Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial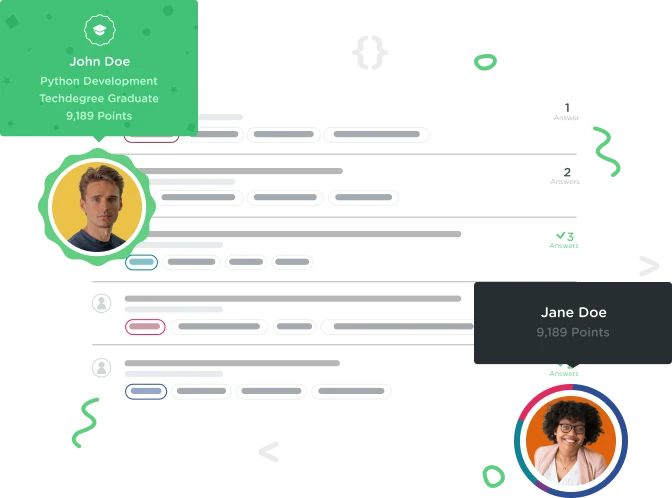
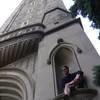
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsConfusion about use of business logic inside controller classes
I know that in MVC the model layer or business logic is supposed to be separate from the rest of the application, and the controllers are just supposed to handle requests and responses and output different data to the views depending on user input. However, it is necessary to use models inside controllers, so I don't know I am supposed to do that without hard coding things. Let me use an example. Suppose I have a LoginController class:
class LoginController
{
//handle control of the login page view
}
And an Authenticator class actings as the model that connects to the database and handles the business logic:
class Authenticator
{
private $db;
public function __construct(PDO $db) {
$this->db = $db;
}
public function login($username, $pass)
{
$user = User::getUser($username, $pass);
$_SESSION['user'] = $user['id'];
}
}
At some point I am going to need to instantiate the Authenticator class inside the LoginController and call the login() method. But this would be hard-coding encapsulated business logic into the controller. Is this even a good practice? What is the right way of including or calling the model and its methods inside the controller?