Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial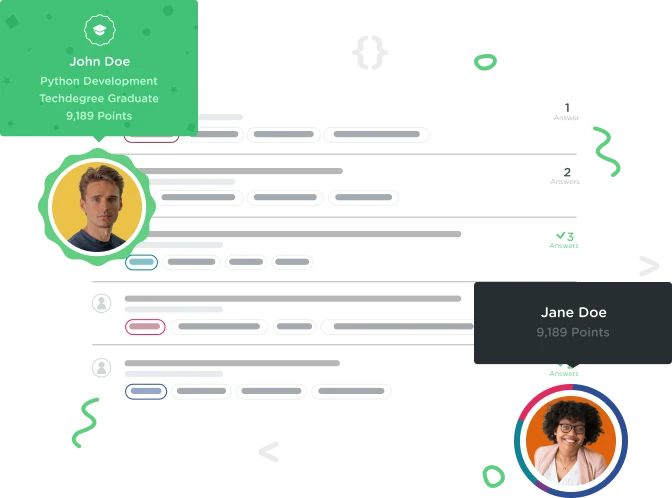

Ryan Voltaire
3,534 PointsConsole says "command not found"
Every time I type in the console commands clear && java Example.java && Example , The console replies "bash: Example: commands not found". I took the last command off and only ran the clear and compile command together and everything works fine, but when I try to open the Example.java file, I get the same error. This is what my code looks like:
Treet.java
package com.teamtreehouse;
import java.util.Date;
public class Treet
{
private String mAuthor;
private String mDescription;
private Date mCreationDate;
// Constructor
public Treet(String author, String description, Date creationDate)
{
mAuthor = author;
mDescription = description;
mCreationDate = creationDate;
}
// ACCESSOR METHODS
public String getAuthor()
{
return mAuthor;
}
public String getDescription()
{
return mDescription;
}
public Date getCreationDate()
{
return mCreationDate;
}
}
Example.java
import java.util.Date;
import com.teamtreehouse.Treet;
public class Example
{
public static void main(String[] args)
{
Treet treet = new Treet(
"Ryan",
"I just earned the Delivering the MVP badge on @treehouse!" +
"Last challenge was really fun! Idk why I suck when it comes to making small games",
new Date(1456309920000L));
System.out.printf("This is a new Treet: %s %n", treet);
}
}
I believe this error is telling me that it simply cannot find the file, but the file hasn't changed names, been moved, or deleted. I feel the answer is right there in front of me, but I'm just not seeing it. Any help is appreciated :D!
1 Answer

dman10345
7,109 PointsYou said you try to open the Example.java class, I'm going to assume you mean run based on your attempted command. Ensure you are running the Example.class file as this is the compiled bytecode made by the compiler.
Simple command line commands
javac Example.java //compiles file
java Example /* notice no extension as. the jvm automatically knows to run .class files and assuming your file compiles with no errors or there is already a compiled version of your java file this command will run the program*/
You are getting a error for command not found because the command line prompt is attempting to use a command called Example which does not exist.
Ryan Voltaire
3,534 PointsRyan Voltaire
3,534 PointsI knew it was something super simple that I was missing! Thank you, I really appreciate it
dman10345
7,109 Pointsdman10345
7,109 PointsAnytime bud, that's the joys of coding! It's usually the small things: typos, missed semicolon, extra curly brace, etc. You start to get an eye for it, it's all in the practice.
Goodluck and happy coding!