Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial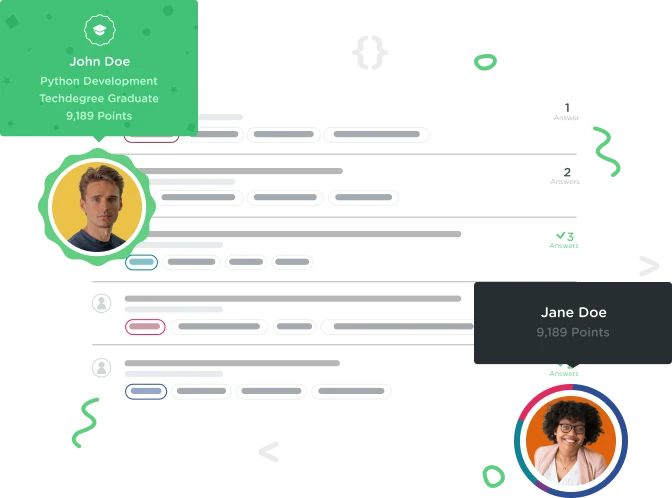

Jakub Szczepanik
10,194 PointsConsole error dataService.getTodos
Hey, so this is how my code looks like
angular.module("todoListApp", [])
.controller('mainCtrl', function($scope, dataService) {
$scope.helloConsole = dataService.helloConsole;
$scope.learningNgChange = function() {
console.log("An input changed!");
};
$scope.todos = dataService.getTodos();
})
.service('dataService', function($http){
this.helloConsole = function(){
console.log('Hello');
};
this.getTodos = $http.get('mock/todos.json')
.then(function(response){
console.log(response.data);
return response.data;
})
});
and the html
<!doctype html>
<html lang="en">
<head>
<title></title>
<link href='https://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link href='styles/main.css' rel='stylesheet' type="text/css">
</head>
<body ng-app="todoListApp">
<h1> My TODOs</h1>
<div ng-controller="mainCtrl" class="list">
<div class="item" ng-class="{'editing-item' : editing, 'edited': todo.edited}" ng-repeat="todo in todos">
<input ng-model="todo.completed" type="checkbox"/>
<label ng-hide="editing">
{{todo.name}}
</label>
<input ng-change="todo.edited = true" ng-blur="editing = false" ng-show="editing" ng-model="todo.name" class="editing-label" type="text"/>
<div class="actions" >
<a href="" ng-click="editing = !editing">Edit</a>
<a href="" ng-click="helloConsole()">Save</a>
<a href="" class="delete">Delete</a>
</div>
</div>
</div>
<script src="vendor/angular.js" type="text/javascript"></script>
<script src="scripts/app.js" type="text/javascript"></script>
</body>
</html>
And the problem is that the console shows error
angular.js:12416 TypeError: dataService.getTodos is not a function at new <anonymous> (app.js:7) at Object.invoke (angular.js:4473) at extend.instance (angular.js:9093) at nodeLinkFn (angular.js:8205) at compositeLinkFn (angular.js:7637) at compositeLinkFn (angular.js:7641) at publicLinkFn (angular.js:7512) at angular.js:1660 at Scope.$eval (angular.js:15916) at Scope.$apply (angular.js:16016) and
angular.js:12416 SyntaxError: Unexpected token ' in JSON at position 8 at Object.parse (native) at fromJson (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:1250:14) at defaultHttpResponseTransform (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:9371:16) at http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:9462:12 at forEach (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:336:20) at transformData (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:9461:3) at transformResponse (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:10241:23) at processQueue (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:14634:28) at http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:14650:27 at Scope.$eval (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:15916:28)
4 Answers

Thomas Nilsen
14,957 Pointsyou're getting: dataService.getTodos is not a function, which is correct, because it's not.
this.getTodos = $http.get('mock/todos.json')
.then(function(response){
console.log(response.data);
return response.data;
})
you need to do something like this:
this.getTodos = function() {
return $http.get('mock/todos.json')
.then(function(response){
console.log(response.data);
return response.data;
})
}
and in your controller do this:
dataService.getTodos().then(function(data) {
$scope.todos = data;
});

Jakub Szczepanik
10,194 PointsStill getting error :/ angular.js:12416 SyntaxError: Unexpected token ' in JSON at position 8 at Object.parse (native) at fromJson (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:1250:14) at defaultHttpResponseTransform (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:9371:16) at http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:9462:12 at forEach (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:336:20) at transformData (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:9461:3) at transformResponse (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:10241:23) at processQueue (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:14634:28) at http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:14650:27 at Scope.$eval (http://port-80-z11x4z210b.treehouse-app.com/vendor/angular.js:15916:28)
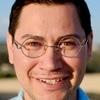
William Ruiz
10,027 PointsI had the same issue. On you app.js code for $scope.todos = dataService.getTodos;
I just removed () and it works without error now. Idk how much of a perfect solution this is but it got rid of my error message :D

Bryn Price
Full Stack JavaScript Techdegree Student 7,253 PointsI have noticed that you have to keep a close eye on what changes between screens, because there will be slight adjustments to the code he doesn't mention, perhaps ones that previously caused an error. Like going from $scope.todos = dataService.getTodos(); before he shows the page and then after it shows $scope.todos = dataService.getTodos;

Thomas Nilsen
14,957 PointsBased on the error alone I'd say you either have the wrong path to the JSON file, or the JSON data is not valid (i.e syntax-errors)
Are you able to share the entire project somewhere? I'll find the error easier that way.
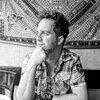
jlampstack
23,932 PointsGoing back to William Ruiz reply, removing the () will solve the problem. Not sure why the instructor shows it as dataService.getTodos(); since getTodos is not a function ???