Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial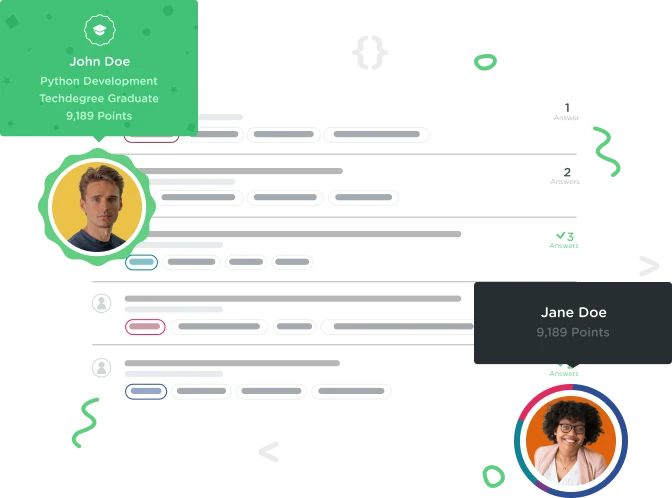
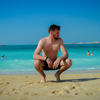
Przemyslaw Chmiel
13,532 PointsConsole Errors. Cannot read property 'length' of undefined
This is a console errors for quiz.. All helps are welcome..
quiz_ui.js:16 Uncaught TypeError: Cannot read property 'length' of undefined
at Object.displayChoices (quiz_ui.js:16)
at Object.displayNext (quiz_ui.js:7)
at app.js:9
var QuizUI = {
displayNext: function() {
if(quiz.hasEnded()){
this.displayScore();
} else {
this.displayQuestion();
this.displayChoices();
this.displayProgress();
}
},
displayQuestion: function() {
this.populateIdWithHTML("question", quiz.getCurrentQuestion().text);
},
displayChoices: function() {
var choices = quiz.getCurrentQuestion().choices;
for(var i = 0; i < choices.length; i++){
this.populateIdWithHTML("choice" + i, choices[i]);
this.guessHandler("quess" + i, choices[i]);
}
},
displayScore: function() {
var gameOverHTML = "<h1>Game Over</h1>";
gameOverHTML += "<h2> Your score is: " + quiz.score + "</h2>";
this.populateIdWithHTML("quiz", gameOverHTML);
},
populateIdWithHTML: function(id, text){
var element = document.getElementById(id);
element.innerHTML = text;
},
guessHandler: function(id, guess) {
var button= document.getElementById(id);
button.onClick = function() {
quiz.guess(guess);
QuizUI.displayNext();
}
},
displayProgress: function() {
var currentQuestionNumber = quiz.currentQuestionIndex + 1;
this.populateIdWithHTML("progress", "Question" + currentQuestionNumber + " of " +
quiz.question.length);
}
};
var questions = [
new Question("Who was the first President of the United States" , ["George Washington", "Thomas Jefferson" ], "George Washington" ),
new Question("What is the answer to the Ultimate Question of Life, the Universe, and Everything?" , ["Pi", "T42" ], "42" )
];
//Create Quiz
var quiz = new Quiz(questions);
//Display Quiz
QuizUI.displayNext();
2 Answers
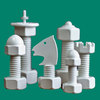
Steven Parker
231,275 PointsIt looks like the issue may be in the "getCurrentQuestion" method, but it is not shown here.
A better way to share the entire project at once is to make a snapshot of your workspace and post the link to it here.
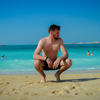
Przemyslaw Chmiel
13,532 Pointsfunction Quiz(questions) {
this.score = 0;
this.questions = questions;
this.currentQuestionIndex = 0;
}
Quiz.prototype.quess = function(answer) {
if(this.getCurrentQuestion().isCorrectAnswer(answer)) {
this.score++;
}
this.currentQuestionIndex++;
};
Quiz.prototype.getCurrentQuestion = function() {
return this.questions[this.currentQuestionIndex];
};
Quiz.prototype.hasEnded = function() {
return this.currentQuestionsIndex >= this.questions.length;
};
function Question(text, choises, answer) {
this.text = text;
this.choises = choises;
this.answer = answer;
}
Question.prototype.isCorrectAnswer = function (choice){
return this.answer === choice;
};
Im did check all of them possible mistakes but i didn't found nothing..
Andrew Basore
Courses Plus Student 6,196 PointsAndrew Basore
Courses Plus Student 6,196 Pointslooks like quiz.getCurrentQuestion() is returning undefined. I dont see the function definition in this code snippet, but that's where you should be looking. Make some logs to check the value of that function and once it starts returning valid information the error should be resolved.