Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial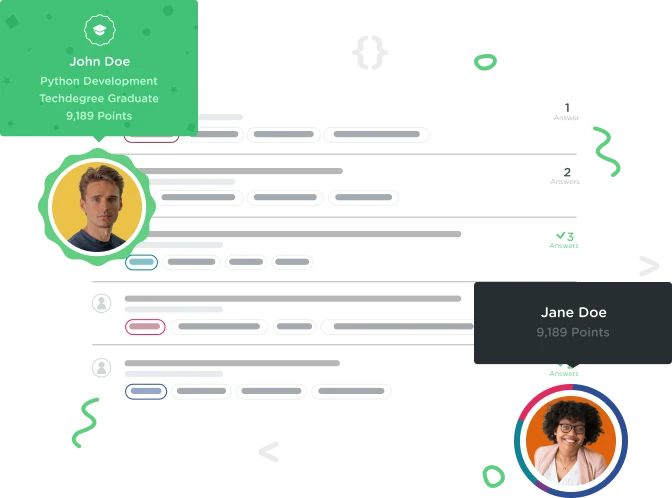
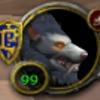
Sergei Miroshnikov
3,313 PointsConfusing error message , function is working as requested .
I have also added print (type (x)) to verify that that x is integer , however I am getting an error Bummer! slice indices must be integers or None or have an index method"
# The first half of the string, rounded with round(), should be lowercased.
# The second half should be uppercased.
# E.g. "Treehouse" should come back as "treeHOUSE"
def sillycase(justastring = 'Treehouse'):
x = len(justastring) / 2
print (type(x))
return (justastring[:x].lower() + justastring[x :].upper())
3 Answers
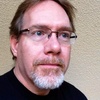
Chris Freeman
Treehouse Moderator 68,457 PointsYour code is very close. The issue is when there is an odd number of characters, your slice variable x
is a float. Correct this by using the round()
function to get the nearest integer:
x = round(len(justastring) / 2)
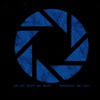
James N
17,864 Pointsi don't think you can put variables in slices. put the len method directly in there.
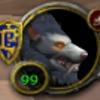
Sergei Miroshnikov
3,313 PointsThank you ! I will change my project interpreter to python 3 , I was suspecting compatibility issue , now thanks to you I know how to fix this , thank you very much !
Sergei Miroshnikov
3,313 PointsSergei Miroshnikov
3,313 Pointsactually when I use round (x) i get the following error from python interpreter "TypeError: slice indices must be integers or None or have an index method" and its type changes to float . I am python 2.7.10. I don't mind casting to int , but it is pointless, hence its integer from the start
x = int(round(len(justastring) / 2))
anyway for some reason the line I just typed above worked , would love to hear explanation why .
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsThe challenges run in Python 3, but I see your error when running Python 2:
To bulletproof the code for both Python 2 and 3 add an extra cast to int