Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial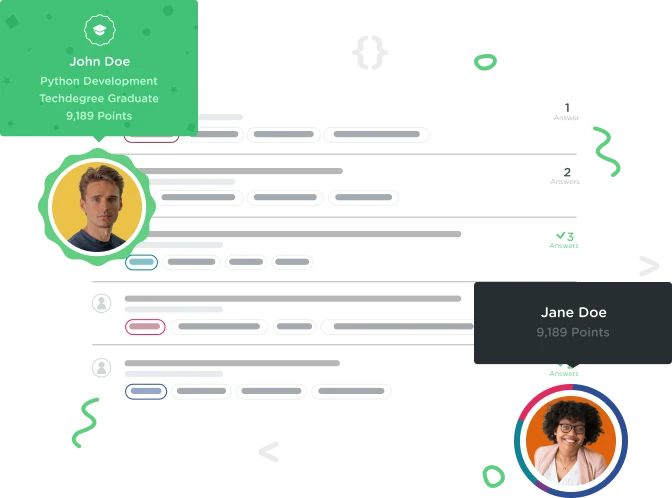

mattcleary2
19,953 PointsConfused with class and initializer
Hey guys,
Sorry to pester you all with yet more questions. I'm having a tough time understanding what to do with the initializer. I don't really know what I'm doing here and just can't get my head around it.
Please help me out?
Thank you
// Enter your code below
class Shape{
var numberOfSides = Int
init (sides: Int){
self.numberOfSides = (1)
}
}
let someShape: numberOfSides(3)
4 Answers

Andrew Taylor
11,500 Points// Enter your code below
So with the init you need to say the self (Shape) numberOfSides variable, will be equal to what the user wants to pass in.
Then when we declare "someShape" we say it is of type Shape and that needs to be passed in a variable (sides) and I gave it a random number 4. Let me know if that helps, this code will work, but it is very important to understand classes fully.
class Shape{
var numberOfSides: Int
init(sides: Int){
self.numberOfSides = sides
}
}
let someShape = Shape(sides: 4)

Reed Carson
8,306 PointsMatt for future reference, if Xcode ever tells you it wants you to put a semicolon somewhere it means it thinks you have 2 different lines of code on the same line. You can do that as long as you separate the different statements with a semicolon.
Like you can do
var shape = Shape(sides: 11) ; var shapeTwo = Shape(sides: 777)
if you wanted to put those statements on the same line.
Basically if its telling you to put a semicolon somewhere, you wrote something wrong lol.
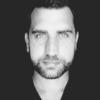
Jhoan Arango
14,575 PointsHello Matt:
In order for you to understand initializers, you need to know what they are for first. This way you will have a more clear on how to use them.
An initializer is the way we give values to a classes's properties in order for us to make instances of it.
class SomeClass {
var myProperty: Int?
}
This is a made up class.. called SomeClass, which has only 1 property. How can we make an instance of it ? How can we access myProperty inside of it ?
In order for us to create an instance of it, we have to have an initializer.
class SomeClass {
var myProperty: Int?
/*
Initializer is a special method, that will give values to the classes's properties
when creating an instance of it. The signature of the init is always the same
init(){ }
*/
init(myProperty: Int?){
self.myProperty = myProperty // This points from the parameter name to the property
}
}
Since we used the name myProperty in both the init's parameter and in the property, then the system may enter in confusion to which one we want to use and for what. So in order for us to make it clear we say self.myProperty pointing to the property of SomeClass. It's as if we had said SomeClass.myProperty, but instead you have to use self.
Now that we have an init in place, we can make an instance of it, and pass it some values.
let anyIntance = SomeClass(myProperty: 1)
// Now we can access the property
anyInstance.myProperty // will print 1
// Or you can change it
anyInstance.myProperty = 2
Hope this helps with your question.
Good luck

Andrew Taylor
11,500 PointsHey Mat,
version of xcode are you running? Mine accepts my code fine :S
mattcleary2
19,953 Pointsmattcleary2
19,953 PointsThank you Andrew!
In xcode however, it is saying that I need a semi-colon after 'Shape"?
Here: let someShape = Shape;(sides: 4)