Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial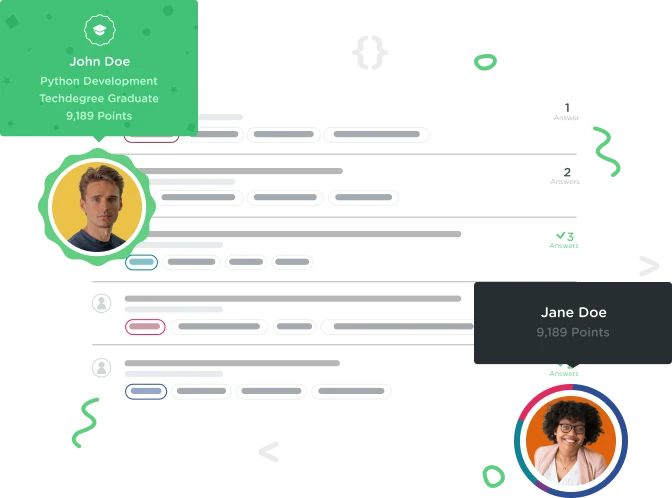
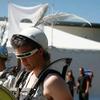
Connor Acott
7,572 PointsConfused about the purpose of Guil's last step.
I understand how the final step works, but I don't understand why it makes the code more efficient and maintainable. I was following when the code looked like this:
let html = '';
const randomValue = () => Math.floor(Math.random() * 256);
function randomRGB() {
const color = `rgb( ${randomValue()}, ${randomValue()}, ${randomValue()} )`;
return color;
}
for ( i = 1; i <= 10; i++ ) {
html += `<div style="background-color: ${randomRGB()}">${i}</div>`;
}
document.querySelector('main').innerHTML = html;
Afterwards, he adds the value
parameter to randomRGB()
, and chooses to pass randomValue()
back into the first function using this new variable:
let html = '';
const randomValue = () => Math.floor(Math.random() * 256);
function randomRGB(value) {
const color = `rgb( ${value()}, ${value()}, ${value()} )`;
return color;
}
for ( i = 1; i <= 10; i++ ) {
html += `<div style="background-color: ${randomRGB(randomValue())}">${i}</div>`;
}
document.querySelector('main').innerHTML = html;
This makes sense, but what purpose does it serve? To my untrained eye, it just looks like roughly the same amount of code, and if anything it seems slightly more complicated after the change.
Thanks!
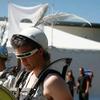
Connor Acott
7,572 PointsOh shoot, I'm sorry! 😅 First time asking a question, and I thought asked it under the video. Thanks for the reply.
https://teamtreehouse.com/library/the-refactor-challenge-duplicate-code
1 Answer

Paul Messmer
15,023 PointsHey no it's completely ok!
So in coding there is not a right or wrong way of doing things as long as you get what you want to work to well work. The DRY method or don't repeat yourself is a good rule of thumb to go by to save you time in coding, make your code easier to understand, and to make your code execute faster.
As far as this video goes I have to agree with you, I think the other way is better and I would have left it as each time you need a value in the RGB to call another randomvalue.
I think what Guil is trying to do in this section is show how code and specifically functions can be used in a number of different ways. So he is passing a function to a function then executing it inside. I don't see a point in this other then to show that it can be done.
Eventually (This is meant to excite you about coding not to stress you out) instead of for loops you can have a function that calls itself a certain number of times then will stop. This method is called recursion. Here is an example
//recursive function
function test (numberOfRounds){
console.log(`this is the recursive function ${numberOfRounds}`)
//this is where you would put the code you wanted to execute
//checks if it should stop the iteration
if (numberOfRounds - 1 > 0){
test(numberOfRounds - 1);
}
}
//calls the test function and says to run it 5 times
test(5)
//does the same thing as the test function
for (let i = 5; i > 0; i--){
console.log(`this is counting down ${i}`)
}
//standard for loop
for (let i = 0; i < 5; i++){
console.log(`this is counting up ${i} `)
}
The way the computer runs the test function is like this test(5) calls test(4) calls test(3) calls test(2) calls test(1) then executes all of them The function is calling itself and then stopping when it hits a certain threshold.
Coding is really neat and there are multiple ways of solving the same issue :)
I hope this helped!!

Paul Messmer
15,023 Pointshttps://paulmessmer.com/randomThemes.html Here is my random color generator if you would like me to explain how I made it I don't mind, I feel like I went a little off topic haha
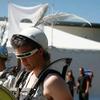
Connor Acott
7,572 PointsOh nice! Okay cool, thanks a lot for that. I could totally see how passing a function to another function could have other uses, so I'm glad to hear it was more of a demonstration and I wasn't missing something important.
Recursion looks awesome! It's kind of trippy that that actually works, putting the function within itself like that... How nice too, to then have a super minimal amount of code to recall the same function later. That is so great, so many solutions to the same problem.

Paul Messmer
15,023 PointsYeah not a problem I am glad it helped you! It is really cool how there are so many solutions to the same problem!
Paul Messmer
15,023 PointsPaul Messmer
15,023 Pointswhat video was this regarding?