Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial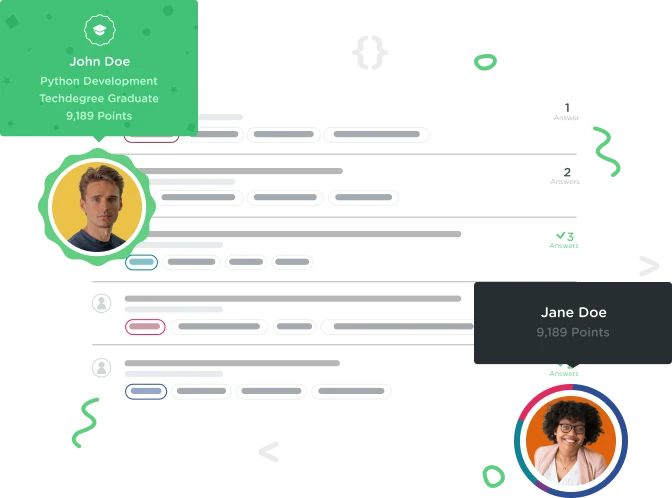

Kamran Khan
837 PointsConsole?
Why do I have to declare Console console = System.console(); in the beginning of the Java Basics program? Is console just the name or does it have a function? Why is it better to use console.printf rather than System.out.println?
3 Answers

Marcus Eley
16,213 PointsHello Kamran,
To answer your first question, yes, console is just a name for the new object that you are creating. So, for instance, you could change that line to Console redConsole = new System.console();
It is important to note that when you do this you will then have to call your methods on the object using the new name like this :
redConsole.printf("This is a formatted line");
Not a big deal right now, but it will be useful in the future for you.
As for your second question, the reason that they use printf instead of println is because printf keeps the formatting that you enter in between your quotation marks. So, you could type something like this console.printf("This is the first line.\nThis is the second line");
It would display in the console like this:
This is the first line.
This is the second line.
I hope this helps
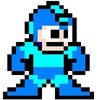
Robert Richey
Courses Plus Student 16,352 PointsHi Kamran,
It's a matter of convenience. There's less typing for console.printf()
than System.out.printf()
.
Regarding your second question, printf
is only better if you need or want to use string formatting, whereas println
cannot perform string formatting. Also, println
auto inserts an end-of-line character for you, but printf
does not.
String greeting = "Hello";
System.out.println(greeting + " world!"); // creates a new line automatically at end of string
System.out.printf("%s world!\n", greeting); // interpolate greeting inside the output string. manually add new line at end
Hope this helps,
Cheers

Kamran Khan
837 PointsIs System.out.printf the same thing as console.printf?
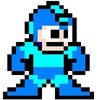
Robert Richey
Courses Plus Student 16,352 PointsKamran,
I'll stop short of saying they are identical, because it is possible there may be minor differences. Otherwise, yes, they function the same.
It is worth mentioning that the Console
class does not provide a method for println
, as we might hope. So, a console object is not a complete replacement for System.out
.
References:
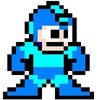
Robert Richey
Courses Plus Student 16,352 PointsHere is an example showing three different ways of printing a formatted string to console output.
import java.io.Console;
public class Food {
public static void main(String[] args) {
Console console = System.console();
String fruit = "apple";
String cheese = "cheddar";
String vegetable = "carrot";
console.printf("%s\n", fruit);
console.format("%s\n", cheese);
System.out.printf("%s\n", vegetable);
}
}

Kamran Khan
837 PointsOkay so I understand what console.printf and System.out.printf mean, but could you clarify what console.format means?
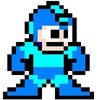
Robert Richey
Courses Plus Student 16,352 Pointsconsole.format is the same as the other two. That's all I was trying to show is that Oracle is giving us several different ways of doing the same thing.
From the link above Oracle - Console class
printf is[sic] A convenience method to write a formatted string to this console's output stream using the specified format string and arguments. An invocation of this method of the form con.printf(format, args) behaves in exactly the same way as the invocation of con.format(format, args)
console.format == console.printf == System.out.printf