Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial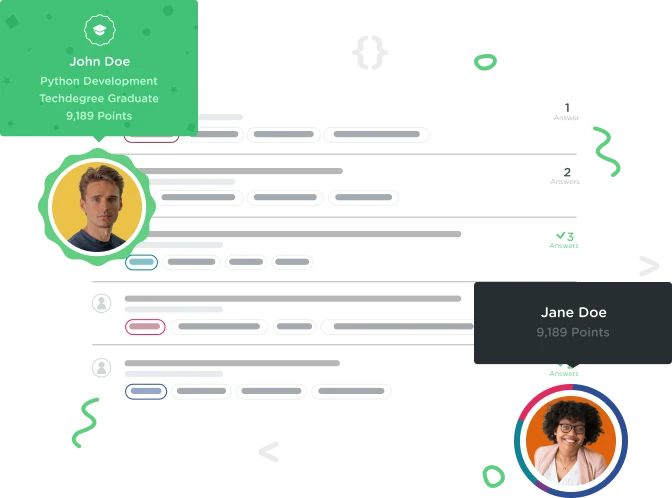

Dale Bailey
20,269 PointsConsole I/O
This code does not work, it throws an error cannot convert from 'string' to 'System.IO.Stream'
on this line
using (var reader = new StreamReader(file.FullName)) {
3 Answers
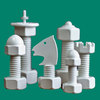
Steven Parker
241,807 PointsThis may require more complete code to analyze.
At first look, this line seems fine. What's even more puzzling is that the constructor for StreamReader is overloaded, and it can take a Stream or a string as an argument, so it should not be trying to convert one to the other.
Even though the error occurs on this line, the actual cause might be elsewhere in the code. Can you post a link to your workspace snapshot or github repo for a more detailed analysis?
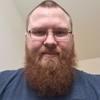
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsI'm was having the same problem, but I made it work by using the work-around provided by Steven (in VS2017 community):
static void Main(string[] args)
{
string currentDirectory = Directory.GetCurrentDirectory();
DirectoryInfo directoryInfo = new DirectoryInfo(currentDirectory);
string fileName = Path.Combine(directoryInfo.FullName, "data.txt");
FileInfo file = new FileInfo(fileName);
if (file.Exists)
{
using (FileStream fs = new FileStream(file.FullName, FileMode.Open))
{
using (StreamReader sr = new StreamReader(fs))
{
}
}
}
}

Samuel Joseph
5,406 PointsHave you tried installing the latest version via Nuget Package Manager?
Dale Bailey
20,269 PointsDale Bailey
20,269 PointsHi, sure.
I just had a look in the StreamReader class and I don't see a overloaded constructor that takes a string...
Steven Parker
241,807 PointsSteven Parker
241,807 PointsWhere did you find that list of constructors? That's only 6 of the 11 that I found. Are you perhaps working with some obsolete assemblies?
I was looking on MSDN here.
Dale Bailey
20,269 PointsDale Bailey
20,269 PointsYeah I know confusing , I believe it's something to do with portable class library as I only have the constructors included in it? but as a noob mac user I don't really understand.
I got it from ctrl clicking the class
Steven Parker
241,807 PointsSteven Parker
241,807 PointsIt does sound like you need a newer version of the System.Io assembly.
Your current version of StreamReader won't work as used in the video. As a work-around you might try replacing "
file.FullName
" with "new FileStream(file.FullName, FileMode.Open)
".Dale Bailey
20,269 PointsDale Bailey
20,269 PointsIt seems strange as it was only downloaded recently so i downloaded it again and still have the same problem, If you look here under .net framework 4 version (I assume thats what i have?) https://msdn.microsoft.com/en-us/library/system.io.streamreader(v=vs.100).aspx you can see the missing constructors are the ones that are "not supported by the portable class library" I assume this is the root of the issue although I don't fully understand how when or why this is implemented?
Dale Bailey
20,269 PointsDale Bailey
20,269 PointsThis doesn't work in visual studio or visual studio code but it does work in xamarin studio... Whats going on why are the constructors available in xamarin studio?
Steven Parker
241,807 PointsSteven Parker
241,807 PointsDoes my work-around suggestion help?
I can only guess that Xamarin studio comes with a more current version of System.IO.
Dale Bailey
20,269 PointsDale Bailey
20,269 PointsIt does but I'm hoping for a solution rather than a work around, I could have thousands of missing constructors which will cause lots of problems...