Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial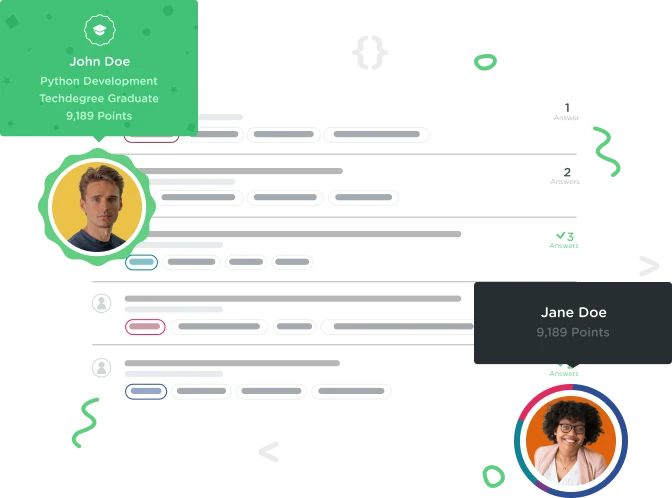

Stivan Radev
1,475 PointsConfused on what the code is doing
OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end.
I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you!
Oh, be sure to look for both uppercase and lowercase vowels!
def disemvowel(word):
word_as_list = list(word)
vowels = list('aeiouAEIOU')
for vowel in vowels:
while vowel in word_as_list:
word_as_list.remove(vowel)
return ''.join(word_as_list)
Can somebody explain to me how this works? I'm a bit confused
3 Answers
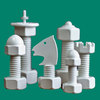
Steven Parker
231,269 PointsI'm not sure your friend is doing you much of a favor by supplying you with "spoiler" answers without explaining them. They might work, but they may also contain unnecessary, redundant, or "bad practice" code that could lead to programming habits that you would do better to avoid. Plus it robs you of the experience of solving the issue yourself, which I think can be an important part of the learning process.
For an example, you are correct in what applying the "list" function to the string of vowels does, but it is entirely unnecessary because a string is iterable, and can be used directly to control a loop.
The rest of the code converts the incoming string into a list so the loop can remove elements from it and then re-assembles it into a string afterwards. But this is just one possible approach to solving the task. You should ask the person who wrote it for their own explanation, and also why they chose this particular strategy.

Stivan Radev
1,475 PointsThank you so much!!

Kayla Johhnson
756 PointsYour issue starts at your For loop. You are comparing the vowels in the vowel list you created when you actually want to be looping through the letters of the 'word' that will be passed through the function. Try: For letter in word or For letter in word_as_list.copy()
Your original code might work with some tweaking but I wouldn't know how - that's out of my league.
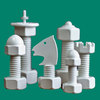
Steven Parker
231,269 PointsThe original code shown above does work with no modifications.

Kayla Johhnson
756 PointsAh, indeed it does. I assumed it didn't since it was posted on here. It is interesting that there are so many different solutions to this problem. I can barely wrap my head around the one I found.

Stivan Radev
1,475 PointsSteven Parker, actually a friend of mine showed me this code. So I'm confused on these parts:
Why is 'word' wrapped in list()
word_as_list = list(word)
is this a short method ...
vowels = list('aeiouAEIOU')
...to this
vowels = ['a','e','i','o','u','A','E','I','O','U']
What is happening here?
while vowel in word_as_list:
word_as_list.remove(vowel)
return ''.join(word_as_list)
Thanks!
Steven Parker
231,269 PointsSteven Parker
231,269 PointsI might help if you first explain why you wrote it that way, and what part of it you are confused about.