Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial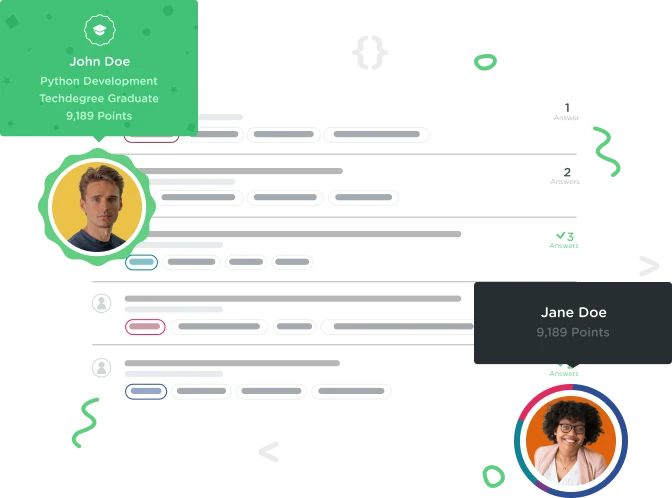

shu Chan
2,951 PointsConfusion with type casting and other things
I am so confused I don't know if I understand or I don't understand. Can someone walk me through my understanding and tell me what I am confused about.
1) In this craig casts other as an Treet object:
public int compareTo(Object obj){
Treet other = (Treet) obj;
This is to required because we are comparing values that will be passed onto the compareTo interface. But Where does (object obj) Come from? Where is it passed from?
Also Craig says every object comes from another Object (which is called object) is that right? So like this origin object is the source of all other objects?
2)
if (equals(other)) {
return 0;
}
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
if (dateCmp ==0) {
return mDescription.compareTo(other.mDescription);
}
return dateCmp;
}
This part here compares the two treets which are passed on from Example,java
Treet[] treets = {secondTreet, treet};
-If the two treets are equal to each other than it will return 0 and nothing will happen (because they are the same treet. BUT WHAT IS BEING COMPARED? date? description? horoscope signs?)
- if the date is the same (which should be impossible given that there is a second counter in the date) than the interface will compare the description to see which one should go first and get a 1 -If one is older than the other than the older one will return a 1
- this value of one is than sorted by this:
Arrays.sort(treets);
3)
Why did we do this?
for (Treet exampleTreet : treets) {
System.out.println(exampleTreet);
why not just
System.out.println(treets);
4)
@Override
This signature tells java that this must be overwritten or the code won't run, is that correct? How does the program know to pass treets into these methods? Is it because of this signature?
I apologize for so many questions, I assure your I watched and rewatched the video at least 6 times...
6 Answers
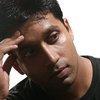
Soumasish Goswami
3,290 PointsIt's been a while that I've watched these videos, so I'll answer your questions more generically. I'm sure someone else can provide you a more context specific answer. First you need to understand the concept of comparator in Java. So say you've a list of numbers, [2, 6, 7, 1, 3, 5], now you want to sort it. That's easy right, the answer list looks something like [1, 2, 3, 5, 6, 7]. So what was the sorting logic here - yes the natural order of real numbers. You could also have reverse sorted the numbers to something like - [7, 6, 5, 3, 2, 1] In both cases the natural order of numbers is used to sort. You can do the same with any primitive data types - int, float, char etc. However things get a bit hairy when you've to compare two objects. Say you have the following Student objects
class Student{
private name;
private age;
}
Now if you need to sort a list of students, do you sort it by the first letter of the name, or the age. Well Java expects you to specify that. By specifying a comparator you're essentially specifying the order of two objects. Thus to specify that students objects should be compared based on their names you can write up something like this,
public class NameComparator implements Comparator {
@Override
public int compare(Student o1, Student o2) {
String name1 = o1.getName();
String name2 = o2.getName();
// ascending order (descending order would be: name2.compareTo(name1))
return name1.compareTo(name2);
}
}
Now passing this comparator to a sort function will enable you to sort the students on the basis of their names. Hope this clarifies - 1 & 2 to some extent. If you wish more on the subject feel free to look up comparators on stack overflow.
And yes every Java object inherits from the Object class. That's a language construct and you don't really need to understand the why, but well that's pretty much how it is.
Finally -3 `for (Treet exampleTreet : treets) { System.out.println(exampleTreet); }' treets is a collection to print all the items you need to iterate the for-loop.
System.out.println(treets);
just prints the address of the collection object which in this case is an ArrayList and not the items contained in the collection.
Hope this helps.
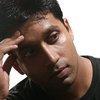
Soumasish Goswami
3,290 PointsEvery class in Java has a concept of this
which is sometimes explicit and at other times implicit. This can often trip new programmers. In essence when you write.
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
It actually means,
int dateCmp = this.mCreationDate.compareTo(othet.mCreationDate);
this
here is the first object.
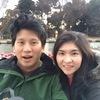
sudhichai ungsuthornrungsi
7,634 PointsThis give me clear picture of the puzzle I have same wondering with shu

shu Chan
2,951 PointsThank you @Soumasish your answer helped me understand a bit more of this.
I'm still confused about how java passes objects into this statement without entering values into any parameters
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
How does it know which is the first object and which is the second? what if there are more than 2 objects in the array of the object it's pulling from?

shu Chan
2,951 PointsAh ok so does that mean when there is an array of objects, this code will choose the first object (set as this.)and compare it to the other objects (set as other) from the array one by one until it reaches the last object in the array?
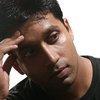
Soumasish Goswami
3,290 PointsYup exactly.

shu Chan
2,951 PointsThanks!
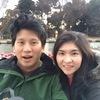
sudhichai ungsuthornrungsi
7,634 Pointsif (equals(other)) { return 0; } int dateCmp = mCreationDate.compareTo(other.mCreationDate); if (dateCmp ==0) { return mDescription.compareTo(other.mDescription); } return dateCmp; }
In the recursive when function call itself how come the -1 come from since it can only return i did not see any execution flow that indicate that there are possible to return as -1
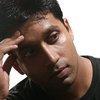
Soumasish Goswami
3,290 PointsThere is no recursion here, it's plain comparison of two objects based on a common property. The convention is if the properties are equal for both objects, return 0, if other is bigger return 1 and if this is bigger return -1.
Darth R3id4k
Courses Plus Student 10,125 PointsDarth R3id4k
Courses Plus Student 10,125 PointsOn the beginning Craig said that this compareTo method is usually incorporated with generics but we all are "blind" about them in this moment of learning path so he used "already well known option" Object to present this issue.
public int compareTo(Object obj) {
hmm.. to compare 2 treets we need they signature (type, name). There is no problem with generics <Treet T>. But here we must must use general option well known for us (for that moment). Treet is taken from array and passed to compareTo method as a general (Object obj) object.
Treet other = (Treet) obj;
In this line general object is cast to Treet object - is explicitly available and can be compare with other Treet object. I suppose the use of generics will exclude this line of code, because the object type and its name will be given immediately on the tray, but this is just my assumption.
@Override helps programmer to read code and helps n compilation process. If parent object change its method signature you will get that information if u try compile this file. Without this annotation compilation process might finish succesfully but the outcome of action such method will be unpredictable.