Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial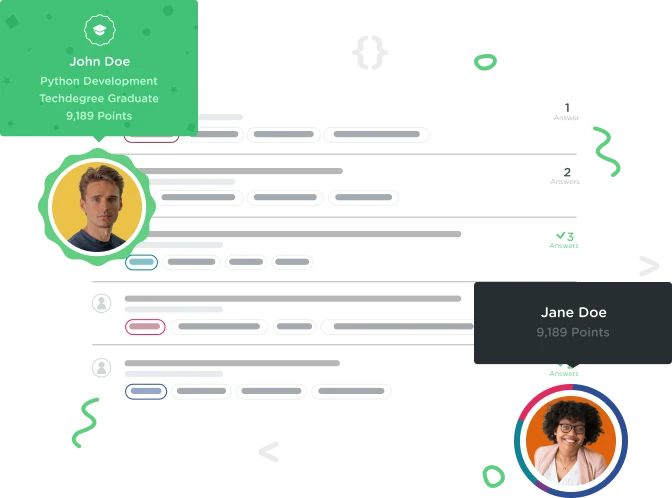

Danielle Gabriszeski
Python Development Techdegree Graduate 8,787 PointsConstant looping - When I use this code a second time through it continuelly loops, regardless of input
Hey guys! One time through the code works great, but if I input 'no' a second time I am just given 'no' looped forever. Can anyone help me figure out why this is happening? Thank you in advance!
name = input("What's your name? ")
understanding = input("Welcome {}! Do you feel like you fully understand 'while' loops?\n(Enter yes/no) ".format(name))
help = input("'while' loops repeat as long as certain Boolean, or True/False statment, is met.\nDo you feel like you understand 'while' loops now {}?\n(Enter yes/no) ".format(name))
while understanding.lower() != 'no':
print(help)
print("Great job {}! This stuff is hard isn't it?".format(name))
[MOD: added ```python formatting -cf]
3 Answers
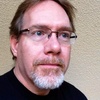
Chris Freeman
Treehouse Moderator 68,441 PointsThe variable help
points at the results of the input
statement. In the code:
while understanding.lower() != 'no':
print(help)
nothing modifies the value of understanding
. So if the condition is met, the code execution will enter the loop but have no way out.
One solution is adding a function inside the loop that returns a new value for understanding
Post back if you need more help. Good luck!!!

Danielle Gabriszeski
Python Development Techdegree Graduate 8,787 PointsHi Chris! Thank you so much for your response. I still don't seem to understand - I'm sorry! Can you define a funtion within a 'while' block? I went back and re watched the while video, but I'm struggling to figure out where certain things are used within it like I tried to use (code below) which also didn't work. I'm sorry to be a pain. I'll re watch videos, but am struggling to even figure out which ones to re watch at this point. Thank you again!
while True:
if understanding.lower == 'yes':
break
elif understanding.lower == 'no':
print(help)
continue
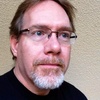
Chris Freeman
Treehouse Moderator 68,441 PointsOne rearrangement might be:
name = input("What's your name? ")
understanding = input("Welcome {}! Do you feel like you fully understand 'while' loops?\n(Enter yes/no) ".format(name))
def get_help():
return input("'while' loops repeat as long as certain Boolean, or True/False statment, is met.\nDo you feel like you understand 'while' loops now {}?\n(Enter yes/no) ".format(name))
need_help = 'no'
while understanding.lower() != 'yes' and need_help.lower() != 'yes':
need_help = get_help()
print("Great job {}! This stuff is hard isn't it?".format(name))
Results in
Whatโs your name? B
Welcome B! Do you feel like you fully understand โwhileโ loops?
(Enter yes/no) no
โwhileโ loops repeat as long as certain Boolean, or True/False statment, is met.
Do you feel like you understand โwhileโ loops now B?
(Enter yes/no) no
โwhileโ loops repeat as long as certain Boolean, or True/False statment, is met.
Do you feel like you understand โwhileโ loops now B?
(Enter yes/no) yes
Great job B! This stuff is hard isnโt it?
Also, be careful of the context of asking yes/no questions to be sure you are getting the โyesโ or โnoโ you expect. In the original code, help was printed if the answer was not โnoโ. I understand but still get help?

Danielle Gabriszeski
Python Development Techdegree Graduate 8,787 PointsOk, thank you Chris! I think I understand the general gist, but I will go back and watch the videos again for another refresh. Thank again for your help!