Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial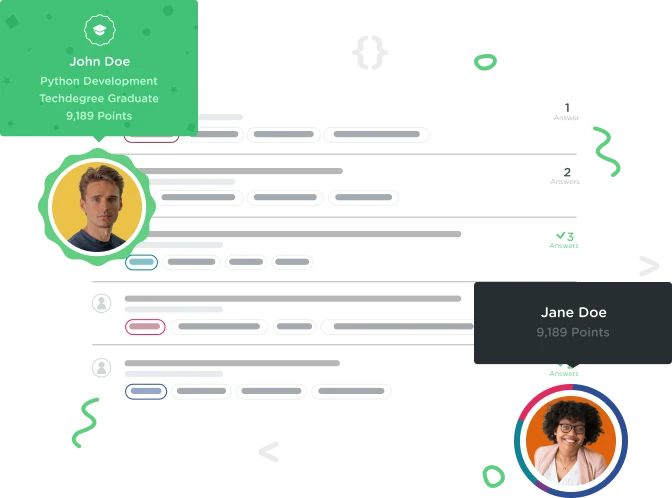

ben127127
1,980 PointsConfused on this "Introducing Dictionaries" Quiz
I do not know how to complete questions 2-3 of this quiz on iterating over dictionaries. Here are the questions. They are both "fill in the blank" only, with the blank coming right after "in student."
- Fill in the blank. Complete the code so it will iterate over only the keys in the student dictionary.
student = {'name': 'Craig', 'major': 'Computer Science', 'credits': 36}
for key in student._():
print(key)
The underscore is the only space I can edit. I would do this by deleting the parens, and just stating for key in student:
. But that's not allowed, so what else can I fill in here?
2. Fill in the blank. Complete the code so it iterates over only the values in the student dictionary.
student = {'name': 'Craig', 'major': 'Computer Science', 'credits': 36}
for val in student._():
print(val)
Same thing, only here I'd want to change it to:
for key, val in student.items()
print(val).
Is it possible to complete this only by filling in the blank? (Which again is the underscore after "in student.") Thanks!
2 Answers

Tai Jun Jie
Full Stack JavaScript Techdegree Graduate 23,907 PointsHey ben, for the challenge, you can use the .keys() method. For more info about it, https://www.w3schools.com/python/ref_dictionary_keys.asp However, your answer is not wrong as well. Keep up the good work.
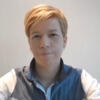
Ave Nurme
20,907 PointsHi Ben
The thing with dictionaries is that they have key-value pairs.
In here...
student = {'name': 'Craig', 'major': 'Computer Science', 'credits': 36}
...the keys are...
name
major
-
credits
and the corresponding values are...
Craig
Computer Science
36
You can iterate over the keys like this:
for key in student.keys():
print(key)
And you can also iterate over the values:
for value in student.values():
print(value)
If you wish to iterate over both keys and values, you need to use the word items()
:
for key, value in student.items():
print(key)
print(value)
You stated that you wished to do this:
for key in student:
This syntax is more for lists, for example:
fruits = ['apples', 'oranges', 'bananas']
for fruit in fruits:
print(fruit)
"""
OUTPUT:
apples
oranges
bananas
"""
If you do this with dictionaries, you only get the keys as output:
fruits = {'apples': 3, 'oranges': 6, 'bananas': 2}
for fruit in fruits:
print(fruit)
"""
OUTPUT:
apples
oranges
bananas
"""
I guess the above is not entirely wrong but for the sake of clarity I would use keys()
here:
for fruit in fruits.keys():
print(fruit)
Does this make any sense?