Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial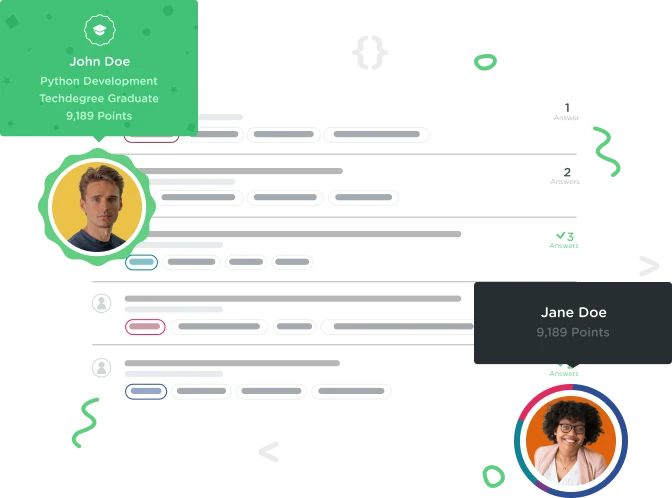

ishakahmed
11,359 PointsConfused with the terms model and table.
Hi, at 6:11 in this video Kenneth referred to Student as a model. Now, I know that the Student class is a model (model - A code object that represents a database table). But in the code (line 40) isn't Student being used to refer to the table, as the table's name is Student.
2 Answers
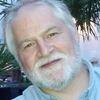
Jeff Muday
Treehouse Moderator 28,726 PointsThe word "model" derives from a specific ORM (Object Relational Mapping) in Python. The model is an "abstraction" of the underlying data representation-- in this case a database table. The Python object/class Student
maps to an actual database table named student
in a relational database through the use of the PeeWee ORM.
Kenneth could have easily taught the use of a different ORM like SQLAlchemy, but the result is the same. A model is an abstraction of an actual table in a database.
There are a number of common ORM system modules you can use in Python. In Flask, you often see PeeWee or SQLAlchemy. In Django you use Django's own ORM. In Web2Py and Py4Web frameworks they use PyDAL (Python Data Abstraction Layer). I sometimes use PyMongo and TinyMongo which works to translate models into the Mongo NoSQL database.
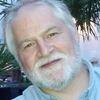
Jeff Muday
Treehouse Moderator 28,726 PointsIt definitely is something worth considering-- the idea of a code object. In this case there are two "meta" level things to consider:
instantiated objects and methods of the Student class, normal for OOP.
"class and static methods" of the Student class itself. This is a way to invoke behaviors of the class by itself that can be consider quasi constructors of objects or lists of objects containing existing data in the database table.
To instantiate a student object you invoke a CLASS METHOD of Student named create()
. This static method is a constructor for a new student object.
current_student = Student.create(lastname='Mathers', firstname='Marshall', dob=date(1980, 5, 5))
This object will have data--
current_student.firstname
current_student.lastname
current_student.dob
This instantiated object will have only a few methods that operate on the current object's data. There are other methods too, but we'll skip consideration of those. The most basic are these:
current_student.save()
saves the current object to the class Meta database and associated table.
current_student.delete_instance()
deletes the current object from the database.
The class itself has methods too. We already saw the class method create()
And there are other important class methods for fetching and querying data that are derived from the PeeWee Model parent class. These are Student.get()
which fetches (and instantiates) a matching student object if the criterion is correct. Then there is Student.select()
which implements a query of the data. This query returns a LIST of objects that match the selection criteria.
I hope this helps your understanding.
ishakahmed
11,359 Pointsishakahmed
11,359 PointsThank you very much for your answer. One thing that confused me whilst reading the definition of model was "A code object". I didn't understand what a code object was, even though I understand OOP. So I just assumed it was referring to the class. Is this assumption correct? In your answer to my previous question you referred to the class Student as an object.