Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial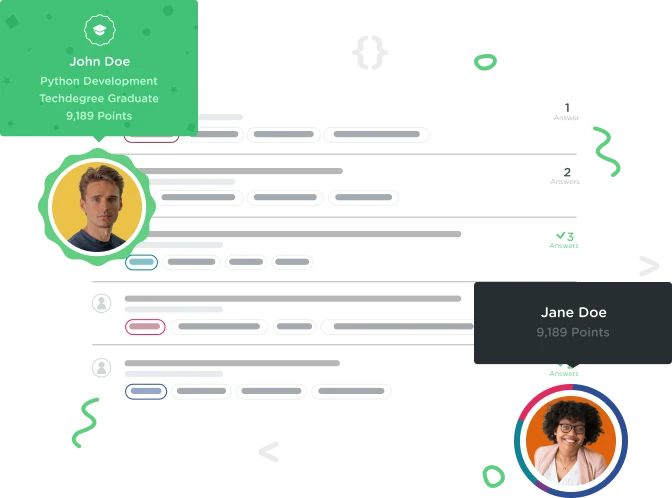

Kostatinos Yacoumakis
Full Stack JavaScript Techdegree Graduate 16,231 PointsConfused on what I'm being asked to do.
I'm having a hard time with this challenge. It's asking me to add a class "highlight " to a p element that is an immediate previous sibling of the button being clicked. Should I be using the insertBefore() method? I've re-watched this sections videos several times and have referenced MDN. Please help me understand this challenge.
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
let classH = document.querySelectorAll('p');
classH.className ="highlight";
classH = classH.previousElementSibling;
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
1 Answer
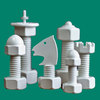
Steven Parker
231,269 PointsIt looks like you have the right idea, but some of the issues here are:
- "classH" is being set to a collection of every paragraph in the document
- there is no "className" propery on a collection (just on individual elements)
- a collection also will not have a "previousElementSibling" property
So some hints:
- use the event target (e.target) as a starting point for DOM traversal
- find the specific paragraph element that follows the clicked button element
- make the class name change on that specific element
Kostatinos Yacoumakis
Full Stack JavaScript Techdegree Graduate 16,231 PointsKostatinos Yacoumakis
Full Stack JavaScript Techdegree Graduate 16,231 PointsYour comments helped but I'm still having trouble. I'm targeting the parent node which is the <p> elements, and adding the highlight class. What am I missing here?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThe paragraph is the sibling of the target. You won't need to reference the parent node in this challenge.