Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial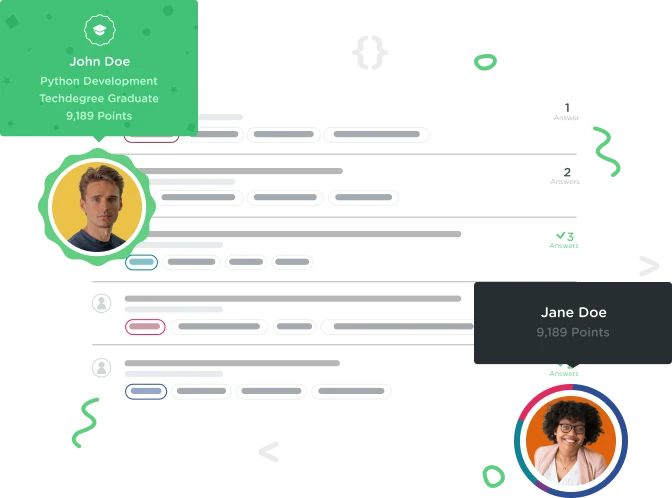
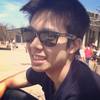
Corey Yao
1,136 PointsConfused about the second if statement he used.
I was confused about what he did the simplify the code. He originally had:
if (correctGuess = true){
document.write ("You guessed the number!");
}else{
document.write ("Sorry you didn't guess right. The number was " + randomNumber);
}
I understood that part. But then he goes to say you can SIMPLIFY the code by taking out the = true part. Giving us:
if(correctGuess){
document.write ("You guessed the number!");
}else{
document.write ("Sorry you didn't guess right. The number was " + randomNumber);
}
I know he explained how this code can work in the video but I was still confused about the explanation. My question is, how does the if else statement know which statement to print out based on the boolean value of correctGuess? Because in the first statement since we told it to print out "You guessed the number!" if it was = true. But in the simplified version, we took out the =true part. So how does the if else statement know to print out "You guessed the number!" when correctGuess is equal to true?
Thanks for all the help in advance!
4 Answers

Philip G
14,600 PointsHi Chungchi,
An IF-Statement always checks if the condition you want to check returns true.
For example:
if(true){
// DO THIS
} else {
// DO THAT
}
Here DO THIS is executed, because the condition is actually true.
When you check a boolean variable that contains true, or if you check a function that returns true, most time you can leave the == true part (to check equality you need two equality signs). Well, I think you can leave it every time :)
When you want to check if a condition is not true, just give a ! on the beginning of the statement:
if(!true){
// DO THIS
} else {
// DO THAT
}
Here DO THAT is executed.
Hope I could help you to understand the JS IF-Statements.
Feel free to ask me again if you don't understand it.
Best regards,
Philip

Gunhoo Yoon
5,027 PointsPhilip Graf already explained nicely on how if statement works but let me add two more things.
First the code example 1 has a bug.
if (correctGuess = true)
If you do something like this there is no point in having else statement. Because your code will always evaluate to true no matter the current state of correctGuess
. Because you are assigning correctGuess to be true when your code reaches the if statement. And I think this is not what you were intending to do since you have else statement.
So if you want to check for equality you need to do something like this.
if (correctGuess == true)
//or
if(correctGuess === true)
You will probably learn the difference between == and ===. Right now what is important to know is = doesn't check for equality.
Second is reason why code example 2 works let me explain step by step.
1 Every if condition has to be true or false. Otherwise it will throw an error.
2 correctGuess variable can be anything since it can be any of these: true, 1, 10, 'correct', 'true', 'false', 'abc' etc...
3 Then how does if statement know which of them are true or false?
4 JavaScript is smart and it will do something called casting which is converting your value to different data type.
5 Problem is JavaScript won't tell you that it's doing all these things. So you need to check it before.
6 For this you just need to follow what JavaScript says what it is. For example...
//These are all correct
0 == false //True
1 == true //True
12345 == true //True
'false' == false //False, what the hell?
and so on....
There are many examples which can confuse you as a beginner but what you need to know is JavaScript sometimes need to change the value to make apple vs orange into apple vs apple.
Now you know this you might notice the potential bug which code sample 2 can produce.
//Let's say your correct guess is number 1
var correctGuess = 1;
//This will pass the true test.
if (correctGuess)
document.write("Correct!")
//However let's say your correct guess is number 0 which is totally possible.
correctGuess = 0;
if (correctGuess)
document.write("Correct!") //This line will never be run.
Do you see the problem? it is possible that correctGuess is actually 0 but then it will never say "Correct!"
Since I'm also a learner I'll be happy if anyone can point out any errors if you see them.

Philip G
14,600 PointsAlso a good answer!

Logan Wood
8,320 PointsI'm just a beginner too, so correct me if I'm wrong. In this particular example, I don't believe 0 will ever be selected because the random number will be between 1 and 6, therefore can't be 0. Also, I was a bit confused about the "simplifying" part of the new "if" statement, but I came to the same realization that they pointed out above... that is that the "if" statement is ALWAYS testing whether it is "true" by definition, so restating // if ( correctGuess === true) //would be redundant. And you know...DRY..."don't repeat yourself".

Gunhoo Yoon
5,027 PointsThere is no sign here which indicates random number is always in a range between 1 and 6.
if ( correctGuess === true)
was there to show what's happening because OP had confusion between assignment and equality operator. I haven't even mentioned a thing about "simplifying"
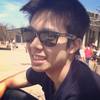
Corey Yao
1,136 PointsThank you both so much for the help! It makes so much more sense now.

Logan Wood
8,320 PointsHere was the original post in which he referenced simplify twice. And in the video, the activity was to have a random number generator that generated a number between 1 and 6, which is why his code had some of the extra lines. Both those points are what I was referring to. Hope that didn't cause any confusion.
"Confused about the second if statement he used.
I was confused about what he did the simplify the code. He originally had: if (correctGuess = true){ document.write ("You guessed the number!"); }else{ document.write ("Sorry you didn't guess right. The number was " + randomNumber); } I understood that part. But then he goes to say you can SIMPLIFY the code by taking out the = true part. Giving us:"

Gunhoo Yoon
5,027 PointsI was only referring to OP's code at the time of writing my post. After watching video, I realized the correctGuess won't cause any problem since it will only hold boolean. I originally thought correctGuess was input from user. So sorry for the confusion there. Other than that I don't really understand what you are trying to say.
nicole grima
5,028 Pointsnicole grima
5,028 Pointsthanks for clearing that up - had same question! :)
Philip G
14,600 PointsPhilip G
14,600 PointsGlad to help! To prevent confusion, I added a note about double equality signs :)
nico dev
20,364 Pointsnico dev
20,364 PointsI know it's been a while, but I wanted to thank you, for this reply was the most crystal-clear I've found for this specific issue that was driving me mad. :)