Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial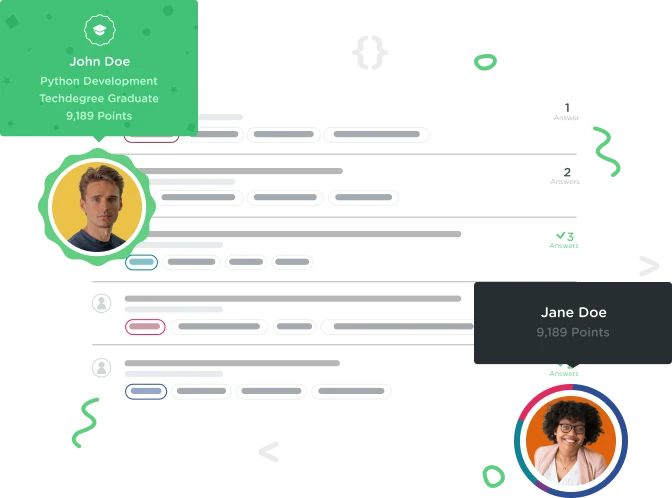

Connor Hamilton
5,023 PointsConfused with Javascript course and interactivity to web pages.
Hello,
Im doing the web page interactivity in Javascript course but im confused on how to make Javascript understand what parts of the HTML is connected to what element.
Ive just got up to when Andrew uses the delete to remove elements from the ul and li but when i am looking at the code i cannot understand how it knows the delete in the HTML is there to delete tasks.
The code/function used is following:
//Delete an existing task
var deleteTask = function() {
console.log("Delete task...");
var listItem = this.parentNode;
var ul = listItem.parentNode;
//Remove the parent list item from the ul
ul.removeChild(listItem);
}
I dont understand where in the above it knows to locate to the delete button in the HTML. Sorry if its extremely obvious but its totally confused me.
3 Answers
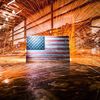
Andrew Shook
31,709 PointsConnor Hamilton, this part of the code is what causes the removal of the html:
//Remove the parent list item from the ul
ul.removeChild(listItem);
Here is the Mozilla Developer's link that explains more about what removeChild() does.

Sophie Rapoport
Courses Plus Student 6,304 PointsI think that what you're struggling with is how to write javascript to select the nodes of the HTML structure that we wish to manipulate. In order to do so, you have to understand the DOM model, and how javascript interacts with it.
The 'this' selector in javascript selects the DOM element that has been acted upon (ie: the delete button).
The problem is that we don't want to simply delete the delete button, we want to delete the task, which contains the delete button, so we have to traverse the DOM a little bit.
In order to select the task that contains the delete button Andrew wrote the following line:
var listItem = this.parentNode;
But he doesn't just want to delete the task, he wants to remove it from the list structure. In order to do this, he has to traverse one more level in the DOM tree:
var ul = listItem.parentNode;
Once he has selected the ul element which is the parent node for the task, he can tell this element to remove the selected child:
ul.removeChild(listItem);
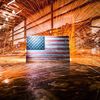
Andrew Shook
31,709 PointsEdited your post to fix formatting of code blocks.

Sophie Rapoport
Courses Plus Student 6,304 PointsThanks Andrew - I'm trying to follow the Markdown cheatsheet instructions so it will work its code coloring magic, but it does not appear to work. Just to make sure it's clear to me, what exactly did you edit?
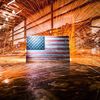
Andrew Shook
31,709 Pointsyou used a single quote instead of a back tick. The back tick key can be found above the tab key on most keyboard and it also has the ~, tilda symbol, on it.

Sophie Rapoport
Courses Plus Student 6,304 Pointsfacepalm. All fixed. Thanks.

Connor Hamilton
5,023 PointsI understand how it removes the HTML, however its in the HTML form itself how does it know the word "delete" is the activator to remove the task?
In this function where does it state that when i clicked the word "delete" it will remove it? That's whats confusing me, theres no find elementbyclass etc to say where this function actually connects to.
I am probably over thinking it but its totally stumped me.
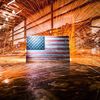
Andrew Shook
31,709 PointsThe click event isn't handle by the function that you posted earlier. My guess is that this is the function executed by the onclick event listener. Look around line 125 for this statement:
deleteButton.onclick = deleteTask;
this is what adds the onclick event to the delete buttons.