Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial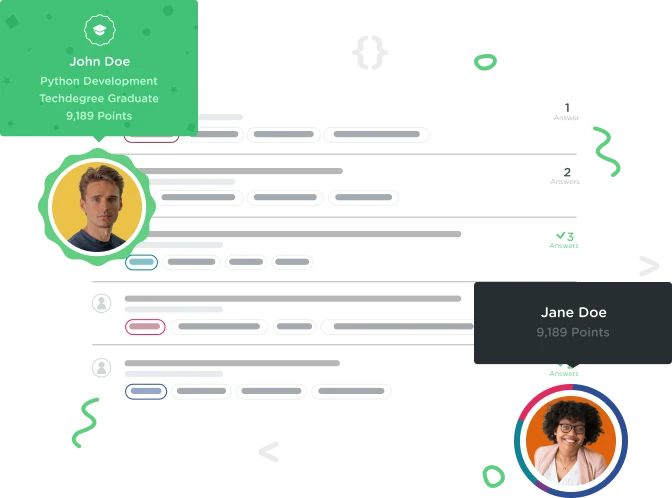

Kshatriiya .
1,464 PointsConfused by Two load methods
Hello in the video there were two different load methods:
public void load() {
load(MAX_PEZ);
}
public void load (int pezAmount) {
mPezCount += pezAmount;
}
1) My first question is, what is the purpose of the first load method then now that second method actually let you input the amount to be loaded? (is it to act as a default function?)
is it to keep this method alive? :
if (dispenser.isEmpty()) {
System.out.println("Loading...");
dispenser.load(); }
2) It is my understanding that second method functions independent of the second load method but not the other way round, please clarify if this is the case.
Thank you very much in advance.
1 Answer
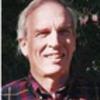
jcorum
71,830 PointsThe second load() method overloads the first. To overload means to write a method with the same name but a different method signature (number and type of parameters).
A really good example of overloading are the 8 println() methods. There is one for ints, one for doubles, one for Strings, etc. The overloaded methods make it possible for you to print ints, doubles, Strings, etc.
In this case one load() method, when called, assigns mPezCount the max pezAmount.
public void load() {
load(MAX_PEZ);
}
If you only have this load method you wouldn't be able to refill a dispenser until it was completely empty.
The other assigns a variable amount: the amount passed in as a parameter:
public void load (int pezAmount) {
mPezCount += pezAmount;
}
This method allows you to top up a dispenser, adding just the amount you want to, or the amount needed to fill the dispenser.
These two load() methods do not act independently. The first calls the second. Before it was modified it was independent:
public void load() {
mPezCount = MAX_PEZ;
}
But now, with the modification, it calls load(int pezAmount) with the max amount.
Why do this? Doing it this way conforms to the DRY principle. You do not want to reproduce in load() any of the code in load(int pezAmount).
There really is no such thing as a default function, unless by "default" you mean a no-parameter function.
Here's an excerpt from the Java API re println. All these methods are in the same class (PrintStream). Each of the 9 after println() overload println():
void println()
Terminates the current line by writing the line separator string.
void println(boolean x)
Prints a boolean and then terminate the line.
void println(char x)
Prints a character and then terminate the line.
void println(char[] x)
Prints an array of characters and then terminate the line.
void println(double x)
Prints a double and then terminate the line.
void println(float x)
Prints a float and then terminate the line.
void println(int x)
Prints an integer and then terminate the line.
void println(long x)
Prints a long and then terminate the line.
void println(Object x)
Prints an Object and then terminate the line.
void println(String x)
Prints a String and then terminate the line.
Alex Garcia
6,374 PointsAlex Garcia
6,374 Points