Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial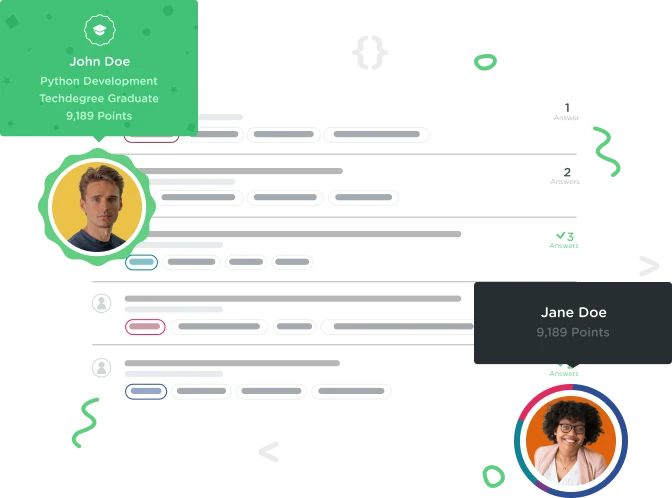

Bryce Hunter
1,186 PointsConfused on what to do
Add a public constructor to the GoKart.java class that allows the parameter color to be passed in. In the constructor store the argument color in the private color field. I'm not sure what I'm doing wrong.
public class GoKart {
private String mColor = "red";
public GoKart (String mColor); {
}
mColor=mColor;
public (String getColor) {
return mColor;
}
}
3 Answers

Luke Snider
2,829 PointsWell first off I'm sure the assignment needs to be within the constructor brackets. so: public GoKart(String color) { mColor = color; }
Also you have already assigned a default value to the private String mColor. But with the constructor method you are initializing a different value for the private String mColor by assigning it whatever is input through the String color from the constructor method.

Taiwo O
2,173 Pointspublic class GoKart {
private String mColor = "red";
public GoKart (String color){
mColor = color;
}
public (String getColor) {
return mColor;
}
}
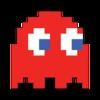
Michael Swoboda
3,543 PointsBasically, your class "GoKart" needs an constructor. If you do not specify one, a default constructor will be defined. The constructor is what is run when creating a new instance of your class (also known as the object) and therefore has to be named like the class itself. The constructor has no additional type declaration as the type is the class itself.
So your constructor may look like that:
public GoKart(String color){
mColor = color;
}
Then you want to expose the color to users of your GoKart-Class - so anyone who creates a new object using your blueprint "GoKart" can retrieve the color. For this there are public functions called "Getters". They just return the value of a specific variable (or value) of the object.
So your getter-function for the color variable of your GoKart-class may look like this:
public String getColor(){
return mColor;
}
Your miss here was that instead of giving your function the return type String and the name getColor, you left the function without a name, but a String parameter needed to run it.
I hope this clears it up somehow.