Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial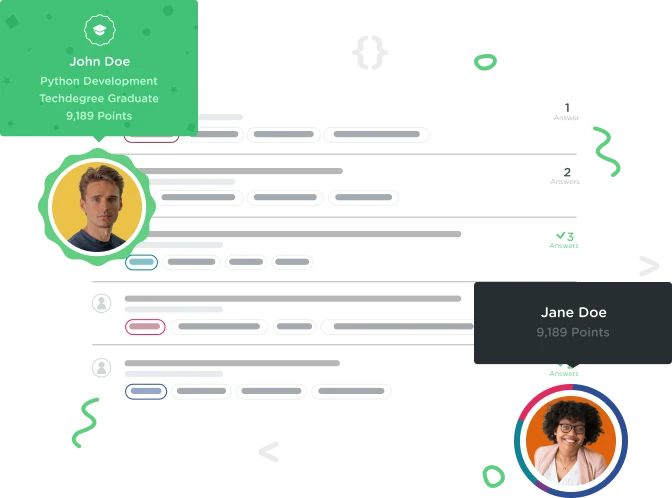
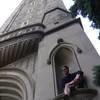
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsConfused about using React with Express
I have some experience with Express, and I have been learning React for the past few weeks and I'm really confused about how developers use these technologies together. It seems to me that React is only good for connecting to an API and wouldn't be appropriate as a the client layer of an Express app. I can't understand how you would use Pug templates with Express or how you would pass data into your views. Is React ever actually used in an Express app or is it more typical to build an Express API and then connect a separate React client app to it? Just need some clarification on how this stack is actually implemented in professional environments. Thanks.
1 Answer
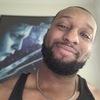
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsHi Michael Cook,
I think in professional environments, you'd see it in a MERN like stack. So MongoDB (or some other database whether it be SQL or NoSQL), Express (which acts as your server on the backend), React (your front-end user-interface), and Node.js (the environment for your app).
In an MVC architecture, React is your view.
That said, React and Pug are not the same. Pug is a template engine. I can't think of a scenario off the top of my head where you'd use React and Pug together. They essentially serve the same purpose, but React offers more power and the ability to add state to your app. With Pug, when a request is made to one of your routes, express would respond by rendering one of your Pug views (and optionally passing in information into that view, so that Pug could dynamically render the proper HTML).
With React, the express server would simply return information to the client, and the client would rely on the React library to create reusable components that a package like Babel would interpret into valid HTML.
Does this answer your question? If not, let me know and I'll try again.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsHey Brandon thanks for your really great response. I guess what I still don't get is how you would inject arguments into your views as you do normally with Pug. I'm also not sure how you would use React Reacter in conjunction with routing on the backend. That sort of thing.
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsBy arguments I’m assuming you mean logic, such as for...in loops and variables, etc. If this is indeed what you mean, React allows you to include expressions that it evaluates. So say you had a library management app. And when your user visited the home page, a request was sent to the server to return a list of all the books in the database. You could use a for...each loop or a map (if the result is sent in the form of an array) to go over each book in the database and insert a BookCard component into the DOM/virtual DOM for every book.
That’s not super unlike anything that you can do with Pug. But say you had a Sign Up form that you created with a Pug. Say you wanted to do some client side validation. For instance, you have a password field and a confirm password field. Before you send this information to the server, you want to verify on the client side that the password and confirm password fields hold the same string, if not you want a message to pop up that informs the user that the values are not the same.
To make something like this work in Pug, you would have to attach a js file to the HTML page rendered for the view, and in that js file, you’d have to create a function that would do this.
With React, the same file that renders the Sign Up form can be the same file in which you create a function that validates the password and confirm password field for your users.
React runs off it’s on server (let’s say localhost:3000 is the base url serving our react-app), so in our example library management app, if we were to travel to localhost:3000/books/3, a GET request would be sent to localhost:3000/books/:id, which would lead to the corresponding component to be rendered. Let’s call the component BookDetail. And when the BookDetail component is rendered to the page, you could have a function inside that sends a request to a separate server or an API (let’s say this other server is located at localhost:5000) for the book information that you will use to render the page correctly.
Not sure if that answer explains things as clearly as I’ve intended. I’m including a link that may help to explain when you might would choose one over the other.
https://www.slant.co/versus/185/10513/~pug-jade_vs_react
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsThanks for your really great answer, it is very detailed. But like I said what I don't understand is how you would inject arguments into the view. Pug makes this easy.
In that simple little example, I can easily inject data from a database call into the pug template. I have no idea how you would do this with React. That's what I mean.
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsYou would not pass in an argument in that way.
With react, it would be done like this...
Then in your react file (the one that sent the request), you would have something like this...
Does that make sense? Pug and React both render what your users see, but they don’t do them in similar ways. Pug is a template engine, React is not. You don’t render React from express. Express simply sends the information back, and you can decide within React what you do with it.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsYou are probably going to start getting pretty frustrated with me, because I still don't get it. I understand the concept of the server sending your data back as JSON. But if the server is sending JSON, then that means it is NOT sending the view file. Which brings me right back to what I was thinking originally, which is that React is good for consuming an API, but would not be appropriate for replacing Pug templates for your view layer. In other words, you can't use React in an Express project, but you can write an API with Express and consume it with React.
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsYou can ask as many questions as you’d like. No frustration on my end.
That said, you are right in that React would be great for consuming APIs. But it’s really just a Javascript library, so you can use it without consuming an API. Just don’t want you to walk away from this believing that it’s only useful for API consumption.
It’s not a template engine so it is not rendered by Express.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsNo I know how useful React is. I've built a few projects with it so far and I love it. I've just had a hard time understanding how you would use it with Express. Before learning JavaScript for the back-end I did some stuff with PHP and I got used to the idea of the LAMP stack. You usually implement an MVC design and your back-end renders your views. But I've struggled to see how that would work with React. You could only write an API that serves JSON and consume it with React. So this is how React is usually used in a professional environment?
Brandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 35,771 PointsIf you're using React and Express together, than yes, you are separating your data storage from your user interface. If you're using React and Express together, then your express app stops being an "express" app, and becomes a Web App. And React acts as the client layer of this Web App, not the client layer of an express app.
So yes, if you're using React and Express together, then Express is acting as your server. It's not rendering any views. It's simply acting as an API that your User Interface–created by React–is consuming. You've got it (and perhaps you've had it all along)!
I would not suggest though that this is how React is only (or usually) used in a professional environment. Certainly if one is working professionally with React, it would be beneficial (potentially even necessary) for one to know how to make http requests and consume APIs. But React's purpose at its core is to build User Interfaces (not consume APIs). So say you got a job with a company that makes Desktop games using Electron.js. Perhaps none of these games require you to make a single request to an API, but the company likes React because of its support and reusable components and its ability to manage state. In this case, you likely would be using React just to create User Interfaces.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsYes I definitely know what you mean. That's part of the beauty of React that it's stack-agnostic and can be used to build just about anything for any platform.