Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial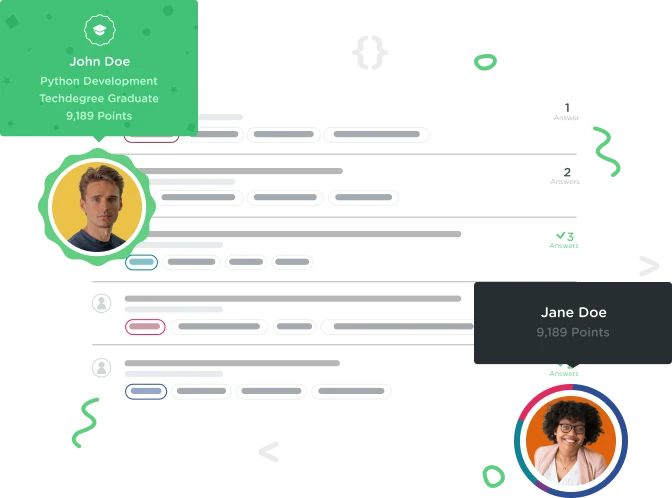
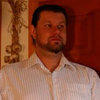
Eric Welch
20,754 Pointsconsole error: ReferenceError: taskListItem is not defined
Basically I'm asking if I missed something in the instructions. I did a search of my workspace code and only returned taskListItem in this function:
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list item events");
// select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
...}
So taskListItem is a variable that should have been declared globally? Am I missing something?
1 Answer
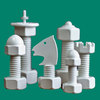
Steven Parker
231,269 Points
Your last loop is using the wrong limit.
Take a look at the last loop of the program:
for (var i = 0; i < incompleteTasksHolder.children.length; i++) {
// bind events to list item's children (task incomplete)
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
Notice that the loop is passing the children of completedTasksHolder to bindTaskEvents one at a time, but the loop itself is using the number of children of incompleteTasksHolder as it's limit. So unless both lists have exactly the same number of children, this loop will run too few or too many (where some will be "not defined") times.
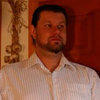
Eric Welch
20,754 PointsThanks, straightened out that loop and things work
Eric Welch
20,754 PointsEric Welch
20,754 Pointsmy entire app.js file: