Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial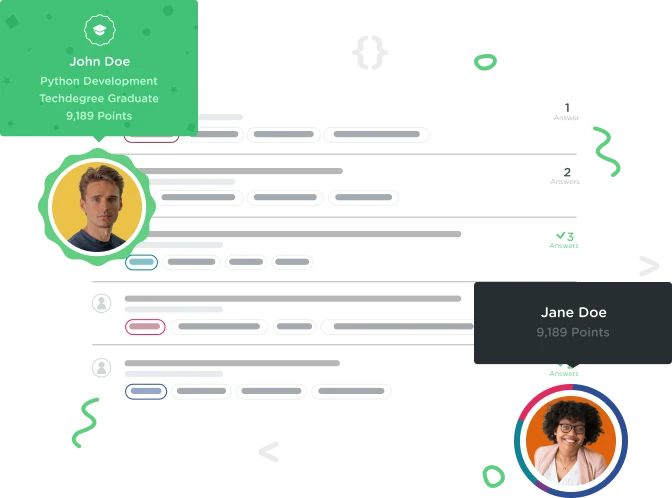
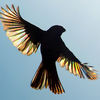
Alex Goldberg
Courses Plus Student 2,408 PointsConsistent error at the end of the Script the Score Counter lesson
At the end of the Script the Score Counter lesson, when I hit the play button the game runs, however when the user moves the frog to collect the flies the score remains the number 0 and the console will consistently throw the following error.
NullReferenceException: Object reference not set to an instance of an object ScoreCounter.Update () (at Assets/Scripts/ScoreCounter.cs:18)
Please let me know if you have any suggestions as to what is wrong in my code. I've rewatched the lesson a few times and am not sure what I'm missing. Thanks!
Below is my ScoreCounter.cs script:
using UnityEngine;
using System.Collections;
using UnityEngine.UI;
public class ScoreCounter : MonoBehaviour {
public static int score;
private Text scoreCounterText;
// Use this for initialization
void Start () {
score = 0;
scoreCounterText = GetComponent<Text> ();
}
// Update is called once per frame
void Update () {
scoreCounterText.text = score + " Flies";
}
}
8 Answers
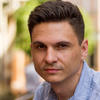
Nick Pettit
Treehouse TeacherHi Alex,
This NullReferenceException error means that when the script is running, it gets to line 18, and the scoreCounterText variable isn't set to anything - it's null. So therefore, calling scoreCounterText.text also won't do anything.
Why might that be? To figure that out, we need to retrace our steps. If scoreCounterText is null, it means it's probably never getting set to anything. So where does scoreCounterText get set?
At the top of the script, we create the variable scoreCounterText and we're saying is a Text object. So that much is given. However, the value of the variable actually gets set in the Start() method, where scoreCounterText is set to GetComponent<Text>() - in other words, the script is looking for a component of type Text on the same game object to which the script itself is attached. The game object Score should have such a component, so if it's not finding it, then it means either the Text component isn't there or the script is attached to the wrong game object.
Alright! So I see that from the help of others, you successfully attached the script to the Score game object and the score counter started working in the game. So then, why might it still be throwing that error?
It's still throwing that error for the same reason - it's attached to a game object somewhere that doesn't have a Text component, so it looks for that text component, it never gets set, and then when it tries to update a non-existent Text component it throws an error.
So how do you fix it? You need to find the game object in your scene that has this script attached where it shouldn't be. And how'd that happen in the first place? You probably clicked the "Add Component" button and originally created the script on the wrong game object. You need to have the Score game object selected when you create the script. At 0:10 of the video, that's why I say "on the Score game object" :)
I hope that helps!
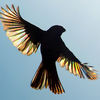
Alex Goldberg
Courses Plus Student 2,408 PointsThanks Nick, I appreciate you responding. Total beginner mistake on my behalf. Great job on the course I'm really enjoying it. Keep up the great work:)
-A
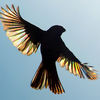
Alex Goldberg
Courses Plus Student 2,408 PointsWe're good here people! I read all the comments when I got home from work and then went for a nice 5K jog and really grasped the concept of how I added the component to the wrong object. I came home just now and quickly discovered that it was added to the Canvas object and not where it should have been which was the Score child of that object. I can start the game, collect the flies, have the score added properly and not throw any exceptions. :)
I work in technology professionally (obviously not as a developer!) and it truly is a team effort. I actually work on an agile team as a Product Analyst overseeing a vendor relationship for an enterprise iOS based mobile app. Anyways, I'm reiterating bothxp's team effort point, from professional experience. Again, I greatly appreciate both of you jumping in and helping me out! I'm very new to both coding and Unity and have a funny feeling I will be posting more questions down the road, but I learned a lot from this mistake, which is the important part.
Thanks again for your help!
Cheers, Alex
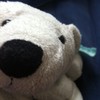
bothxp
16,510 PointsYay!
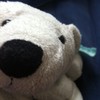
bothxp
16,510 PointsHi,
Can you confirm that you have attached the script to the 'Score' GameObject? (I know that might sound a bit silly as the script is being triggered, but I thought it was worth starting by making sure that the script is attached to the right gameobject to be able to access the Text component.)
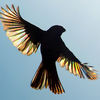
Alex Goldberg
Courses Plus Student 2,408 PointsI feel that this might sound even sillier, but how would I go about confirming that the script was attached?
(Ignore my comment below where I first tried to pose this question, Thanks)
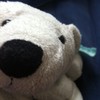
bothxp
16,510 PointsI'll have a look at that section of the course tonight and see if I can replicate the error message that you are getting.
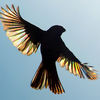
Alex Goldberg
Courses Plus Student 2,408 PointsI know (or feel) that this might sound even sillier, but how would I go about confirming that the script was attached?

John Shoffner
1,514 PointsHi Alex,
The error is because the scoreCounterText is null; when you try to use the property, you're going to see this exception. Under the "Canvas" Game object, please confirm that there is the "Game State Text" game object; if this game object is missing, then GetComponent<Text>() would return null.
- HTH
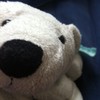
bothxp
16,510 PointsFrom the error message is sounds as if scoreCounterText.text is not being correctly set in the scoreCounterText = GetComponent<Text>(); line.
That line should work as long as the script is attached to the 'Score' gameObject that has the 'Text' component. Which is why I'd earlier asked if the script was correctly attached.
To check this find your 'Hierarchy' tab and look for the Canvas element. If you have followed the steps in the video you should have a Text element under Canvas that you will have have named Score.
The 'Score' gameObject should be displaying '0 Flies' in the top right of the game screen.
Now for your script to work it needs to have been added to this 'Score' gameObject. So select the 'Score' object in the Hierarchy and then the details of all of its components should appear in the 'Inspector' tab. I'd expect it to have a: Rect Transform Canvas Renderer Text (Script) Score Counter (Script)
Now for it to work your ScoreCounter script needs to be attached to this 'Score' text gameObject, if it's not it won't be in the list. So look down the list of components currently displaying in the inspector tab and make sure that you can see 'Score Counter (Script)' listed, probably directly below Text (Script). Look again at the very start of https://teamtreehouse.com/library/how-to-make-a-video-game/score-enemies-and-game-state/script-the-score-counter If it is not appearing as a component of the 'Score' gameobject then you can click the 'Add Component' - Scripts and then select your ScoreCounter file.
Then try running the game again.
I can't see anything wrong in the code that you have posted and the fact that you are getting the error I think means that the script is running, so I assume that it must be attached to something.
If this hasn't help (& I'm not sure it's going to :-/ ) and no one is able to focus in on the exact issue then I think we might have to ask Nick Pettit for his troubleshooting advice.
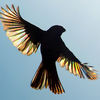
Alex Goldberg
Courses Plus Student 2,408 PointsThanks John Shoffner and bothxp! I really appreciate your help.
Okay, I added the "Score Counter (Script)" component to the Score game object. When I hit play again I now have my score numerically incrementing upon each fly that the user navigates the frog to collect. However in the console I'm still throwing the same error. This seems weird that it would be working in the game (frog collects fly = score increases appropriately) and then still throw the same NullReferenceException error pasted below
NullReferenceException: Object reference not set to an instance of an object ScoreCounter.Update () (at Assets/Scripts/ScoreCounter.cs:18)
Why is the error still being throw with the game seeming to work?
Again thanks for the help that both of you have provided. At this point anything to get that error to go away is much appreciated.
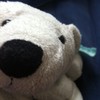
bothxp
16,510 PointsRight, so now you just need to track down the gameObject that you mistakenly added the script to in the first place. To do that just select the gameObjects in the 'Hierarchy' tab one at a time and have a look through the list of components that is listed for each in the 'Inspector' tab. You're looking for a 'Score Counter (Script)' component. If you find one attached to anything other that your 'Score' gameObject then remove it.
My guess is that you might have added it to the Canvas gameObject.
Let us know how you get on!

John Shoffner
1,514 PointsHi Alex,
Glad to hear you're finally able to keep score! ;-) Now that your score is incrementing, it suggest that the scoreCounterText object is being set; otherwise, I don't see how you'd even see the score increment on the screen. But, we need to verify that. Here's what I would do: 1) I would focus on the exception; code-comment out the scoreCounterText in both the Start() method and the Update() method. Run the game and see if you still see the exception error. 1a) If the exception is still thrown, then the scoreCounterText isn't the direct problem. There's another class object that is not being instantiated. 1b) If the exception is not thrown, then it does suggests the scoreCounterText is the culprit, suggesting you may have duplicate script files. Try to trace how the score is being updated. I would comment out the scoreCounterText in the Update() method and hard-code the text value (for scoreCounterText) in the Start() method. Run the game and see if the onscreen text updates accordingly. 2) Put a debug point on scoreCounterText in the Start() method. Verify that scoreCounterText is not null.
- HTH
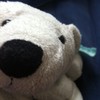
bothxp
16,510 PointsHi John,
We have identified that the error is due to the ScoreCounter script originally being added as a component to the wrong gameObject. The error is triggered because that incorrect gameObject does not have a Text component, hence the null reference. So we have asked Alex to check all of the gameObjects and remove the script from the gameObject that it was incorrectly added to.

John Shoffner
1,514 Pointsbothxp. There's no reason to get nasty about this. Until the problem is resolved, the idea is to pass along any helpful information. Troubleshooting is an art, not an exact science. There have been many times I thought I knew the issue and went down a rabbit-hole, only realize I missed the obvious.
Although it does sound like a duplicate script, which was already suggested, I prefer to pass on additional information which may/may not help Alex.
Hi Alex: the bottom-line is I hope all of the suggestions here help you resolve this issue.
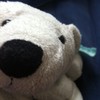
bothxp
16,510 PointsHi John, Sorry if that last comment came across badly, it certainly wasn't supposed to. I was just trying to fit in a few replies in a few spare minutes at work and was just trying to update you on where we'd got to.
I fully agree about the issues in troubleshooting this sort of issue. With something like the Unity client the issue could be in some many settings and tick boxes let alone in the scripts themselves. As Nick had highlighted the most likely cause my feeling was that we should give Alex some time to check it out before we move on to other possibilities. But we do all come at these things differently and it is at the end of the day a team effort.
And yes, I've certainly disappeared down a few unfortunate rabbit holes in the past :-/

John Shoffner
1,514 PointsNo worries... I have to say that I am interested in what happened. I can so see myself misplacing a script file! ;-)
Alex Goldberg
Courses Plus Student 2,408 PointsAlex Goldberg
Courses Plus Student 2,408 PointsAnd btw this is line 18 in that script:
scoreCounterText.text = score + " Flies";