Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial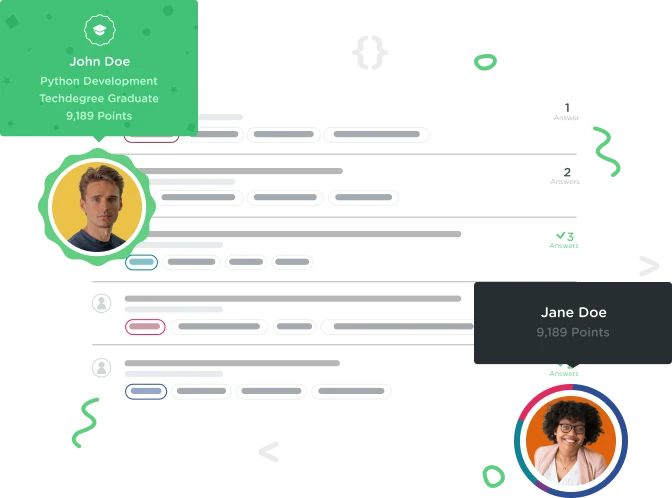
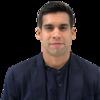
Johnny Garces
Courses Plus Student 8,551 PointsConsole error: "Cannot read property 'appendChild' of null"
I noticed in this video that the instructor did not use DOM manipulation inside of the callback function.
I tried to access some items in the DOM and create the unordered list wrapper and list-items that would populate the ul list wrapper.
The console logged widget.js:12 Uncaught TypeError: Cannot read property 'appendChild' of null.
However, when I did the same thing without use of AJAX (and without requesting from a server), I saw a populated list items inside of my unordered list wrapper. https://jsfiddle.net/jgarces09/17c17596/2/
Any ideas as to why DOM manipulation does not work with AJAX callbacks?
Here's my code: HTML (the only thing that matters is the div with id of 'employeeList'
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX Office Status Widget</title>
<link href='//fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<script src="js/widget.js"></script>
</head>
<body>
<div class="grid-container centered">
<div class="grid-100">
<div class="contained">
<div class="grid-100">
<div class="heading">
<h1>Corporate Intranet</h1>
</div>
</div>
<section class="grid-70 main">
<h2>Lorem Ipsum Headline</h2>
<p>Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo. Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem. Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi consequatur? Quis autem vel eum iure reprehenderit qui in ea voluptate velit esse quam nihil molestiae consequatur, vel illum qui dolorem eum fugiat quo voluptas nulla pariatur?</p>
<p>Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo. Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem. Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi consequatur? Quis autem vel eum iure reprehenderit qui in ea voluptate velit esse quam nihil molestiae consequatur, vel illum qui dolorem eum fugiat quo voluptas nulla pariatur?</p>
<p>Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo. Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem. Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi consequatur? Quis autem vel eum iure reprehenderit qui in ea voluptate velit esse quam nihil molestiae consequatur, vel illum qui dolorem eum fugiat quo voluptas nulla pariatur?</p>
</section>
<aside class="grid-30 list">
<h2>Employee Office Status</h2>
<div id="employeeList">
</div>
</aside>
<footer class="grid-100">
<p>Copyright, The Intranet Corporation</p>
</footer>
</div>
</div>
</div>
</body>
</html>
and my JS
var ajax_object = new XMLHttpRequest();
var employeeContainer = document.getElementById('employeeList');
ajax_object.onreadystatechange = function(){
if(ajax_object.readyState === 4 && ajax_object.status === 200){
var employeeListParse = JSON.parse(ajax_object.responseText);
var employeeListWrapper = document.createElement('ul');
employeeListWrapper.setAttribute('id', 'employeeListWrapper');
var employeeListName = document.createElement('li');
employeeListName.appendChild(document.createTextNode(employeeListParse[0].name));
employeeListWrapper.appendChild(employeeListName);
employeeContainer.appendChild(employeeListWrapper);
}
}
ajax_object.open('GET', 'data/employees.json', true);
ajax_object.send();
2 Answers
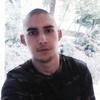
Farid Wilhelm Zimmermann
16,753 PointsHey mate,
I had a similar problem and could fix it by declaring the variable that holds the reference to the div with the id employeeList not so early.
Try moving it into the end of your callback, it worked for me.
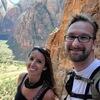
Jeremy Castanza
12,081 PointsI haven't tested this... But just going through the logic of your code, I'm going to go on a limb and say that the answer lies in your error message. On Line 11, you have the following:
employeeListWrapper.appendChild(employeeListName);
In order to use Line 12, you need the information from Line 11 to be STORED as a variable. Otherwise, I'm pretty sure that the AppendChild method just prints to the screen.
For example - maybe something like this:
var employeeCombinedULLI = employeeListWrapper.appendChild(employeeListName);
employeeContainer.appendChild(employeeCombinedULLI);
Hope that helps! If not, let me know. I'm curious if I'm right or wrong on this one.