Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial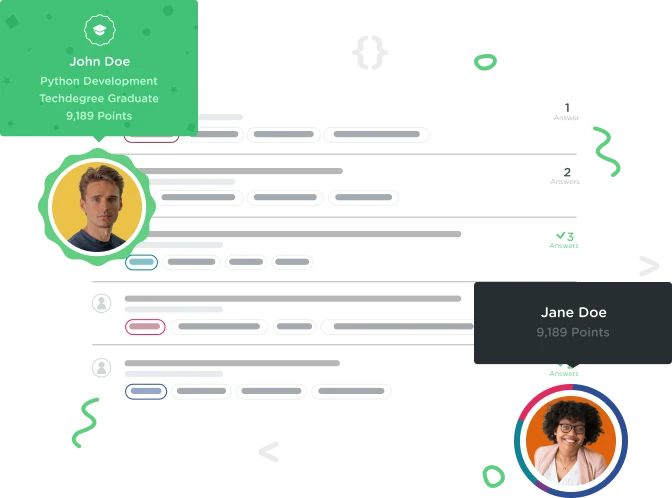
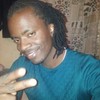
Adlight Sibanda
5,701 Pointsconfused/lost on statement type
Finally, add the statement to execute the creation of your new table!
public class AutoSQLiteHelper extends SQLiteOpenHelper {
public static final String COLUMN_COMPANY_NAME = "COMPANY_NAME";
public static final String COLUMN_CAR_NAME = "CAR_NAME";
public static final String TABLE;
public static final String CREATE_CAR_MAKERS =
"CREATE TABLE CAR_MAKERS (_id INTEGER PRIMARY KEY AUTOINCREMENT, " + COLUMN_COMPANY_NAME + " TEXT)";
public static final String CREATE_CAR_MODELS =
"CREATE TABLE CAR_MODELS (_id INTEGER PRIMARY KEY AUTOINCREMENT, " + COLUMN_CAR_NAME + " TEXT)";
@Override
public void onCreate(SQLiteDatabase database) {
database.execSQL(CREATE_CAR_MAKERS);
database.execSQL(CREATE_CAR_MODELS);
database.execSQL(CREATE_TABLE);
}
}
1 Answer
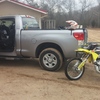
Ryan Ruscett
23,309 PointsYou will want to have a version in there as well for the future. You may want to add an onUpgrade() method at some point.
So I will just explain this and hopefully it helps if you are actually asking a question and not just giving out answers for free.
I do it like this
public class CreateDBHelper extends SQLiteOpenHelper {
// I want to keep track of these.
private static final String DATABASE_NAME = "skateBoard.db";
private static final int DATABASE_VERSION = 1;
//DB to create
private static final String CREATE_TABLE_SKATEBOARD = "create table skateboard (_id integer primary key autoincrement,"
+ "boardChosen text not null, ";
//Then a call to the super to create the DB and the Version so I can do my onUpgrade method later when the db changes or
//features are added
public CreateDBHelper (Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION); }
//Then in the on create, I create the database.
public void onCreate(SQLiteDatabase database) {
database.execSQL(CREATE_TABLE_SKATEBOARD); }