed to the function when it is called. The names don't matter but it is good practice to call them something that relates to what they will contain or be used for.
- As I said, these names are just placeholders but "message" implies that the actual argument will contain a message of some sort.
- The name "printList" is the function itself, but "list" represents the argument that will be given to it. And it is the length of the argument that is being used in the loop test.
- The name of the first function is "print". It is declared (and defined) in the first 3 lines of code. It has global scope so it can be called from anywhere in the program.

Unsubscribed User
3,436 PointsThank you for the reply.
Can you explain to me what is the outcome of the following code in this video? 'document.write(message);'
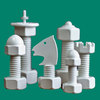
Steven Parker
230,274 PointsThe document.write function causes the provided argument to be shown on the web page. In the video, it is used to display the user's music play list with each song shown in number order.
Unsubscribed User
3,436 PointsUnsubscribed User
3,436 PointsThank you for explaining. I have two more question and will link them to the previous points: 1- Can I call the function by the function name and the parameter? I mean is this wrong: "document.write (print)"? instead of "document.write (message)". 4- Is this right: "message (listHtml)"? instead of "print (listHtml)"
Steven Parker
230,274 PointsSteven Parker
230,274 Pointsdocument.write(print)
" would write the function code itself for the print function into the document, instead of the message.message(listHtml)
" would cause an error.