Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial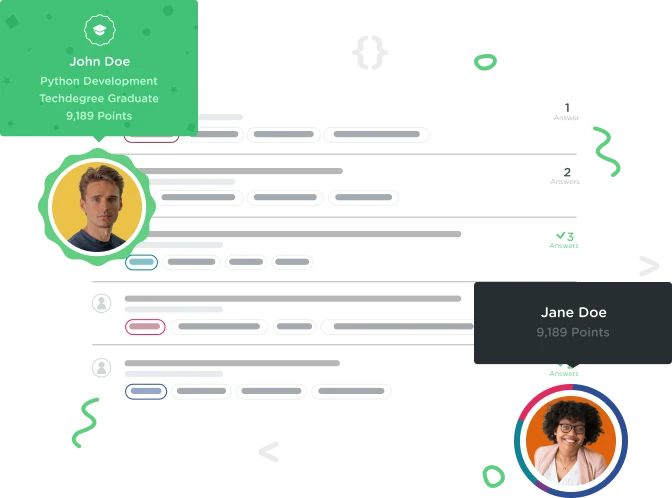

Oscar Lopez
971 Pointsconsole.printf(); versus, console.println();
What is the difference? Why do we use console.printf(); for this course instead of console.println();?
3 Answers

manav
5,466 PointsQ: Why do we use console.printf(); for this course instead of console.println();?
A: First of all, console.println() will not work:
Console console = System.console();
console.println("Hello World!"); // will throw compile-time error
Reason being, the Console class does not have direct access to println() method. To access the println() method you need to invoke another method writer() to retrieve the object of PrintWriter class which has access to the println() method. Hence the working code will look like this:
Console console = System.console();
console.writer().println("Hello World!"); // Hello World!
Another reason could be the flexibility of printf() method that allows output to be formatted like left-justified, right-justified, character width, decimal places etc.
Console console = System.console();
int n = 123;
console.printf("%d%n", n); // "123"
console.printf("%05d%n", n); // "00123"
console.printf("%+5d%n", n); // " +123"
double pi = Math.PI;
console.printf("%f%n", pi); // "3.141593"
console.printf("%.2f%n", pi); // "3.14"
console.printf("%8.2f%n", pi); // " 3.14"
console.printf("%-8.2f%n", pi); // "3.14 "
Hope this helps!

Aung Maw
643 PointsCan someone please explain what is %d%n, %f%n, %.2f%n are? Sorry I am new to this
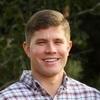
Zachary Betz
10,413 PointsHi Oscar, to add onto doctacloak's explanation, see the below examples.
Both examples print "Jim has 2 pets".
String name = "Jim";
int petCount = 2;
System.out.printf("%s has %d pets", name, petCount);
System.out.println(name + " has " + petCount + " pets");

Oscar Lopez
971 PointsThank you for clarifying.
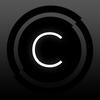
doctacloak
12,202 PointsOscar Lopez β You're welcome man, don't mention it!
doctacloak
12,202 Pointsdoctacloak
12,202 PointsHello Oscar!
First it is important to understand that the names are simply short versions of their function.
println
β This is short for "Print Line"Whenever we come across println(), what is happening is a new blank line is being triggered or a return and then your message is being printed; in this case, printed to the console.
One thing I would take into account is that println() does not give you the ability to write format specifiers within the parenthesis, be wary of that. In fact, it is actually quite similar to print() except β I am unsure if you've taken the console foundations course, you should if you haven't βΒ it puts the cursor on the starting of the next line after printing the data being passed to it.
printf
βΒ This is short for "Print Formatter"Print Formatter essentially grants you the ability to mark where in the strings variables will be passed to and it will pass in those variables with it. This will save you from having to do a long String concatenation, and could possibly improve your efficiency on the job as well.
Now in the context of your question, you are asking about both of these in regards to the console. The same thing will occur for both of them as I have described above, the only difference is it will be printed to the User/You/Me/Bob/Sandy in the console. Sometimes you will see System.out even but people rarely use those unless they want quick single outputs that do not require anything extravagant with it, such as debugging.