Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial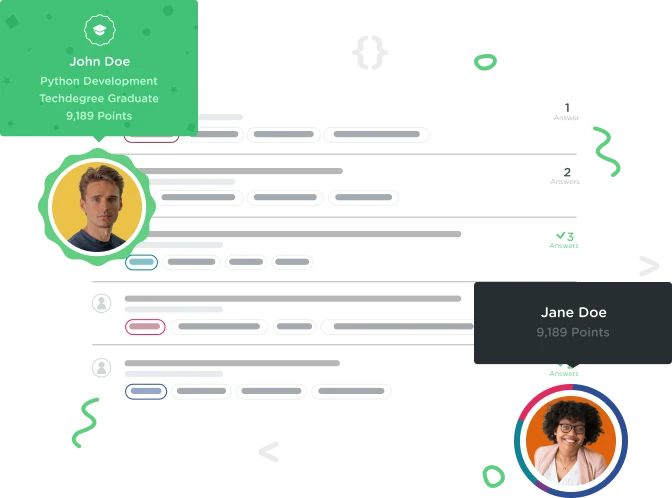

karan Badhwar
Web Development Techdegree Graduate 18,135 PointsConfused about whole Error handling concept
I am kind of confused when what to use and where to use throw or new Error and any reason @Guil used new Error here and why we we just cannot pass the String in promise.reject()?
Secondly, how it bubbles up to which catch method am not able to understand, even in asynchronous programming videos, too he used bubble technique but I am not getting it, any assistance would be much appreciated.

Caleb Kemp
12,754 PointsGlad I was able to help
1 Answer

Caleb Kemp
12,754 PointsWhen you handle an error with the ".catch" method, it will always pass you an error to handle. When you call the ".catch" method, you can then reference this error. This is what happens in the video at 1:10. When you write a "catch" block, it will only run if and only if an error occurs. If we want to manually make the "catch" block run, you can make this happen by calling the "reject" method. Since the "catch" method requires an error, Guil had to create an error in the "reject" method to pass to it. This is not a perfect explanation, but here is a link to documentation on promise.reject and promise.catch methods if that is more helpful.
As for bubbling. The concept of bubbling is fairly simple. in Javascript, an event starts with the "target" (what triggered the event like a click). This then propagates to its parent element, then to that element's parent etc. until there is no longer a parent element to propagate to. Let me give an example of this. Just say you ran some code like this (you can paste this here if you want to see it run)
<!DOCTYPE html>
<html onclick="myFunction3(event)">
<body onclick="myFunction2(event)">
<button onclick="myFunction(event)">Try it</button>
<script>
function myFunction(event) { var x = event.bubbles; alert("hello");}
function myFunction2(event) { var x = event.bubbles; alert("hello2");}
function myFunction3(event) { var x = event.bubbles; alert("hello3");}
</script>
</body>
</html>
If you were to click the try it
button, you can see this propagation at work. First, it starts with the target (try it button), and will print "hello", the event will then proceed up the chain to the "try it button's" parent which is "body". This will trigger the print "hello2". Finally, the event will propagate to the "body's" parent which is "html" and will print "hello3".
Now that we see how bubbling works in general, let me give a more specific (more like the video) use case. Just say, every time I clicked on an element, I wanted to see a message telling me what type of element was being clicked. How would I go about this? Well, I could add an "onclick" method for every possible element on the page OR I could write just one "onclick" in the highest most parent element and use event bubbling to determine what made the click. Here is what that looks like
<!DOCTYPE html>
<html onclick="myFunction(event)">
<body>
<p>Click on any element to see what it is, ps I am a p element</p>
<span>Click me I am a span <br><br></span>
<button>I am a button</button>
<a><br><br>I am a link or "a" tag</a>
<script>
function myFunction(event) {
alert(event.target.tagName);
}
</script>
</body>
</html>
If you ran this, you can see that by putting an event listener in a parent, I can capture the events of all its children. That is all that Guil does in the video. He moved the "catch" method from "fetchData" to its parent. Now that the "catch" is in the parent method, it will automatically capture the events (errors) bubbling from its children. I realize this may not be the best explanation, but I hope it helps.
karan Badhwar
Web Development Techdegree Graduate 18,135 Pointskaran Badhwar
Web Development Techdegree Graduate 18,135 PointsHi Caleb Kemp , it is indeed a very good explanation. Thankyou so much for clearing my doubts out sir