Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial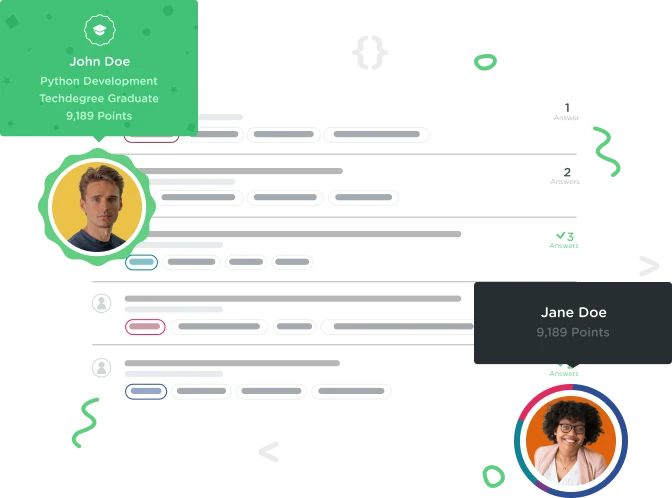
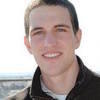
Paul Westbrook
11,340 PointsConfused as to where to put the do/catch statement for this challenge.
When I put the do/catch statement outside of the struct then the compiler doesn't recognize the func parse() but if I move it within the struct where the func parse() is defined then it doesnt recognize "do". I'm just a little confused on what I'm doing wrong.
enum ParserError: ErrorType {
case EmptyDictionary
case InvalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
if data == nil {
throw ParserError.EmptyDictionary
}
if data?.keys.contains("someKey") == false {
throw ParserError.InvalidKey
}
}
}
do {
try parse()
} catch {
print("You blew it")
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
2 Answers
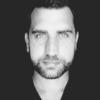
Jhoan Arango
14,575 PointsHello Paul:
You are very close, the only thing you have wrong is the placement of your do.
Use the constant already declared by the challenge parser to call on the parse method.
enum ParserError: ErrorType {
case EmptyDictionary
case InvalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let data = data else {
throw ParserError.EmptyDictionary
}
guard let key = data["someKey"] else {
throw ParserError.InvalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
// You have to place your "do" here, since it's after the declaration of the constant "parser"
do {
try parser.parse() // Use the instance of Parse to call on the instance method parse.
} catch {
print("You blew it")
}
Good luck
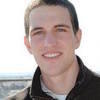
Paul Westbrook
11,340 PointsIt's always the one thing I didn't try :) Thanks for the help! That makes sense to me now.